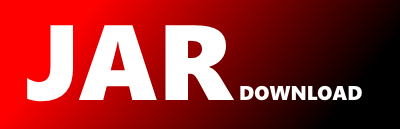
org.jclouds.googlecloudstorage.domain.AutoValue_ObjectAccessControls Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.googlecloudstorage.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ObjectAccessControls extends ObjectAccessControls {
private final String id;
private final String bucket;
private final String object;
private final Long generation;
private final String entity;
private final String entityId;
private final DomainResourceReferences.ObjectRole role;
private final String email;
private final String domain;
private final ProjectTeam projectTeam;
AutoValue_ObjectAccessControls(
@Nullable String id,
@Nullable String bucket,
@Nullable String object,
@Nullable Long generation,
String entity,
@Nullable String entityId,
DomainResourceReferences.ObjectRole role,
@Nullable String email,
@Nullable String domain,
@Nullable ProjectTeam projectTeam) {
this.id = id;
this.bucket = bucket;
this.object = object;
this.generation = generation;
if (entity == null) {
throw new NullPointerException("Null entity");
}
this.entity = entity;
this.entityId = entityId;
if (role == null) {
throw new NullPointerException("Null role");
}
this.role = role;
this.email = email;
this.domain = domain;
this.projectTeam = projectTeam;
}
@Nullable
@Override
public String id() {
return id;
}
@Nullable
@Override
public String bucket() {
return bucket;
}
@Nullable
@Override
public String object() {
return object;
}
@Nullable
@Override
public Long generation() {
return generation;
}
@Override
public String entity() {
return entity;
}
@Nullable
@Override
public String entityId() {
return entityId;
}
@Override
public DomainResourceReferences.ObjectRole role() {
return role;
}
@Nullable
@Override
public String email() {
return email;
}
@Nullable
@Override
public String domain() {
return domain;
}
@Nullable
@Override
public ProjectTeam projectTeam() {
return projectTeam;
}
@Override
public String toString() {
return "ObjectAccessControls{"
+ "id=" + id + ", "
+ "bucket=" + bucket + ", "
+ "object=" + object + ", "
+ "generation=" + generation + ", "
+ "entity=" + entity + ", "
+ "entityId=" + entityId + ", "
+ "role=" + role + ", "
+ "email=" + email + ", "
+ "domain=" + domain + ", "
+ "projectTeam=" + projectTeam
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ObjectAccessControls) {
ObjectAccessControls that = (ObjectAccessControls) o;
return ((this.id == null) ? (that.id() == null) : this.id.equals(that.id()))
&& ((this.bucket == null) ? (that.bucket() == null) : this.bucket.equals(that.bucket()))
&& ((this.object == null) ? (that.object() == null) : this.object.equals(that.object()))
&& ((this.generation == null) ? (that.generation() == null) : this.generation.equals(that.generation()))
&& (this.entity.equals(that.entity()))
&& ((this.entityId == null) ? (that.entityId() == null) : this.entityId.equals(that.entityId()))
&& (this.role.equals(that.role()))
&& ((this.email == null) ? (that.email() == null) : this.email.equals(that.email()))
&& ((this.domain == null) ? (that.domain() == null) : this.domain.equals(that.domain()))
&& ((this.projectTeam == null) ? (that.projectTeam() == null) : this.projectTeam.equals(that.projectTeam()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (id == null) ? 0 : this.id.hashCode();
h *= 1000003;
h ^= (bucket == null) ? 0 : this.bucket.hashCode();
h *= 1000003;
h ^= (object == null) ? 0 : this.object.hashCode();
h *= 1000003;
h ^= (generation == null) ? 0 : this.generation.hashCode();
h *= 1000003;
h ^= this.entity.hashCode();
h *= 1000003;
h ^= (entityId == null) ? 0 : this.entityId.hashCode();
h *= 1000003;
h ^= this.role.hashCode();
h *= 1000003;
h ^= (email == null) ? 0 : this.email.hashCode();
h *= 1000003;
h ^= (domain == null) ? 0 : this.domain.hashCode();
h *= 1000003;
h ^= (projectTeam == null) ? 0 : this.projectTeam.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy