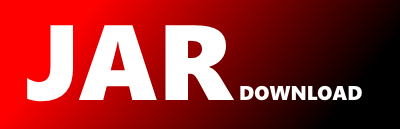
org.jclouds.azurecompute.arm.domain.Deployment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jclouds.azurecompute.arm.domain;
import java.util.List;
import java.util.Map;
import org.jclouds.azurecompute.arm.util.GetEnumValue;
import org.jclouds.domain.JsonBall;
import org.jclouds.javax.annotation.Nullable;
import org.jclouds.json.SerializedNames;
import com.google.auto.value.AutoValue;
import shaded.com.google.common.collect.ImmutableMap;
import static shaded.com.google.common.collect.ImmutableList.copyOf;
@AutoValue
public abstract class Deployment {
public enum ProvisioningState {
ACCEPTED,
READY,
CANCELED,
FAILED,
DELETED,
SUCCEEDED,
RUNNING,
UNRECOGNIZED;
public static ProvisioningState fromValue(final String text) {
return (ProvisioningState) GetEnumValue.fromValueOrDefault(text, ProvisioningState.UNRECOGNIZED);
}
}
public enum DeploymentMode {
INCREMENTAL,
COMPLETE,
UNRECOGNIZED;
public static DeploymentMode fromValue(final String text) {
return (DeploymentMode) GetEnumValue.fromValueOrDefault(text, DeploymentMode.UNRECOGNIZED);
}
}
@AutoValue
public abstract static class TypeValue {
public abstract String type();
public abstract String value();
@SerializedNames({"type", "value"})
public static TypeValue create(final String type, final String value) {
return new AutoValue_Deployment_TypeValue(type, value);
}
}
@AutoValue
public abstract static class ProviderResourceType {
@Nullable
public abstract String resourceType();
@Nullable
public abstract List locations();
@Nullable
public abstract List apiVersions();
@Nullable
public abstract Map properties();
@SerializedNames({"resourceType", "locations", "apiVersions", "properties"})
public static ProviderResourceType create(final String resourceType,
final List locations,
final List apiVersions,
@Nullable final Map properties) {
return new AutoValue_Deployment_ProviderResourceType(resourceType,
locations == null ? null : copyOf(locations),
apiVersions == null ? null : copyOf(apiVersions),
properties == null ? ImmutableMap.builder().build() : ImmutableMap.copyOf(properties));
}
}
@AutoValue
public abstract static class Provider {
@Nullable
public abstract String id();
@Nullable
public abstract String namespace();
@Nullable
public abstract String registrationState();
@Nullable
public abstract List resourceTypes();
@SerializedNames({"id", "namespace", "registrationState", "resourceTypes"})
public static Provider create(final String id,
final String namespace,
final String registrationState,
final List resourceTypes) {
return new AutoValue_Deployment_Provider(id, namespace, registrationState, resourceTypes == null ? null : copyOf(resourceTypes));
}
}
@AutoValue
public abstract static class Dependency {
@Nullable
public abstract List dependencies();
@Nullable
public abstract List dependsOn();
@Nullable
public abstract String id();
@Nullable
public abstract String resourceType();
@Nullable
public abstract String resourceName();
@SerializedNames({"dependencies", "dependsOn", "id", "resourceType", "resourceName"})
public static Dependency create(final List dependencies,
final List dependsOn,
final String id,
final String resourceType,
final String resourceName) {
return new AutoValue_Deployment_Dependency(dependencies == null ? null : copyOf(dependencies),
dependsOn == null ? null : copyOf(dependsOn), id, resourceType, resourceName);
}
}
@AutoValue
public abstract static class ContentLink {
public abstract String uri();
@Nullable
public abstract String contentVersion();
@SerializedNames({"uri", "contentVersion"})
public static ContentLink create(final String uri, final String contentVersion) {
return new AutoValue_Deployment_ContentLink(uri, contentVersion);
}
}
@AutoValue
public abstract static class DeploymentProperties implements Provisionable {
@Nullable
public abstract String provisioningState();
@Nullable
public abstract String correlationId();
@Nullable
public abstract String timestamp();
@Nullable
public abstract Map outputs();
@Nullable
public abstract List providers();
@Nullable
public abstract List dependencies();
@Nullable
public abstract Map template();
@Nullable
public abstract ContentLink templateLink();
@Nullable
public abstract Map parameters();
@Nullable
public abstract ContentLink parametersLink();
public abstract String mode();
// The entries below seem to be dynamic/not documented in the specification
@Nullable
public abstract String duration();
@Nullable
public abstract List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy