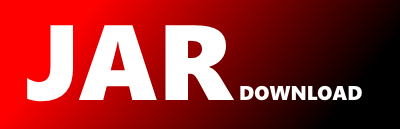
org.jclouds.b2.domain.AutoValue_B2Object Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.b2.domain;
import java.util.Date;
import java.util.Map;
import javax.annotation.Generated;
import org.jclouds.io.Payload;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_B2Object extends B2Object {
private final String fileId;
private final String fileName;
private final String contentSha1;
private final Map fileInfo;
private final Payload payload;
private final Date uploadTimestamp;
private final Action action;
private final String accountId;
private final String bucketId;
private final Long contentLength;
private final String contentType;
private final String contentRange;
AutoValue_B2Object(
String fileId,
String fileName,
@Nullable String contentSha1,
@Nullable Map fileInfo,
@Nullable Payload payload,
@Nullable Date uploadTimestamp,
@Nullable Action action,
@Nullable String accountId,
@Nullable String bucketId,
@Nullable Long contentLength,
@Nullable String contentType,
@Nullable String contentRange) {
if (fileId == null) {
throw new NullPointerException("Null fileId");
}
this.fileId = fileId;
if (fileName == null) {
throw new NullPointerException("Null fileName");
}
this.fileName = fileName;
this.contentSha1 = contentSha1;
this.fileInfo = fileInfo;
this.payload = payload;
this.uploadTimestamp = uploadTimestamp;
this.action = action;
this.accountId = accountId;
this.bucketId = bucketId;
this.contentLength = contentLength;
this.contentType = contentType;
this.contentRange = contentRange;
}
@Override
public String fileId() {
return fileId;
}
@Override
public String fileName() {
return fileName;
}
@Nullable
@Override
public String contentSha1() {
return contentSha1;
}
@Nullable
@Override
public Map fileInfo() {
return fileInfo;
}
@Nullable
@Override
public Payload payload() {
return payload;
}
@Nullable
@Override
public Date uploadTimestamp() {
return uploadTimestamp;
}
@Nullable
@Override
public Action action() {
return action;
}
@Nullable
@Override
public String accountId() {
return accountId;
}
@Nullable
@Override
public String bucketId() {
return bucketId;
}
@Nullable
@Override
public Long contentLength() {
return contentLength;
}
@Nullable
@Override
public String contentType() {
return contentType;
}
@Nullable
@Override
public String contentRange() {
return contentRange;
}
@Override
public String toString() {
return "B2Object{"
+ "fileId=" + fileId + ", "
+ "fileName=" + fileName + ", "
+ "contentSha1=" + contentSha1 + ", "
+ "fileInfo=" + fileInfo + ", "
+ "payload=" + payload + ", "
+ "uploadTimestamp=" + uploadTimestamp + ", "
+ "action=" + action + ", "
+ "accountId=" + accountId + ", "
+ "bucketId=" + bucketId + ", "
+ "contentLength=" + contentLength + ", "
+ "contentType=" + contentType + ", "
+ "contentRange=" + contentRange
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof B2Object) {
B2Object that = (B2Object) o;
return (this.fileId.equals(that.fileId()))
&& (this.fileName.equals(that.fileName()))
&& ((this.contentSha1 == null) ? (that.contentSha1() == null) : this.contentSha1.equals(that.contentSha1()))
&& ((this.fileInfo == null) ? (that.fileInfo() == null) : this.fileInfo.equals(that.fileInfo()))
&& ((this.payload == null) ? (that.payload() == null) : this.payload.equals(that.payload()))
&& ((this.uploadTimestamp == null) ? (that.uploadTimestamp() == null) : this.uploadTimestamp.equals(that.uploadTimestamp()))
&& ((this.action == null) ? (that.action() == null) : this.action.equals(that.action()))
&& ((this.accountId == null) ? (that.accountId() == null) : this.accountId.equals(that.accountId()))
&& ((this.bucketId == null) ? (that.bucketId() == null) : this.bucketId.equals(that.bucketId()))
&& ((this.contentLength == null) ? (that.contentLength() == null) : this.contentLength.equals(that.contentLength()))
&& ((this.contentType == null) ? (that.contentType() == null) : this.contentType.equals(that.contentType()))
&& ((this.contentRange == null) ? (that.contentRange() == null) : this.contentRange.equals(that.contentRange()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.fileId.hashCode();
h *= 1000003;
h ^= this.fileName.hashCode();
h *= 1000003;
h ^= (contentSha1 == null) ? 0 : this.contentSha1.hashCode();
h *= 1000003;
h ^= (fileInfo == null) ? 0 : this.fileInfo.hashCode();
h *= 1000003;
h ^= (payload == null) ? 0 : this.payload.hashCode();
h *= 1000003;
h ^= (uploadTimestamp == null) ? 0 : this.uploadTimestamp.hashCode();
h *= 1000003;
h ^= (action == null) ? 0 : this.action.hashCode();
h *= 1000003;
h ^= (accountId == null) ? 0 : this.accountId.hashCode();
h *= 1000003;
h ^= (bucketId == null) ? 0 : this.bucketId.hashCode();
h *= 1000003;
h ^= (contentLength == null) ? 0 : this.contentLength.hashCode();
h *= 1000003;
h ^= (contentType == null) ? 0 : this.contentType.hashCode();
h *= 1000003;
h ^= (contentRange == null) ? 0 : this.contentRange.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy