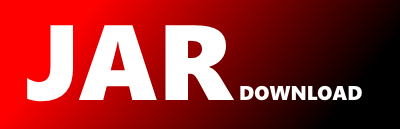
org.jclouds.googlecomputeengine.options.AutoValue_BackendServiceOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.googlecomputeengine.options;
import java.net.URI;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.googlecomputeengine.domain.BackendService;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_BackendServiceOptions extends BackendServiceOptions {
private final String name;
private final String description;
private final List healthChecks;
private final List backends;
private final Integer timeoutSec;
private final Integer port;
private final String protocol;
private final String fingerprint;
private final String portName;
AutoValue_BackendServiceOptions(
@Nullable String name,
@Nullable String description,
@Nullable List healthChecks,
@Nullable List backends,
@Nullable Integer timeoutSec,
@Nullable Integer port,
@Nullable String protocol,
@Nullable String fingerprint,
@Nullable String portName) {
this.name = name;
this.description = description;
this.healthChecks = healthChecks;
this.backends = backends;
this.timeoutSec = timeoutSec;
this.port = port;
this.protocol = protocol;
this.fingerprint = fingerprint;
this.portName = portName;
}
@Nullable
@Override
public String name() {
return name;
}
@Nullable
@Override
public String description() {
return description;
}
@Nullable
@Override
public List healthChecks() {
return healthChecks;
}
@Nullable
@Override
public List backends() {
return backends;
}
@Nullable
@Override
public Integer timeoutSec() {
return timeoutSec;
}
@Nullable
@Override
public Integer port() {
return port;
}
@Nullable
@Override
public String protocol() {
return protocol;
}
@Nullable
@Override
public String fingerprint() {
return fingerprint;
}
@Nullable
@Override
public String portName() {
return portName;
}
@Override
public String toString() {
return "BackendServiceOptions{"
+ "name=" + name + ", "
+ "description=" + description + ", "
+ "healthChecks=" + healthChecks + ", "
+ "backends=" + backends + ", "
+ "timeoutSec=" + timeoutSec + ", "
+ "port=" + port + ", "
+ "protocol=" + protocol + ", "
+ "fingerprint=" + fingerprint + ", "
+ "portName=" + portName
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof BackendServiceOptions) {
BackendServiceOptions that = (BackendServiceOptions) o;
return ((this.name == null) ? (that.name() == null) : this.name.equals(that.name()))
&& ((this.description == null) ? (that.description() == null) : this.description.equals(that.description()))
&& ((this.healthChecks == null) ? (that.healthChecks() == null) : this.healthChecks.equals(that.healthChecks()))
&& ((this.backends == null) ? (that.backends() == null) : this.backends.equals(that.backends()))
&& ((this.timeoutSec == null) ? (that.timeoutSec() == null) : this.timeoutSec.equals(that.timeoutSec()))
&& ((this.port == null) ? (that.port() == null) : this.port.equals(that.port()))
&& ((this.protocol == null) ? (that.protocol() == null) : this.protocol.equals(that.protocol()))
&& ((this.fingerprint == null) ? (that.fingerprint() == null) : this.fingerprint.equals(that.fingerprint()))
&& ((this.portName == null) ? (that.portName() == null) : this.portName.equals(that.portName()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (name == null) ? 0 : this.name.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : this.description.hashCode();
h *= 1000003;
h ^= (healthChecks == null) ? 0 : this.healthChecks.hashCode();
h *= 1000003;
h ^= (backends == null) ? 0 : this.backends.hashCode();
h *= 1000003;
h ^= (timeoutSec == null) ? 0 : this.timeoutSec.hashCode();
h *= 1000003;
h ^= (port == null) ? 0 : this.port.hashCode();
h *= 1000003;
h ^= (protocol == null) ? 0 : this.protocol.hashCode();
h *= 1000003;
h ^= (fingerprint == null) ? 0 : this.fingerprint.hashCode();
h *= 1000003;
h ^= (portName == null) ? 0 : this.portName.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy