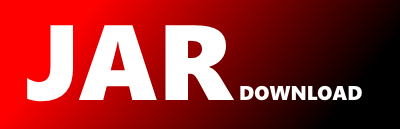
org.jclouds.azurecompute.arm.domain.AutoValue_VirtualMachineProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_VirtualMachineProperties extends VirtualMachineProperties {
private final String vmId;
private final String licenseType;
private final IdReference availabilitySet;
private final HardwareProfile hardwareProfile;
private final StorageProfile storageProfile;
private final OSProfile osProfile;
private final NetworkProfile networkProfile;
private final DiagnosticsProfile diagnosticsProfile;
private final VirtualMachineProperties.ProvisioningState provisioningState;
private AutoValue_VirtualMachineProperties(
@Nullable String vmId,
@Nullable String licenseType,
@Nullable IdReference availabilitySet,
@Nullable HardwareProfile hardwareProfile,
@Nullable StorageProfile storageProfile,
@Nullable OSProfile osProfile,
@Nullable NetworkProfile networkProfile,
@Nullable DiagnosticsProfile diagnosticsProfile,
@Nullable VirtualMachineProperties.ProvisioningState provisioningState) {
this.vmId = vmId;
this.licenseType = licenseType;
this.availabilitySet = availabilitySet;
this.hardwareProfile = hardwareProfile;
this.storageProfile = storageProfile;
this.osProfile = osProfile;
this.networkProfile = networkProfile;
this.diagnosticsProfile = diagnosticsProfile;
this.provisioningState = provisioningState;
}
@Nullable
@Override
public String vmId() {
return vmId;
}
@Nullable
@Override
public String licenseType() {
return licenseType;
}
@Nullable
@Override
public IdReference availabilitySet() {
return availabilitySet;
}
@Nullable
@Override
public HardwareProfile hardwareProfile() {
return hardwareProfile;
}
@Nullable
@Override
public StorageProfile storageProfile() {
return storageProfile;
}
@Nullable
@Override
public OSProfile osProfile() {
return osProfile;
}
@Nullable
@Override
public NetworkProfile networkProfile() {
return networkProfile;
}
@Nullable
@Override
public DiagnosticsProfile diagnosticsProfile() {
return diagnosticsProfile;
}
@Nullable
@Override
public VirtualMachineProperties.ProvisioningState provisioningState() {
return provisioningState;
}
@Override
public String toString() {
return "VirtualMachineProperties{"
+ "vmId=" + vmId + ", "
+ "licenseType=" + licenseType + ", "
+ "availabilitySet=" + availabilitySet + ", "
+ "hardwareProfile=" + hardwareProfile + ", "
+ "storageProfile=" + storageProfile + ", "
+ "osProfile=" + osProfile + ", "
+ "networkProfile=" + networkProfile + ", "
+ "diagnosticsProfile=" + diagnosticsProfile + ", "
+ "provisioningState=" + provisioningState
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof VirtualMachineProperties) {
VirtualMachineProperties that = (VirtualMachineProperties) o;
return ((this.vmId == null) ? (that.vmId() == null) : this.vmId.equals(that.vmId()))
&& ((this.licenseType == null) ? (that.licenseType() == null) : this.licenseType.equals(that.licenseType()))
&& ((this.availabilitySet == null) ? (that.availabilitySet() == null) : this.availabilitySet.equals(that.availabilitySet()))
&& ((this.hardwareProfile == null) ? (that.hardwareProfile() == null) : this.hardwareProfile.equals(that.hardwareProfile()))
&& ((this.storageProfile == null) ? (that.storageProfile() == null) : this.storageProfile.equals(that.storageProfile()))
&& ((this.osProfile == null) ? (that.osProfile() == null) : this.osProfile.equals(that.osProfile()))
&& ((this.networkProfile == null) ? (that.networkProfile() == null) : this.networkProfile.equals(that.networkProfile()))
&& ((this.diagnosticsProfile == null) ? (that.diagnosticsProfile() == null) : this.diagnosticsProfile.equals(that.diagnosticsProfile()))
&& ((this.provisioningState == null) ? (that.provisioningState() == null) : this.provisioningState.equals(that.provisioningState()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (vmId == null) ? 0 : this.vmId.hashCode();
h *= 1000003;
h ^= (licenseType == null) ? 0 : this.licenseType.hashCode();
h *= 1000003;
h ^= (availabilitySet == null) ? 0 : this.availabilitySet.hashCode();
h *= 1000003;
h ^= (hardwareProfile == null) ? 0 : this.hardwareProfile.hashCode();
h *= 1000003;
h ^= (storageProfile == null) ? 0 : this.storageProfile.hashCode();
h *= 1000003;
h ^= (osProfile == null) ? 0 : this.osProfile.hashCode();
h *= 1000003;
h ^= (networkProfile == null) ? 0 : this.networkProfile.hashCode();
h *= 1000003;
h ^= (diagnosticsProfile == null) ? 0 : this.diagnosticsProfile.hashCode();
h *= 1000003;
h ^= (provisioningState == null) ? 0 : this.provisioningState.hashCode();
return h;
}
@Override
public VirtualMachineProperties.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends VirtualMachineProperties.Builder {
private String vmId;
private String licenseType;
private IdReference availabilitySet;
private HardwareProfile hardwareProfile;
private StorageProfile storageProfile;
private OSProfile osProfile;
private NetworkProfile networkProfile;
private DiagnosticsProfile diagnosticsProfile;
private VirtualMachineProperties.ProvisioningState provisioningState;
Builder() {
}
private Builder(VirtualMachineProperties source) {
this.vmId = source.vmId();
this.licenseType = source.licenseType();
this.availabilitySet = source.availabilitySet();
this.hardwareProfile = source.hardwareProfile();
this.storageProfile = source.storageProfile();
this.osProfile = source.osProfile();
this.networkProfile = source.networkProfile();
this.diagnosticsProfile = source.diagnosticsProfile();
this.provisioningState = source.provisioningState();
}
@Override
public VirtualMachineProperties.Builder vmId(@Nullable String vmId) {
this.vmId = vmId;
return this;
}
@Override
public VirtualMachineProperties.Builder licenseType(@Nullable String licenseType) {
this.licenseType = licenseType;
return this;
}
@Override
public VirtualMachineProperties.Builder availabilitySet(@Nullable IdReference availabilitySet) {
this.availabilitySet = availabilitySet;
return this;
}
@Override
public VirtualMachineProperties.Builder hardwareProfile(@Nullable HardwareProfile hardwareProfile) {
this.hardwareProfile = hardwareProfile;
return this;
}
@Override
public VirtualMachineProperties.Builder storageProfile(@Nullable StorageProfile storageProfile) {
this.storageProfile = storageProfile;
return this;
}
@Override
public VirtualMachineProperties.Builder osProfile(@Nullable OSProfile osProfile) {
this.osProfile = osProfile;
return this;
}
@Override
public VirtualMachineProperties.Builder networkProfile(@Nullable NetworkProfile networkProfile) {
this.networkProfile = networkProfile;
return this;
}
@Override
public VirtualMachineProperties.Builder diagnosticsProfile(@Nullable DiagnosticsProfile diagnosticsProfile) {
this.diagnosticsProfile = diagnosticsProfile;
return this;
}
@Override
public VirtualMachineProperties.Builder provisioningState(@Nullable VirtualMachineProperties.ProvisioningState provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@Override
public VirtualMachineProperties build() {
return new AutoValue_VirtualMachineProperties(
this.vmId,
this.licenseType,
this.availabilitySet,
this.hardwareProfile,
this.storageProfile,
this.osProfile,
this.networkProfile,
this.diagnosticsProfile,
this.provisioningState);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy