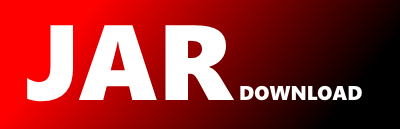
org.jclouds.googlecomputeengine.options.AutoValue_TargetPoolCreationOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.googlecomputeengine.options;
import java.net.URI;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TargetPoolCreationOptions extends TargetPoolCreationOptions {
private final String name;
private final List healthChecks;
private final List instances;
private final TargetPoolCreationOptions.SessionAffinityValue sessionAffinity;
private final Float failoverRatio;
private final URI backupPool;
private final String description;
AutoValue_TargetPoolCreationOptions(
String name,
@Nullable List healthChecks,
@Nullable List instances,
@Nullable TargetPoolCreationOptions.SessionAffinityValue sessionAffinity,
@Nullable Float failoverRatio,
@Nullable URI backupPool,
@Nullable String description) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
this.healthChecks = healthChecks;
this.instances = instances;
this.sessionAffinity = sessionAffinity;
this.failoverRatio = failoverRatio;
this.backupPool = backupPool;
this.description = description;
}
@Override
public String name() {
return name;
}
@Nullable
@Override
public List healthChecks() {
return healthChecks;
}
@Nullable
@Override
public List instances() {
return instances;
}
@Nullable
@Override
public TargetPoolCreationOptions.SessionAffinityValue sessionAffinity() {
return sessionAffinity;
}
@Nullable
@Override
public Float failoverRatio() {
return failoverRatio;
}
@Nullable
@Override
public URI backupPool() {
return backupPool;
}
@Nullable
@Override
public String description() {
return description;
}
@Override
public String toString() {
return "TargetPoolCreationOptions{"
+ "name=" + name + ", "
+ "healthChecks=" + healthChecks + ", "
+ "instances=" + instances + ", "
+ "sessionAffinity=" + sessionAffinity + ", "
+ "failoverRatio=" + failoverRatio + ", "
+ "backupPool=" + backupPool + ", "
+ "description=" + description
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TargetPoolCreationOptions) {
TargetPoolCreationOptions that = (TargetPoolCreationOptions) o;
return (this.name.equals(that.name()))
&& ((this.healthChecks == null) ? (that.healthChecks() == null) : this.healthChecks.equals(that.healthChecks()))
&& ((this.instances == null) ? (that.instances() == null) : this.instances.equals(that.instances()))
&& ((this.sessionAffinity == null) ? (that.sessionAffinity() == null) : this.sessionAffinity.equals(that.sessionAffinity()))
&& ((this.failoverRatio == null) ? (that.failoverRatio() == null) : this.failoverRatio.equals(that.failoverRatio()))
&& ((this.backupPool == null) ? (that.backupPool() == null) : this.backupPool.equals(that.backupPool()))
&& ((this.description == null) ? (that.description() == null) : this.description.equals(that.description()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= (healthChecks == null) ? 0 : this.healthChecks.hashCode();
h *= 1000003;
h ^= (instances == null) ? 0 : this.instances.hashCode();
h *= 1000003;
h ^= (sessionAffinity == null) ? 0 : this.sessionAffinity.hashCode();
h *= 1000003;
h ^= (failoverRatio == null) ? 0 : this.failoverRatio.hashCode();
h *= 1000003;
h ^= (backupPool == null) ? 0 : this.backupPool.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : this.description.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy