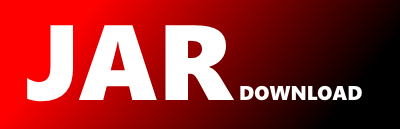
org.jclouds.googlecloudstorage.domain.AutoValue_GoogleCloudStorageObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.googlecloudstorage.domain;
import java.net.URI;
import java.util.Date;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_GoogleCloudStorageObject extends GoogleCloudStorageObject {
private final String id;
private final URI selfLink;
private final String etag;
private final String name;
private final String bucket;
private final long generation;
private final long metageneration;
private final String contentType;
private final Date updated;
private final Date timeDeleted;
private final DomainResourceReferences.StorageClass storageClass;
private final long size;
private final String md5Hash;
private final URI mediaLink;
private final Map metadata;
private final String contentEncoding;
private final String contentDisposition;
private final String contentLanguage;
private final String cacheControl;
private final List acl;
private final Owner owner;
private final String crc32c;
private final Integer componentCount;
AutoValue_GoogleCloudStorageObject(
String id,
URI selfLink,
String etag,
String name,
String bucket,
long generation,
long metageneration,
@Nullable String contentType,
Date updated,
@Nullable Date timeDeleted,
DomainResourceReferences.StorageClass storageClass,
long size,
@Nullable String md5Hash,
URI mediaLink,
Map metadata,
@Nullable String contentEncoding,
@Nullable String contentDisposition,
@Nullable String contentLanguage,
@Nullable String cacheControl,
List acl,
@Nullable Owner owner,
@Nullable String crc32c,
@Nullable Integer componentCount) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (selfLink == null) {
throw new NullPointerException("Null selfLink");
}
this.selfLink = selfLink;
if (etag == null) {
throw new NullPointerException("Null etag");
}
this.etag = etag;
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
if (bucket == null) {
throw new NullPointerException("Null bucket");
}
this.bucket = bucket;
this.generation = generation;
this.metageneration = metageneration;
this.contentType = contentType;
if (updated == null) {
throw new NullPointerException("Null updated");
}
this.updated = updated;
this.timeDeleted = timeDeleted;
if (storageClass == null) {
throw new NullPointerException("Null storageClass");
}
this.storageClass = storageClass;
this.size = size;
this.md5Hash = md5Hash;
if (mediaLink == null) {
throw new NullPointerException("Null mediaLink");
}
this.mediaLink = mediaLink;
if (metadata == null) {
throw new NullPointerException("Null metadata");
}
this.metadata = metadata;
this.contentEncoding = contentEncoding;
this.contentDisposition = contentDisposition;
this.contentLanguage = contentLanguage;
this.cacheControl = cacheControl;
if (acl == null) {
throw new NullPointerException("Null acl");
}
this.acl = acl;
this.owner = owner;
this.crc32c = crc32c;
this.componentCount = componentCount;
}
@Override
public String id() {
return id;
}
@Override
public URI selfLink() {
return selfLink;
}
@Override
public String etag() {
return etag;
}
@Override
public String name() {
return name;
}
@Override
public String bucket() {
return bucket;
}
@Override
public long generation() {
return generation;
}
@Override
public long metageneration() {
return metageneration;
}
@Nullable
@Override
public String contentType() {
return contentType;
}
@Override
public Date updated() {
return updated;
}
@Nullable
@Override
public Date timeDeleted() {
return timeDeleted;
}
@Override
public DomainResourceReferences.StorageClass storageClass() {
return storageClass;
}
@Override
public long size() {
return size;
}
@Nullable
@Override
public String md5Hash() {
return md5Hash;
}
@Override
public URI mediaLink() {
return mediaLink;
}
@Override
public Map metadata() {
return metadata;
}
@Nullable
@Override
public String contentEncoding() {
return contentEncoding;
}
@Nullable
@Override
public String contentDisposition() {
return contentDisposition;
}
@Nullable
@Override
public String contentLanguage() {
return contentLanguage;
}
@Nullable
@Override
public String cacheControl() {
return cacheControl;
}
@Override
public List acl() {
return acl;
}
@Nullable
@Override
public Owner owner() {
return owner;
}
@Nullable
@Override
public String crc32c() {
return crc32c;
}
@Nullable
@Override
public Integer componentCount() {
return componentCount;
}
@Override
public String toString() {
return "GoogleCloudStorageObject{"
+ "id=" + id + ", "
+ "selfLink=" + selfLink + ", "
+ "etag=" + etag + ", "
+ "name=" + name + ", "
+ "bucket=" + bucket + ", "
+ "generation=" + generation + ", "
+ "metageneration=" + metageneration + ", "
+ "contentType=" + contentType + ", "
+ "updated=" + updated + ", "
+ "timeDeleted=" + timeDeleted + ", "
+ "storageClass=" + storageClass + ", "
+ "size=" + size + ", "
+ "md5Hash=" + md5Hash + ", "
+ "mediaLink=" + mediaLink + ", "
+ "metadata=" + metadata + ", "
+ "contentEncoding=" + contentEncoding + ", "
+ "contentDisposition=" + contentDisposition + ", "
+ "contentLanguage=" + contentLanguage + ", "
+ "cacheControl=" + cacheControl + ", "
+ "acl=" + acl + ", "
+ "owner=" + owner + ", "
+ "crc32c=" + crc32c + ", "
+ "componentCount=" + componentCount
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof GoogleCloudStorageObject) {
GoogleCloudStorageObject that = (GoogleCloudStorageObject) o;
return (this.id.equals(that.id()))
&& (this.selfLink.equals(that.selfLink()))
&& (this.etag.equals(that.etag()))
&& (this.name.equals(that.name()))
&& (this.bucket.equals(that.bucket()))
&& (this.generation == that.generation())
&& (this.metageneration == that.metageneration())
&& ((this.contentType == null) ? (that.contentType() == null) : this.contentType.equals(that.contentType()))
&& (this.updated.equals(that.updated()))
&& ((this.timeDeleted == null) ? (that.timeDeleted() == null) : this.timeDeleted.equals(that.timeDeleted()))
&& (this.storageClass.equals(that.storageClass()))
&& (this.size == that.size())
&& ((this.md5Hash == null) ? (that.md5Hash() == null) : this.md5Hash.equals(that.md5Hash()))
&& (this.mediaLink.equals(that.mediaLink()))
&& (this.metadata.equals(that.metadata()))
&& ((this.contentEncoding == null) ? (that.contentEncoding() == null) : this.contentEncoding.equals(that.contentEncoding()))
&& ((this.contentDisposition == null) ? (that.contentDisposition() == null) : this.contentDisposition.equals(that.contentDisposition()))
&& ((this.contentLanguage == null) ? (that.contentLanguage() == null) : this.contentLanguage.equals(that.contentLanguage()))
&& ((this.cacheControl == null) ? (that.cacheControl() == null) : this.cacheControl.equals(that.cacheControl()))
&& (this.acl.equals(that.acl()))
&& ((this.owner == null) ? (that.owner() == null) : this.owner.equals(that.owner()))
&& ((this.crc32c == null) ? (that.crc32c() == null) : this.crc32c.equals(that.crc32c()))
&& ((this.componentCount == null) ? (that.componentCount() == null) : this.componentCount.equals(that.componentCount()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.selfLink.hashCode();
h *= 1000003;
h ^= this.etag.hashCode();
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= this.bucket.hashCode();
h *= 1000003;
h ^= (this.generation >>> 32) ^ this.generation;
h *= 1000003;
h ^= (this.metageneration >>> 32) ^ this.metageneration;
h *= 1000003;
h ^= (contentType == null) ? 0 : this.contentType.hashCode();
h *= 1000003;
h ^= this.updated.hashCode();
h *= 1000003;
h ^= (timeDeleted == null) ? 0 : this.timeDeleted.hashCode();
h *= 1000003;
h ^= this.storageClass.hashCode();
h *= 1000003;
h ^= (this.size >>> 32) ^ this.size;
h *= 1000003;
h ^= (md5Hash == null) ? 0 : this.md5Hash.hashCode();
h *= 1000003;
h ^= this.mediaLink.hashCode();
h *= 1000003;
h ^= this.metadata.hashCode();
h *= 1000003;
h ^= (contentEncoding == null) ? 0 : this.contentEncoding.hashCode();
h *= 1000003;
h ^= (contentDisposition == null) ? 0 : this.contentDisposition.hashCode();
h *= 1000003;
h ^= (contentLanguage == null) ? 0 : this.contentLanguage.hashCode();
h *= 1000003;
h ^= (cacheControl == null) ? 0 : this.cacheControl.hashCode();
h *= 1000003;
h ^= this.acl.hashCode();
h *= 1000003;
h ^= (owner == null) ? 0 : this.owner.hashCode();
h *= 1000003;
h ^= (crc32c == null) ? 0 : this.crc32c.hashCode();
h *= 1000003;
h ^= (componentCount == null) ? 0 : this.componentCount.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy