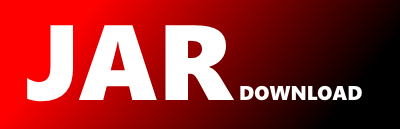
org.jclouds.digitalocean2.domain.options.AutoValue_CreateDropletOptions_DropletRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.digitalocean2.domain.options;
import java.util.Set;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_CreateDropletOptions_DropletRequest extends CreateDropletOptions.DropletRequest {
private final String name;
private final String region;
private final String size;
private final String image;
private final Set sshKeys;
private final Boolean backups;
private final Boolean ipv6;
private final Boolean privateNetworking;
private final String userData;
AutoValue_CreateDropletOptions_DropletRequest(
String name,
String region,
String size,
String image,
Set sshKeys,
Boolean backups,
Boolean ipv6,
Boolean privateNetworking,
@Nullable String userData) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
if (region == null) {
throw new NullPointerException("Null region");
}
this.region = region;
if (size == null) {
throw new NullPointerException("Null size");
}
this.size = size;
if (image == null) {
throw new NullPointerException("Null image");
}
this.image = image;
if (sshKeys == null) {
throw new NullPointerException("Null sshKeys");
}
this.sshKeys = sshKeys;
if (backups == null) {
throw new NullPointerException("Null backups");
}
this.backups = backups;
if (ipv6 == null) {
throw new NullPointerException("Null ipv6");
}
this.ipv6 = ipv6;
if (privateNetworking == null) {
throw new NullPointerException("Null privateNetworking");
}
this.privateNetworking = privateNetworking;
this.userData = userData;
}
@Override
String name() {
return name;
}
@Override
String region() {
return region;
}
@Override
String size() {
return size;
}
@Override
String image() {
return image;
}
@Override
Set sshKeys() {
return sshKeys;
}
@Override
Boolean backups() {
return backups;
}
@Override
Boolean ipv6() {
return ipv6;
}
@Override
Boolean privateNetworking() {
return privateNetworking;
}
@Nullable
@Override
String userData() {
return userData;
}
@Override
public String toString() {
return "DropletRequest{"
+ "name=" + name + ", "
+ "region=" + region + ", "
+ "size=" + size + ", "
+ "image=" + image + ", "
+ "sshKeys=" + sshKeys + ", "
+ "backups=" + backups + ", "
+ "ipv6=" + ipv6 + ", "
+ "privateNetworking=" + privateNetworking + ", "
+ "userData=" + userData
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CreateDropletOptions.DropletRequest) {
CreateDropletOptions.DropletRequest that = (CreateDropletOptions.DropletRequest) o;
return (this.name.equals(that.name()))
&& (this.region.equals(that.region()))
&& (this.size.equals(that.size()))
&& (this.image.equals(that.image()))
&& (this.sshKeys.equals(that.sshKeys()))
&& (this.backups.equals(that.backups()))
&& (this.ipv6.equals(that.ipv6()))
&& (this.privateNetworking.equals(that.privateNetworking()))
&& ((this.userData == null) ? (that.userData() == null) : this.userData.equals(that.userData()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= this.region.hashCode();
h *= 1000003;
h ^= this.size.hashCode();
h *= 1000003;
h ^= this.image.hashCode();
h *= 1000003;
h ^= this.sshKeys.hashCode();
h *= 1000003;
h ^= this.backups.hashCode();
h *= 1000003;
h ^= this.ipv6.hashCode();
h *= 1000003;
h ^= this.privateNetworking.hashCode();
h *= 1000003;
h ^= (userData == null) ? 0 : this.userData.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy