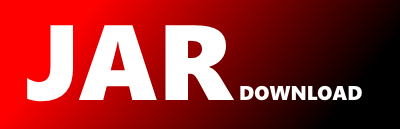
org.jclouds.azurecompute.arm.domain.AutoValue_VirtualMachineScaleSetVirtualMachineProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_VirtualMachineScaleSetVirtualMachineProfile extends VirtualMachineScaleSetVirtualMachineProfile {
private final StorageProfile storageProfile;
private final VirtualMachineScaleSetOSProfile osProfile;
private final VirtualMachineScaleSetNetworkProfile networkProfile;
private final ExtensionProfile extensionProfile;
private AutoValue_VirtualMachineScaleSetVirtualMachineProfile(
StorageProfile storageProfile,
VirtualMachineScaleSetOSProfile osProfile,
VirtualMachineScaleSetNetworkProfile networkProfile,
ExtensionProfile extensionProfile) {
this.storageProfile = storageProfile;
this.osProfile = osProfile;
this.networkProfile = networkProfile;
this.extensionProfile = extensionProfile;
}
@Override
public StorageProfile storageProfile() {
return storageProfile;
}
@Override
public VirtualMachineScaleSetOSProfile osProfile() {
return osProfile;
}
@Override
public VirtualMachineScaleSetNetworkProfile networkProfile() {
return networkProfile;
}
@Override
public ExtensionProfile extensionProfile() {
return extensionProfile;
}
@Override
public String toString() {
return "VirtualMachineScaleSetVirtualMachineProfile{"
+ "storageProfile=" + storageProfile + ", "
+ "osProfile=" + osProfile + ", "
+ "networkProfile=" + networkProfile + ", "
+ "extensionProfile=" + extensionProfile
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof VirtualMachineScaleSetVirtualMachineProfile) {
VirtualMachineScaleSetVirtualMachineProfile that = (VirtualMachineScaleSetVirtualMachineProfile) o;
return (this.storageProfile.equals(that.storageProfile()))
&& (this.osProfile.equals(that.osProfile()))
&& (this.networkProfile.equals(that.networkProfile()))
&& (this.extensionProfile.equals(that.extensionProfile()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.storageProfile.hashCode();
h *= 1000003;
h ^= this.osProfile.hashCode();
h *= 1000003;
h ^= this.networkProfile.hashCode();
h *= 1000003;
h ^= this.extensionProfile.hashCode();
return h;
}
@Override
public VirtualMachineScaleSetVirtualMachineProfile.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends VirtualMachineScaleSetVirtualMachineProfile.Builder {
private StorageProfile storageProfile;
private VirtualMachineScaleSetOSProfile osProfile;
private VirtualMachineScaleSetNetworkProfile networkProfile;
private ExtensionProfile extensionProfile;
Builder() {
}
private Builder(VirtualMachineScaleSetVirtualMachineProfile source) {
this.storageProfile = source.storageProfile();
this.osProfile = source.osProfile();
this.networkProfile = source.networkProfile();
this.extensionProfile = source.extensionProfile();
}
@Override
public VirtualMachineScaleSetVirtualMachineProfile.Builder storageProfile(StorageProfile storageProfile) {
if (storageProfile == null) {
throw new NullPointerException("Null storageProfile");
}
this.storageProfile = storageProfile;
return this;
}
@Override
public VirtualMachineScaleSetVirtualMachineProfile.Builder osProfile(VirtualMachineScaleSetOSProfile osProfile) {
if (osProfile == null) {
throw new NullPointerException("Null osProfile");
}
this.osProfile = osProfile;
return this;
}
@Override
public VirtualMachineScaleSetVirtualMachineProfile.Builder networkProfile(VirtualMachineScaleSetNetworkProfile networkProfile) {
if (networkProfile == null) {
throw new NullPointerException("Null networkProfile");
}
this.networkProfile = networkProfile;
return this;
}
@Override
public VirtualMachineScaleSetVirtualMachineProfile.Builder extensionProfile(ExtensionProfile extensionProfile) {
if (extensionProfile == null) {
throw new NullPointerException("Null extensionProfile");
}
this.extensionProfile = extensionProfile;
return this;
}
@Override
public VirtualMachineScaleSetVirtualMachineProfile build() {
String missing = "";
if (this.storageProfile == null) {
missing += " storageProfile";
}
if (this.osProfile == null) {
missing += " osProfile";
}
if (this.networkProfile == null) {
missing += " networkProfile";
}
if (this.extensionProfile == null) {
missing += " extensionProfile";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_VirtualMachineScaleSetVirtualMachineProfile(
this.storageProfile,
this.osProfile,
this.networkProfile,
this.extensionProfile);
}
}
}