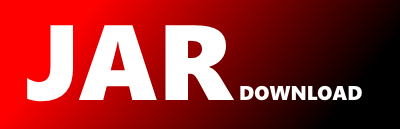
org.jclouds.azurecompute.arm.domain.loadbalancer.AutoValue_LoadBalancer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.azurecompute.arm.domain.loadbalancer;
import java.util.Map;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_LoadBalancer extends LoadBalancer {
private final String id;
private final String name;
private final String location;
private final String etag;
private final Map tags;
private final LoadBalancerProperties properties;
private final LoadBalancer.SKU sku;
private AutoValue_LoadBalancer(
@Nullable String id,
@Nullable String name,
@Nullable String location,
@Nullable String etag,
@Nullable Map tags,
@Nullable LoadBalancerProperties properties,
@Nullable LoadBalancer.SKU sku) {
this.id = id;
this.name = name;
this.location = location;
this.etag = etag;
this.tags = tags;
this.properties = properties;
this.sku = sku;
}
@Nullable
@Override
public String id() {
return id;
}
@Nullable
@Override
public String name() {
return name;
}
@Nullable
@Override
public String location() {
return location;
}
@Nullable
@Override
public String etag() {
return etag;
}
@Nullable
@Override
public Map tags() {
return tags;
}
@Nullable
@Override
public LoadBalancerProperties properties() {
return properties;
}
@Nullable
@Override
public LoadBalancer.SKU sku() {
return sku;
}
@Override
public String toString() {
return "LoadBalancer{"
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "location=" + location + ", "
+ "etag=" + etag + ", "
+ "tags=" + tags + ", "
+ "properties=" + properties + ", "
+ "sku=" + sku
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof LoadBalancer) {
LoadBalancer that = (LoadBalancer) o;
return ((this.id == null) ? (that.id() == null) : this.id.equals(that.id()))
&& ((this.name == null) ? (that.name() == null) : this.name.equals(that.name()))
&& ((this.location == null) ? (that.location() == null) : this.location.equals(that.location()))
&& ((this.etag == null) ? (that.etag() == null) : this.etag.equals(that.etag()))
&& ((this.tags == null) ? (that.tags() == null) : this.tags.equals(that.tags()))
&& ((this.properties == null) ? (that.properties() == null) : this.properties.equals(that.properties()))
&& ((this.sku == null) ? (that.sku() == null) : this.sku.equals(that.sku()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (id == null) ? 0 : this.id.hashCode();
h *= 1000003;
h ^= (name == null) ? 0 : this.name.hashCode();
h *= 1000003;
h ^= (location == null) ? 0 : this.location.hashCode();
h *= 1000003;
h ^= (etag == null) ? 0 : this.etag.hashCode();
h *= 1000003;
h ^= (tags == null) ? 0 : this.tags.hashCode();
h *= 1000003;
h ^= (properties == null) ? 0 : this.properties.hashCode();
h *= 1000003;
h ^= (sku == null) ? 0 : this.sku.hashCode();
return h;
}
@Override
public LoadBalancer.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends LoadBalancer.Builder {
private String id;
private String name;
private String location;
private String etag;
private Map tags;
private LoadBalancerProperties properties;
private LoadBalancer.SKU sku;
Builder() {
}
private Builder(LoadBalancer source) {
this.id = source.id();
this.name = source.name();
this.location = source.location();
this.etag = source.etag();
this.tags = source.tags();
this.properties = source.properties();
this.sku = source.sku();
}
@Override
public LoadBalancer.Builder id(@Nullable String id) {
this.id = id;
return this;
}
@Override
public LoadBalancer.Builder name(@Nullable String name) {
this.name = name;
return this;
}
@Override
public LoadBalancer.Builder location(@Nullable String location) {
this.location = location;
return this;
}
@Override
public LoadBalancer.Builder etag(@Nullable String etag) {
this.etag = etag;
return this;
}
@Override
public LoadBalancer.Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@Override
@Nullable Map tags() {
return tags;
}
@Override
public LoadBalancer.Builder properties(@Nullable LoadBalancerProperties properties) {
this.properties = properties;
return this;
}
@Override
public LoadBalancer.Builder sku(@Nullable LoadBalancer.SKU sku) {
this.sku = sku;
return this;
}
@Override
LoadBalancer autoBuild() {
return new AutoValue_LoadBalancer(
this.id,
this.name,
this.location,
this.etag,
this.tags,
this.properties,
this.sku);
}
}
}