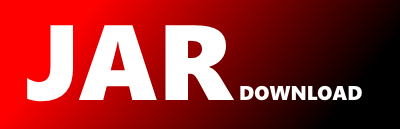
org.jclouds.googlecomputeengine.domain.AutoValue_UrlMap_PathMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.googlecomputeengine.domain;
import java.net.URI;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_UrlMap_PathMatcher extends UrlMap.PathMatcher {
private final String name;
private final String description;
private final URI defaultService;
private final List pathRules;
AutoValue_UrlMap_PathMatcher(
String name,
@Nullable String description,
URI defaultService,
List pathRules) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
this.description = description;
if (defaultService == null) {
throw new NullPointerException("Null defaultService");
}
this.defaultService = defaultService;
if (pathRules == null) {
throw new NullPointerException("Null pathRules");
}
this.pathRules = pathRules;
}
@Override
public String name() {
return name;
}
@Nullable
@Override
public String description() {
return description;
}
@Override
public URI defaultService() {
return defaultService;
}
@Override
public List pathRules() {
return pathRules;
}
@Override
public String toString() {
return "PathMatcher{"
+ "name=" + name + ", "
+ "description=" + description + ", "
+ "defaultService=" + defaultService + ", "
+ "pathRules=" + pathRules
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof UrlMap.PathMatcher) {
UrlMap.PathMatcher that = (UrlMap.PathMatcher) o;
return (this.name.equals(that.name()))
&& ((this.description == null) ? (that.description() == null) : this.description.equals(that.description()))
&& (this.defaultService.equals(that.defaultService()))
&& (this.pathRules.equals(that.pathRules()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : this.description.hashCode();
h *= 1000003;
h ^= this.defaultService.hashCode();
h *= 1000003;
h ^= this.pathRules.hashCode();
return h;
}
}