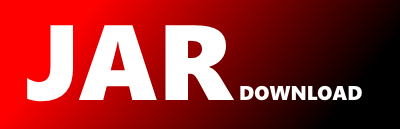
org.jclouds.googlecomputeengine.domain.AutoValue_Address Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.googlecomputeengine.domain;
import java.net.URI;
import java.util.Date;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Address extends Address {
private final String id;
private final URI selfLink;
private final String name;
private final Date creationTimestamp;
private final String description;
private final Address.Status status;
private final List users;
private final URI region;
private final String address;
AutoValue_Address(
String id,
URI selfLink,
String name,
Date creationTimestamp,
@Nullable String description,
Address.Status status,
@Nullable List users,
URI region,
String address) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (selfLink == null) {
throw new NullPointerException("Null selfLink");
}
this.selfLink = selfLink;
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
if (creationTimestamp == null) {
throw new NullPointerException("Null creationTimestamp");
}
this.creationTimestamp = creationTimestamp;
this.description = description;
if (status == null) {
throw new NullPointerException("Null status");
}
this.status = status;
this.users = users;
if (region == null) {
throw new NullPointerException("Null region");
}
this.region = region;
if (address == null) {
throw new NullPointerException("Null address");
}
this.address = address;
}
@Override
public String id() {
return id;
}
@Override
public URI selfLink() {
return selfLink;
}
@Override
public String name() {
return name;
}
@Override
public Date creationTimestamp() {
return creationTimestamp;
}
@Nullable
@Override
public String description() {
return description;
}
@Override
public Address.Status status() {
return status;
}
@Nullable
@Override
public List users() {
return users;
}
@Override
public URI region() {
return region;
}
@Override
public String address() {
return address;
}
@Override
public String toString() {
return "Address{"
+ "id=" + id + ", "
+ "selfLink=" + selfLink + ", "
+ "name=" + name + ", "
+ "creationTimestamp=" + creationTimestamp + ", "
+ "description=" + description + ", "
+ "status=" + status + ", "
+ "users=" + users + ", "
+ "region=" + region + ", "
+ "address=" + address
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Address) {
Address that = (Address) o;
return (this.id.equals(that.id()))
&& (this.selfLink.equals(that.selfLink()))
&& (this.name.equals(that.name()))
&& (this.creationTimestamp.equals(that.creationTimestamp()))
&& ((this.description == null) ? (that.description() == null) : this.description.equals(that.description()))
&& (this.status.equals(that.status()))
&& ((this.users == null) ? (that.users() == null) : this.users.equals(that.users()))
&& (this.region.equals(that.region()))
&& (this.address.equals(that.address()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.selfLink.hashCode();
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= this.creationTimestamp.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : this.description.hashCode();
h *= 1000003;
h ^= this.status.hashCode();
h *= 1000003;
h ^= (users == null) ? 0 : this.users.hashCode();
h *= 1000003;
h ^= this.region.hashCode();
h *= 1000003;
h ^= this.address.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy