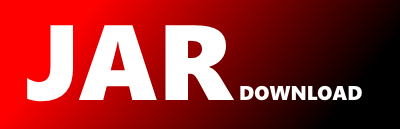
org.jclouds.openstack.neutron.v2.domain.AutoValue_UpdateFirewallRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.openstack.neutron.v2.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_UpdateFirewallRule extends UpdateFirewallRule {
private final String tenantId;
private final String name;
private final String description;
private final String firewallPolicyId;
private final Boolean shared;
private final String protocol;
private final IpVersion ipVersion;
private final String sourceIpAddress;
private final String destinationIpAddress;
private final String sourcePort;
private final String destinationPort;
private final Integer position;
private final String action;
private final Boolean enabled;
private AutoValue_UpdateFirewallRule(
@Nullable String tenantId,
@Nullable String name,
@Nullable String description,
@Nullable String firewallPolicyId,
@Nullable Boolean shared,
@Nullable String protocol,
@Nullable IpVersion ipVersion,
@Nullable String sourceIpAddress,
@Nullable String destinationIpAddress,
@Nullable String sourcePort,
@Nullable String destinationPort,
@Nullable Integer position,
@Nullable String action,
@Nullable Boolean enabled) {
this.tenantId = tenantId;
this.name = name;
this.description = description;
this.firewallPolicyId = firewallPolicyId;
this.shared = shared;
this.protocol = protocol;
this.ipVersion = ipVersion;
this.sourceIpAddress = sourceIpAddress;
this.destinationIpAddress = destinationIpAddress;
this.sourcePort = sourcePort;
this.destinationPort = destinationPort;
this.position = position;
this.action = action;
this.enabled = enabled;
}
@Nullable
@Override
public String getTenantId() {
return tenantId;
}
@Nullable
@Override
public String getName() {
return name;
}
@Nullable
@Override
public String getDescription() {
return description;
}
@Nullable
@Override
public String getFirewallPolicyId() {
return firewallPolicyId;
}
@Nullable
@Override
public Boolean getShared() {
return shared;
}
@Nullable
@Override
public String getProtocol() {
return protocol;
}
@Nullable
@Override
public IpVersion getIpVersion() {
return ipVersion;
}
@Nullable
@Override
public String getSourceIpAddress() {
return sourceIpAddress;
}
@Nullable
@Override
public String getDestinationIpAddress() {
return destinationIpAddress;
}
@Nullable
@Override
public String getSourcePort() {
return sourcePort;
}
@Nullable
@Override
public String getDestinationPort() {
return destinationPort;
}
@Nullable
@Override
public Integer getPosition() {
return position;
}
@Nullable
@Override
public String getAction() {
return action;
}
@Nullable
@Override
public Boolean getEnabled() {
return enabled;
}
@Override
public String toString() {
return "UpdateFirewallRule{"
+ "tenantId=" + tenantId + ", "
+ "name=" + name + ", "
+ "description=" + description + ", "
+ "firewallPolicyId=" + firewallPolicyId + ", "
+ "shared=" + shared + ", "
+ "protocol=" + protocol + ", "
+ "ipVersion=" + ipVersion + ", "
+ "sourceIpAddress=" + sourceIpAddress + ", "
+ "destinationIpAddress=" + destinationIpAddress + ", "
+ "sourcePort=" + sourcePort + ", "
+ "destinationPort=" + destinationPort + ", "
+ "position=" + position + ", "
+ "action=" + action + ", "
+ "enabled=" + enabled
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof UpdateFirewallRule) {
UpdateFirewallRule that = (UpdateFirewallRule) o;
return ((this.tenantId == null) ? (that.getTenantId() == null) : this.tenantId.equals(that.getTenantId()))
&& ((this.name == null) ? (that.getName() == null) : this.name.equals(that.getName()))
&& ((this.description == null) ? (that.getDescription() == null) : this.description.equals(that.getDescription()))
&& ((this.firewallPolicyId == null) ? (that.getFirewallPolicyId() == null) : this.firewallPolicyId.equals(that.getFirewallPolicyId()))
&& ((this.shared == null) ? (that.getShared() == null) : this.shared.equals(that.getShared()))
&& ((this.protocol == null) ? (that.getProtocol() == null) : this.protocol.equals(that.getProtocol()))
&& ((this.ipVersion == null) ? (that.getIpVersion() == null) : this.ipVersion.equals(that.getIpVersion()))
&& ((this.sourceIpAddress == null) ? (that.getSourceIpAddress() == null) : this.sourceIpAddress.equals(that.getSourceIpAddress()))
&& ((this.destinationIpAddress == null) ? (that.getDestinationIpAddress() == null) : this.destinationIpAddress.equals(that.getDestinationIpAddress()))
&& ((this.sourcePort == null) ? (that.getSourcePort() == null) : this.sourcePort.equals(that.getSourcePort()))
&& ((this.destinationPort == null) ? (that.getDestinationPort() == null) : this.destinationPort.equals(that.getDestinationPort()))
&& ((this.position == null) ? (that.getPosition() == null) : this.position.equals(that.getPosition()))
&& ((this.action == null) ? (that.getAction() == null) : this.action.equals(that.getAction()))
&& ((this.enabled == null) ? (that.getEnabled() == null) : this.enabled.equals(that.getEnabled()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (tenantId == null) ? 0 : this.tenantId.hashCode();
h *= 1000003;
h ^= (name == null) ? 0 : this.name.hashCode();
h *= 1000003;
h ^= (description == null) ? 0 : this.description.hashCode();
h *= 1000003;
h ^= (firewallPolicyId == null) ? 0 : this.firewallPolicyId.hashCode();
h *= 1000003;
h ^= (shared == null) ? 0 : this.shared.hashCode();
h *= 1000003;
h ^= (protocol == null) ? 0 : this.protocol.hashCode();
h *= 1000003;
h ^= (ipVersion == null) ? 0 : this.ipVersion.hashCode();
h *= 1000003;
h ^= (sourceIpAddress == null) ? 0 : this.sourceIpAddress.hashCode();
h *= 1000003;
h ^= (destinationIpAddress == null) ? 0 : this.destinationIpAddress.hashCode();
h *= 1000003;
h ^= (sourcePort == null) ? 0 : this.sourcePort.hashCode();
h *= 1000003;
h ^= (destinationPort == null) ? 0 : this.destinationPort.hashCode();
h *= 1000003;
h ^= (position == null) ? 0 : this.position.hashCode();
h *= 1000003;
h ^= (action == null) ? 0 : this.action.hashCode();
h *= 1000003;
h ^= (enabled == null) ? 0 : this.enabled.hashCode();
return h;
}
@Override
public UpdateFirewallRule.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends UpdateFirewallRule.Builder {
private String tenantId;
private String name;
private String description;
private String firewallPolicyId;
private Boolean shared;
private String protocol;
private IpVersion ipVersion;
private String sourceIpAddress;
private String destinationIpAddress;
private String sourcePort;
private String destinationPort;
private Integer position;
private String action;
private Boolean enabled;
Builder() {
}
private Builder(UpdateFirewallRule source) {
this.tenantId = source.getTenantId();
this.name = source.getName();
this.description = source.getDescription();
this.firewallPolicyId = source.getFirewallPolicyId();
this.shared = source.getShared();
this.protocol = source.getProtocol();
this.ipVersion = source.getIpVersion();
this.sourceIpAddress = source.getSourceIpAddress();
this.destinationIpAddress = source.getDestinationIpAddress();
this.sourcePort = source.getSourcePort();
this.destinationPort = source.getDestinationPort();
this.position = source.getPosition();
this.action = source.getAction();
this.enabled = source.getEnabled();
}
@Override
public UpdateFirewallRule.Builder tenantId(@Nullable String tenantId) {
this.tenantId = tenantId;
return this;
}
@Override
@Nullable public String getTenantId() {
return tenantId;
}
@Override
public UpdateFirewallRule.Builder name(@Nullable String name) {
this.name = name;
return this;
}
@Override
@Nullable public String getName() {
return name;
}
@Override
public UpdateFirewallRule.Builder description(@Nullable String description) {
this.description = description;
return this;
}
@Override
@Nullable public String getDescription() {
return description;
}
@Override
public UpdateFirewallRule.Builder firewallPolicyId(@Nullable String firewallPolicyId) {
this.firewallPolicyId = firewallPolicyId;
return this;
}
@Override
@Nullable public String getFirewallPolicyId() {
return firewallPolicyId;
}
@Override
public UpdateFirewallRule.Builder shared(@Nullable Boolean shared) {
this.shared = shared;
return this;
}
@Override
@Nullable public Boolean getShared() {
return shared;
}
@Override
public UpdateFirewallRule.Builder protocol(@Nullable String protocol) {
this.protocol = protocol;
return this;
}
@Override
@Nullable public String getProtocol() {
return protocol;
}
@Override
public UpdateFirewallRule.Builder ipVersion(@Nullable IpVersion ipVersion) {
this.ipVersion = ipVersion;
return this;
}
@Override
@Nullable public IpVersion getIpVersion() {
return ipVersion;
}
@Override
public UpdateFirewallRule.Builder sourceIpAddress(@Nullable String sourceIpAddress) {
this.sourceIpAddress = sourceIpAddress;
return this;
}
@Override
@Nullable public String getSourceIpAddress() {
return sourceIpAddress;
}
@Override
public UpdateFirewallRule.Builder destinationIpAddress(@Nullable String destinationIpAddress) {
this.destinationIpAddress = destinationIpAddress;
return this;
}
@Override
@Nullable public String getDestinationIpAddress() {
return destinationIpAddress;
}
@Override
public UpdateFirewallRule.Builder sourcePort(@Nullable String sourcePort) {
this.sourcePort = sourcePort;
return this;
}
@Override
@Nullable public String getSourcePort() {
return sourcePort;
}
@Override
public UpdateFirewallRule.Builder destinationPort(@Nullable String destinationPort) {
this.destinationPort = destinationPort;
return this;
}
@Override
@Nullable public String getDestinationPort() {
return destinationPort;
}
@Override
public UpdateFirewallRule.Builder position(@Nullable Integer position) {
this.position = position;
return this;
}
@Override
@Nullable public Integer getPosition() {
return position;
}
@Override
public UpdateFirewallRule.Builder action(@Nullable String action) {
this.action = action;
return this;
}
@Override
@Nullable public String getAction() {
return action;
}
@Override
public UpdateFirewallRule.Builder enabled(@Nullable Boolean enabled) {
this.enabled = enabled;
return this;
}
@Override
@Nullable public Boolean getEnabled() {
return enabled;
}
@Override
public UpdateFirewallRule build() {
return new AutoValue_UpdateFirewallRule(
this.tenantId,
this.name,
this.description,
this.firewallPolicyId,
this.shared,
this.protocol,
this.ipVersion,
this.sourceIpAddress,
this.destinationIpAddress,
this.sourcePort,
this.destinationPort,
this.position,
this.action,
this.enabled);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy