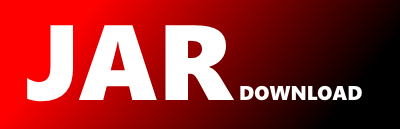
org.jclouds.googlecomputeengine.compute.config.GoogleComputeEngineServiceContextModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jclouds.googlecomputeengine.compute.config;
import static shaded.com.google.common.base.Suppliers.memoizeWithExpiration;
import static java.util.concurrent.TimeUnit.MILLISECONDS;
import static java.util.concurrent.TimeUnit.SECONDS;
import static org.jclouds.Constants.PROPERTY_SESSION_INTERVAL;
import static org.jclouds.googlecomputeengine.config.GoogleComputeEngineProperties.OPERATION_COMPLETE_INTERVAL;
import static org.jclouds.googlecomputeengine.config.GoogleComputeEngineProperties.OPERATION_COMPLETE_TIMEOUT;
import static org.jclouds.rest.config.BinderUtils.bindHttpApi;
import static org.jclouds.util.Predicates2.retry;
import java.net.URI;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.atomic.AtomicReference;
import javax.inject.Named;
import javax.inject.Singleton;
import org.jclouds.collect.Memoized;
import org.jclouds.compute.ComputeService;
import org.jclouds.compute.ComputeServiceAdapter;
import org.jclouds.compute.config.ComputeServiceAdapterContextModule;
import org.jclouds.compute.domain.Hardware;
import org.jclouds.compute.domain.NodeMetadata;
import org.jclouds.compute.domain.OperatingSystem;
import org.jclouds.compute.domain.OsFamily;
import org.jclouds.compute.options.TemplateOptions;
import org.jclouds.domain.Location;
import org.jclouds.domain.LoginCredentials;
import org.jclouds.googlecomputeengine.compute.GoogleComputeEngineService;
import org.jclouds.googlecomputeengine.compute.GoogleComputeEngineServiceAdapter;
import org.jclouds.googlecomputeengine.compute.functions.FirewallTagNamingConvention;
import org.jclouds.googlecomputeengine.compute.functions.GoogleComputeEngineImageToImage;
import org.jclouds.googlecomputeengine.compute.functions.ImageNameToOperatingSystem;
import org.jclouds.googlecomputeengine.compute.functions.InstanceToNodeMetadata;
import org.jclouds.googlecomputeengine.compute.functions.MachineTypeToHardware;
import org.jclouds.googlecomputeengine.compute.functions.OrphanedGroupsFromDeadNodes;
import org.jclouds.googlecomputeengine.compute.functions.Resources;
import org.jclouds.googlecomputeengine.compute.functions.ResetWindowsPassword;
import org.jclouds.googlecomputeengine.compute.loaders.DiskURIToImage;
import org.jclouds.googlecomputeengine.compute.loaders.SubnetworkLoader;
import org.jclouds.googlecomputeengine.compute.options.GoogleComputeEngineTemplateOptions;
import org.jclouds.googlecomputeengine.compute.predicates.AtomicInstanceVisible;
import org.jclouds.googlecomputeengine.compute.predicates.AtomicOperationDone;
import org.jclouds.googlecomputeengine.compute.predicates.GroupIsEmpty;
import org.jclouds.googlecomputeengine.compute.strategy.CreateNodesWithGroupEncodedIntoNameThenAddToSet;
import org.jclouds.googlecomputeengine.domain.Image;
import org.jclouds.googlecomputeengine.domain.Instance;
import org.jclouds.googlecomputeengine.domain.MachineType;
import org.jclouds.googlecomputeengine.domain.Operation;
import org.jclouds.googlecomputeengine.domain.Subnetwork;
import org.jclouds.location.suppliers.ImplicitLocationSupplier;
import org.jclouds.location.suppliers.implicit.FirstZone;
import shaded.com.google.common.base.Optional;
import shaded.com.google.common.base.Function;
import shaded.com.google.common.base.Functions;
import shaded.com.google.common.base.Predicate;
import shaded.com.google.common.base.Supplier;
import shaded.com.google.common.cache.CacheBuilder;
import shaded.com.google.common.cache.CacheLoader;
import shaded.com.google.common.cache.LoadingCache;
import shaded.com.google.common.collect.ImmutableMap;
import shaded.com.google.inject.Injector;
import shaded.com.google.inject.Provides;
import shaded.com.google.inject.Scopes;
import shaded.com.google.inject.TypeLiteral;
import org.jclouds.compute.domain.internal.TemplateBuilderImpl;
import org.jclouds.compute.functions.NodeAndTemplateOptionsToStatement;
import org.jclouds.compute.functions.NodeAndTemplateOptionsToStatementWithoutPublicKey;
import org.jclouds.googlecomputeengine.compute.domain.internal.GoogleComputeEngineArbitraryCpuRamTemplateBuilderImpl;
import org.jclouds.googlecomputeengine.compute.domain.internal.RegionAndName;
public final class GoogleComputeEngineServiceContextModule
extends ComputeServiceAdapterContextModule {
@Override
protected void configure() {
super.configure();
bind(ComputeService.class).to(GoogleComputeEngineService.class);
bind(new TypeLiteral>() {
}).to(GoogleComputeEngineServiceAdapter.class);
bind(TemplateBuilderImpl.class).to(GoogleComputeEngineArbitraryCpuRamTemplateBuilderImpl.class);
// Use compute service to supply locations, which are always zones.
install(new LocationsFromComputeServiceAdapterModule() {
});
bind(new TypeLiteral>() {
}).toInstance(Functions.identity());
bind(ImplicitLocationSupplier.class).to(FirstZone.class);
bind(new TypeLiteral>() {
}).to(InstanceToNodeMetadata.class);
bind(new TypeLiteral>() {
}).to(MachineTypeToHardware.class);
bind(new TypeLiteral>() {
}).to(GoogleComputeEngineImageToImage.class);
bind(org.jclouds.compute.strategy.impl.CreateNodesWithGroupEncodedIntoNameThenAddToSet.class)
.to(CreateNodesWithGroupEncodedIntoNameThenAddToSet.class);
bind(TemplateOptions.class).to(GoogleComputeEngineTemplateOptions.class);
bind(NodeAndTemplateOptionsToStatement.class).to(NodeAndTemplateOptionsToStatementWithoutPublicKey.class);
bind(new TypeLiteral, Set>>() {
}).to(OrphanedGroupsFromDeadNodes.class);
bind(new TypeLiteral>() {
}).to(GroupIsEmpty.class);
bind(new TypeLiteral>() {
}).to(ImageNameToOperatingSystem.class);
bind(new TypeLiteral, String>>() {
}).to(ResetWindowsPassword.class);
bind(FirewallTagNamingConvention.Factory.class).in(Scopes.SINGLETON);
bind(new TypeLiteral>>() {
}).to(DiskURIToImage.class);
bind(new TypeLiteral>>() {
}).to(SubnetworkLoader.class);
bindHttpApi(binder(), Resources.class);
}
// TODO: these timeouts need thinking through.
@Provides Predicate> operationDone(AtomicOperationDone input,
@Named(OPERATION_COMPLETE_TIMEOUT) long timeout, @Named(OPERATION_COMPLETE_INTERVAL) long interval) {
return retry(input, timeout, interval, MILLISECONDS);
}
@Provides Predicate> instanceVisible(AtomicInstanceVisible input,
@Named(OPERATION_COMPLETE_TIMEOUT) long timeout, @Named(OPERATION_COMPLETE_INTERVAL) long interval) {
return retry(input, timeout, interval, MILLISECONDS);
}
@Provides @Singleton @Memoized Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy