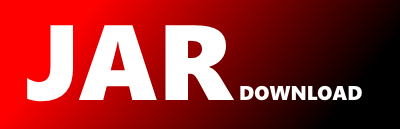
org.jclouds.gogrid.compute.strategy.GoGridComputeServiceAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jclouds.gogrid.compute.strategy;
import static shaded.com.google.common.base.Preconditions.checkNotNull;
import static org.jclouds.util.Predicates2.retry;
import java.security.SecureRandom;
import java.util.Set;
import javax.annotation.Resource;
import javax.inject.Inject;
import javax.inject.Singleton;
import org.jclouds.compute.ComputeServiceAdapter;
import org.jclouds.compute.domain.Hardware;
import org.jclouds.compute.domain.Template;
import org.jclouds.compute.reference.ComputeServiceConstants.Timeouts;
import org.jclouds.domain.LoginCredentials;
import org.jclouds.gogrid.GoGridApi;
import org.jclouds.gogrid.compute.suppliers.GoGridHardwareSupplier;
import org.jclouds.gogrid.domain.Ip;
import org.jclouds.gogrid.domain.IpType;
import org.jclouds.gogrid.domain.Option;
import org.jclouds.gogrid.domain.PowerCommand;
import org.jclouds.gogrid.domain.Server;
import org.jclouds.gogrid.domain.ServerImage;
import org.jclouds.gogrid.options.GetIpListOptions;
import org.jclouds.gogrid.predicates.ServerLatestJobCompleted;
import org.jclouds.logging.Logger;
import shaded.com.google.common.base.Function;
import shaded.com.google.common.base.Predicate;
import shaded.com.google.common.base.Throwables;
import shaded.com.google.common.collect.FluentIterable;
import shaded.com.google.common.collect.Iterables;
import shaded.com.google.common.primitives.Longs;
/**
* defines the connection between the {@link org.jclouds.gogrid.GoGridApi} implementation and the jclouds
* {@link ComputeService}
*/
@Singleton
public class GoGridComputeServiceAdapter implements ComputeServiceAdapter {
@Resource
protected Logger logger = Logger.NULL;
private final GoGridApi client;
private final Function sizeToRam;
private final Predicate serverLatestJobCompleted;
private final Predicate serverLatestJobCompletedShort;
@Inject
protected GoGridComputeServiceAdapter(GoGridApi client, Function sizeToRam, Timeouts timeouts) {
this.client = checkNotNull(client, "client");
this.sizeToRam = checkNotNull(sizeToRam, "sizeToRam");
this.serverLatestJobCompleted = retry(new ServerLatestJobCompleted(client.getJobServices()),
timeouts.nodeRunning * 9L / 10L);
this.serverLatestJobCompletedShort = retry(new ServerLatestJobCompleted(client.getJobServices()),
timeouts.nodeRunning * 1L / 10L);
}
@Override
public NodeAndInitialCredentials createNodeWithGroupEncodedIntoName(String group, String name,
Template template) {
Server addedServer = null;
boolean notStarted = true;
int numOfRetries = 20;
GetIpListOptions unassignedIps = new GetIpListOptions().onlyUnassigned().inDatacenter(
template.getLocation().getId()).onlyWithType(IpType.PUBLIC);
// lock-free consumption of a shared resource: IP address pool
while (notStarted) { // TODO: replace with Predicate-based thread
// collision avoidance for simplicity
Set availableIps = client.getIpServices().getIpList(unassignedIps);
if (availableIps.isEmpty())
throw new RuntimeException("No IPs available on this identity.");
int ipIndex = new SecureRandom().nextInt(availableIps.size());
Ip availableIp = Iterables.get(availableIps, ipIndex);
try {
addedServer = addServer(name, template, availableIp);
notStarted = false;
} catch (Exception e) {
if (--numOfRetries == 0)
Throwables.propagate(e);
notStarted = true;
}
}
if (template.getOptions().shouldBlockUntilRunning()) {
serverLatestJobCompleted.apply(addedServer);
client.getServerServices().power(addedServer.getName(), PowerCommand.START);
serverLatestJobCompletedShort.apply(addedServer);
addedServer = Iterables.getOnlyElement(client.getServerServices().getServersByName(addedServer.getName()));
}
LoginCredentials credentials = LoginCredentials.fromCredentials(client.getServerServices()
.getServerCredentialsList().get(addedServer.getName()));
return new NodeAndInitialCredentials(addedServer, addedServer.getId() + "", credentials);
}
private Server addServer(String name, Template template, Ip availableIp) {
Server addedServer = client.getServerServices().addServer(name,
checkNotNull(template.getImage().getProviderId()), sizeToRam.apply(template.getHardware()),
availableIp.getIp());
return addedServer;
}
@Override
public Iterable listHardwareProfiles() {
return GoGridHardwareSupplier.H_ALL;
}
@Override
public Iterable listImages() {
return client.getImageServices().getImageList();
}
@Override
public Iterable listNodes() {
return client.getServerServices().getServerList();
}
@Override
public Iterable listNodesByIds(final Iterable ids) {
Set idsAsLongs = FluentIterable.from(ids)
.transform(toLong())
.toSet();
return client.getServerServices().getServersById(Longs.toArray(idsAsLongs));
}
@Override
public Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy