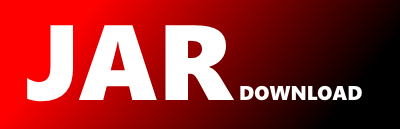
org.jclouds.docker.domain.AutoValue_HostConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.docker.domain;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_HostConfig extends HostConfig {
private final String containerIDFile;
private final List binds;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy