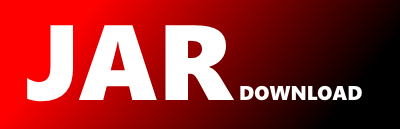
org.jclouds.azurecompute.arm.domain.AutoValue_VMImage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_VMImage extends VMImage {
private final String publisher;
private final String offer;
private final String sku;
private final String version;
private final String location;
private final String group;
private final String storage;
private final String vhd1;
private final String vhd2;
private final String name;
private final boolean custom;
private final String customImageId;
private final String resourceGroup;
private final Version.VersionProperties versionProperties;
private AutoValue_VMImage(
@Nullable String publisher,
@Nullable String offer,
@Nullable String sku,
@Nullable String version,
@Nullable String location,
@Nullable String group,
@Nullable String storage,
@Nullable String vhd1,
@Nullable String vhd2,
@Nullable String name,
boolean custom,
@Nullable String customImageId,
@Nullable String resourceGroup,
@Nullable Version.VersionProperties versionProperties) {
this.publisher = publisher;
this.offer = offer;
this.sku = sku;
this.version = version;
this.location = location;
this.group = group;
this.storage = storage;
this.vhd1 = vhd1;
this.vhd2 = vhd2;
this.name = name;
this.custom = custom;
this.customImageId = customImageId;
this.resourceGroup = resourceGroup;
this.versionProperties = versionProperties;
}
@Nullable
@Override
public String publisher() {
return publisher;
}
@Nullable
@Override
public String offer() {
return offer;
}
@Nullable
@Override
public String sku() {
return sku;
}
@Nullable
@Override
public String version() {
return version;
}
@Nullable
@Override
public String location() {
return location;
}
@Nullable
@Override
public String group() {
return group;
}
@Nullable
@Override
public String storage() {
return storage;
}
@Nullable
@Override
public String vhd1() {
return vhd1;
}
@Nullable
@Override
public String vhd2() {
return vhd2;
}
@Nullable
@Override
public String name() {
return name;
}
@Override
public boolean custom() {
return custom;
}
@Nullable
@Override
public String customImageId() {
return customImageId;
}
@Nullable
@Override
public String resourceGroup() {
return resourceGroup;
}
@Nullable
@Override
public Version.VersionProperties versionProperties() {
return versionProperties;
}
@Override
public String toString() {
return "VMImage{"
+ "publisher=" + publisher + ", "
+ "offer=" + offer + ", "
+ "sku=" + sku + ", "
+ "version=" + version + ", "
+ "location=" + location + ", "
+ "group=" + group + ", "
+ "storage=" + storage + ", "
+ "vhd1=" + vhd1 + ", "
+ "vhd2=" + vhd2 + ", "
+ "name=" + name + ", "
+ "custom=" + custom + ", "
+ "customImageId=" + customImageId + ", "
+ "resourceGroup=" + resourceGroup + ", "
+ "versionProperties=" + versionProperties
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof VMImage) {
VMImage that = (VMImage) o;
return ((this.publisher == null) ? (that.publisher() == null) : this.publisher.equals(that.publisher()))
&& ((this.offer == null) ? (that.offer() == null) : this.offer.equals(that.offer()))
&& ((this.sku == null) ? (that.sku() == null) : this.sku.equals(that.sku()))
&& ((this.version == null) ? (that.version() == null) : this.version.equals(that.version()))
&& ((this.location == null) ? (that.location() == null) : this.location.equals(that.location()))
&& ((this.group == null) ? (that.group() == null) : this.group.equals(that.group()))
&& ((this.storage == null) ? (that.storage() == null) : this.storage.equals(that.storage()))
&& ((this.vhd1 == null) ? (that.vhd1() == null) : this.vhd1.equals(that.vhd1()))
&& ((this.vhd2 == null) ? (that.vhd2() == null) : this.vhd2.equals(that.vhd2()))
&& ((this.name == null) ? (that.name() == null) : this.name.equals(that.name()))
&& (this.custom == that.custom())
&& ((this.customImageId == null) ? (that.customImageId() == null) : this.customImageId.equals(that.customImageId()))
&& ((this.resourceGroup == null) ? (that.resourceGroup() == null) : this.resourceGroup.equals(that.resourceGroup()))
&& ((this.versionProperties == null) ? (that.versionProperties() == null) : this.versionProperties.equals(that.versionProperties()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (publisher == null) ? 0 : this.publisher.hashCode();
h *= 1000003;
h ^= (offer == null) ? 0 : this.offer.hashCode();
h *= 1000003;
h ^= (sku == null) ? 0 : this.sku.hashCode();
h *= 1000003;
h ^= (version == null) ? 0 : this.version.hashCode();
h *= 1000003;
h ^= (location == null) ? 0 : this.location.hashCode();
h *= 1000003;
h ^= (group == null) ? 0 : this.group.hashCode();
h *= 1000003;
h ^= (storage == null) ? 0 : this.storage.hashCode();
h *= 1000003;
h ^= (vhd1 == null) ? 0 : this.vhd1.hashCode();
h *= 1000003;
h ^= (vhd2 == null) ? 0 : this.vhd2.hashCode();
h *= 1000003;
h ^= (name == null) ? 0 : this.name.hashCode();
h *= 1000003;
h ^= this.custom ? 1231 : 1237;
h *= 1000003;
h ^= (customImageId == null) ? 0 : this.customImageId.hashCode();
h *= 1000003;
h ^= (resourceGroup == null) ? 0 : this.resourceGroup.hashCode();
h *= 1000003;
h ^= (versionProperties == null) ? 0 : this.versionProperties.hashCode();
return h;
}
@Override
public VMImage.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends VMImage.Builder {
private String publisher;
private String offer;
private String sku;
private String version;
private String location;
private String group;
private String storage;
private String vhd1;
private String vhd2;
private String name;
private Boolean custom;
private String customImageId;
private String resourceGroup;
private Version.VersionProperties versionProperties;
Builder() {
}
private Builder(VMImage source) {
this.publisher = source.publisher();
this.offer = source.offer();
this.sku = source.sku();
this.version = source.version();
this.location = source.location();
this.group = source.group();
this.storage = source.storage();
this.vhd1 = source.vhd1();
this.vhd2 = source.vhd2();
this.name = source.name();
this.custom = source.custom();
this.customImageId = source.customImageId();
this.resourceGroup = source.resourceGroup();
this.versionProperties = source.versionProperties();
}
@Override
public VMImage.Builder publisher(@Nullable String publisher) {
this.publisher = publisher;
return this;
}
@Override
public VMImage.Builder offer(@Nullable String offer) {
this.offer = offer;
return this;
}
@Override
public VMImage.Builder sku(@Nullable String sku) {
this.sku = sku;
return this;
}
@Override
public VMImage.Builder version(@Nullable String version) {
this.version = version;
return this;
}
@Override
public VMImage.Builder location(@Nullable String location) {
this.location = location;
return this;
}
@Override
public VMImage.Builder group(@Nullable String group) {
this.group = group;
return this;
}
@Override
public VMImage.Builder storage(@Nullable String storage) {
this.storage = storage;
return this;
}
@Override
public VMImage.Builder vhd1(@Nullable String vhd1) {
this.vhd1 = vhd1;
return this;
}
@Override
public VMImage.Builder vhd2(@Nullable String vhd2) {
this.vhd2 = vhd2;
return this;
}
@Override
public VMImage.Builder name(@Nullable String name) {
this.name = name;
return this;
}
@Override
public VMImage.Builder custom(boolean custom) {
this.custom = custom;
return this;
}
@Override
public VMImage.Builder customImageId(@Nullable String customImageId) {
this.customImageId = customImageId;
return this;
}
@Override
public VMImage.Builder resourceGroup(@Nullable String resourceGroup) {
this.resourceGroup = resourceGroup;
return this;
}
@Override
public VMImage.Builder versionProperties(@Nullable Version.VersionProperties versionProperties) {
this.versionProperties = versionProperties;
return this;
}
@Override
public VMImage build() {
String missing = "";
if (this.custom == null) {
missing += " custom";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_VMImage(
this.publisher,
this.offer,
this.sku,
this.version,
this.location,
this.group,
this.storage,
this.vhd1,
this.vhd2,
this.name,
this.custom,
this.customImageId,
this.resourceGroup,
this.versionProperties);
}
}
}