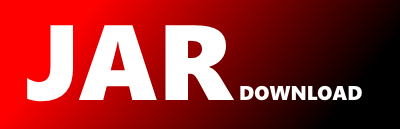
org.jclouds.digitalocean2.domain.AutoValue_Action Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.digitalocean2.domain;
import java.util.Date;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Action extends Action {
private final int id;
private final Action.Status status;
private final String type;
private final Date startedAt;
private final Date completedAt;
private final long resourceId;
private final String resourceType;
private final Region region;
private final String regionSlug;
AutoValue_Action(
int id,
Action.Status status,
String type,
Date startedAt,
@Nullable Date completedAt,
long resourceId,
String resourceType,
@Nullable Region region,
@Nullable String regionSlug) {
this.id = id;
if (status == null) {
throw new NullPointerException("Null status");
}
this.status = status;
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
if (startedAt == null) {
throw new NullPointerException("Null startedAt");
}
this.startedAt = startedAt;
this.completedAt = completedAt;
this.resourceId = resourceId;
if (resourceType == null) {
throw new NullPointerException("Null resourceType");
}
this.resourceType = resourceType;
this.region = region;
this.regionSlug = regionSlug;
}
@Override
public int id() {
return id;
}
@Override
public Action.Status status() {
return status;
}
@Override
public String type() {
return type;
}
@Override
public Date startedAt() {
return startedAt;
}
@Nullable
@Override
public Date completedAt() {
return completedAt;
}
@Override
public long resourceId() {
return resourceId;
}
@Override
public String resourceType() {
return resourceType;
}
@Nullable
@Override
public Region region() {
return region;
}
@Nullable
@Override
public String regionSlug() {
return regionSlug;
}
@Override
public String toString() {
return "Action{"
+ "id=" + id + ", "
+ "status=" + status + ", "
+ "type=" + type + ", "
+ "startedAt=" + startedAt + ", "
+ "completedAt=" + completedAt + ", "
+ "resourceId=" + resourceId + ", "
+ "resourceType=" + resourceType + ", "
+ "region=" + region + ", "
+ "regionSlug=" + regionSlug
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Action) {
Action that = (Action) o;
return (this.id == that.id())
&& (this.status.equals(that.status()))
&& (this.type.equals(that.type()))
&& (this.startedAt.equals(that.startedAt()))
&& ((this.completedAt == null) ? (that.completedAt() == null) : this.completedAt.equals(that.completedAt()))
&& (this.resourceId == that.resourceId())
&& (this.resourceType.equals(that.resourceType()))
&& ((this.region == null) ? (that.region() == null) : this.region.equals(that.region()))
&& ((this.regionSlug == null) ? (that.regionSlug() == null) : this.regionSlug.equals(that.regionSlug()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id;
h *= 1000003;
h ^= this.status.hashCode();
h *= 1000003;
h ^= this.type.hashCode();
h *= 1000003;
h ^= this.startedAt.hashCode();
h *= 1000003;
h ^= (completedAt == null) ? 0 : this.completedAt.hashCode();
h *= 1000003;
h ^= (this.resourceId >>> 32) ^ this.resourceId;
h *= 1000003;
h ^= this.resourceType.hashCode();
h *= 1000003;
h ^= (region == null) ? 0 : this.region.hashCode();
h *= 1000003;
h ^= (regionSlug == null) ? 0 : this.regionSlug.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy