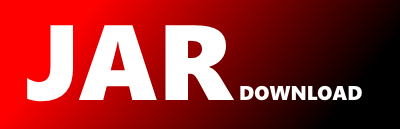
org.jclouds.ec2.domain.Reservation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jclouds.ec2.domain;
import static shaded.com.google.common.base.Preconditions.checkNotNull;
import java.util.Set;
import org.jclouds.javax.annotation.Nullable;
import shaded.com.google.common.base.MoreObjects;
import shaded.com.google.common.base.Objects;
import shaded.com.google.common.collect.ComparisonChain;
import shaded.com.google.common.collect.ForwardingSet;
import shaded.com.google.common.collect.ImmutableSet;
import shaded.com.google.common.collect.Ordering;
/**
*
* @see
*/
public class Reservation extends ForwardingSet implements Comparable> {
public static Builder builder() {
return new Builder();
}
public Builder toBuilder() {
return Reservation. builder().fromReservation(this);
}
public static class Builder {
private String region;
private String ownerId;
private String requesterId;
private String reservationId;
private ImmutableSet.Builder instances = ImmutableSet. builder();
private ImmutableSet.Builder groupNames = ImmutableSet. builder();
/**
* @see Reservation#getRegion()
*/
public Builder region(String region) {
this.region = region;
return this;
}
/**
* @see Reservation#getOwnerId()
*/
public Builder ownerId(String ownerId) {
this.ownerId = ownerId;
return this;
}
/**
* @see Reservation#getRequesterId()
*/
public Builder requesterId(String requesterId) {
this.requesterId = requesterId;
return this;
}
/**
* @see Reservation#getReservationId()
*/
public Builder reservationId(String reservationId) {
this.reservationId = reservationId;
return this;
}
/**
* @see Reservation#iterator
*/
public Builder instance(T instance) {
this.instances.add(checkNotNull(instance, "instance"));
return this;
}
/**
* @see Reservation#iterator
*/
public Builder instances(Set instances) {
this.instances.addAll(checkNotNull(instances, "instances"));
return this;
}
/**
* @see Reservation#getGroupNames()
*/
public Builder groupName(String groupName) {
this.groupNames.add(checkNotNull(groupName, "groupName"));
return this;
}
/**
* @see Reservation#getGroupNames()
*/
public Builder groupNames(Iterable groupNames) {
this.groupNames = ImmutableSet. builder().addAll(checkNotNull(groupNames, "groupNames"));
return this;
}
public Reservation build() {
return new Reservation(region, groupNames.build(), instances.build(), ownerId, requesterId, reservationId);
}
public Builder fromReservation(Reservation in) {
return region(in.region).ownerId(in.ownerId).requesterId(in.requesterId).reservationId(in.reservationId)
.instances(in).groupNames(in.groupNames);
}
}
private final String region;
private final ImmutableSet groupNames;
private final ImmutableSet instances;
@Nullable
private final String ownerId;
@Nullable
private final String requesterId;
@Nullable
private final String reservationId;
public Reservation(String region, Iterable groupNames, Iterable instances, @Nullable String ownerId,
@Nullable String requesterId, @Nullable String reservationId) {
this.region = checkNotNull(region, "region");
this.groupNames = ImmutableSet.copyOf(checkNotNull(groupNames, "groupNames"));
this.instances = ImmutableSet.copyOf(checkNotNull(instances, "instances"));
this.ownerId = ownerId;
this.requesterId = requesterId;
this.reservationId = reservationId;
}
@Override
protected Set delegate() {
return instances;
}
/**
* To be removed in jclouds 1.6 Warning
*
* Especially on EC2 clones that may not support regions, this value is fragile. Consider
* alternate means to determine context.
*/
@Deprecated
public String getRegion() {
return region;
}
/**
* Names of the security groups.
*/
public Set getGroupNames() {
return groupNames;
}
/**
* AWS Access Key ID of the user who owns the reservation.
*/
public String getOwnerId() {
return ownerId;
}
/**
* The ID of the requester that launched the instances on your behalf (for example, AWS
* Management Console or Auto Scaling).
*/
public String getRequesterId() {
return requesterId;
}
/**
* Unique ID of the reservation.
*/
public String getReservationId() {
return reservationId;
}
@Override
public int hashCode() {
return Objects.hashCode(region, reservationId, super.hashCode());
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null || getClass() != obj.getClass())
return false;
@SuppressWarnings("unchecked")
Reservation that = Reservation.class.cast(obj);
return super.equals(that) && Objects.equal(this.region, that.region)
&& Objects.equal(this.reservationId, that.reservationId);
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return MoreObjects.toStringHelper(this).omitNullValues().add("region", region).add("reservationId", reservationId)
.add("requesterId", requesterId).add("instances", instances).add("groupNames", groupNames).toString();
}
@Override
public int compareTo(Reservation other) {
return ComparisonChain.start().compare(region, other.region)
.compare(reservationId, other.reservationId, Ordering.natural().nullsLast()).result();
}
}