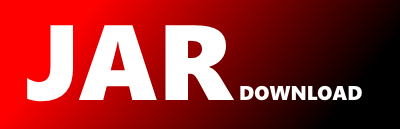
org.jclouds.openstack.v2_0.functions.PresentWhenExtensionAnnotationMatchesExtensionSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jclouds.openstack.v2_0.functions;
import static shaded.com.google.common.base.Preconditions.checkNotNull;
import static shaded.com.google.common.collect.Iterables.any;
import static org.jclouds.openstack.v2_0.predicates.ExtensionPredicates.aliasEquals;
import static org.jclouds.openstack.v2_0.predicates.ExtensionPredicates.nameEquals;
import static org.jclouds.openstack.v2_0.predicates.ExtensionPredicates.namespaceOrAliasEquals;
import static org.jclouds.util.Optionals2.unwrapIfOptional;
import java.net.URI;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.inject.Inject;
import org.jclouds.openstack.keystone.v2_0.config.NamespaceAliases;
import org.jclouds.openstack.v2_0.domain.Extension;
import org.jclouds.reflect.InvocationSuccess;
import org.jclouds.rest.functions.ImplicitOptionalConverter;
import shaded.com.google.common.base.Optional;
import shaded.com.google.common.cache.LoadingCache;
import shaded.com.google.common.collect.ImmutableMap;
import shaded.com.google.common.collect.Sets;
/**
* We use the annotation {@link Extension} to bind a class that implements an extension
* API to an {@link Extension}.
*
* Match in the following order:
*
* 1. Match by namespace
* 2. Match by namespace aliases
* 3. Match by alias
* 4. Match by name
*
* New versions of openstack have no namespaces anymore.
* Alias is different than a namespace alias - it's an alternative namespace URL to match against.
*/
public class PresentWhenExtensionAnnotationMatchesExtensionSet implements
ImplicitOptionalConverter {
private final LoadingCache> extensions;
private final Map> aliases;
@Inject
PresentWhenExtensionAnnotationMatchesExtensionSet(
LoadingCache> extensions, @NamespaceAliases Map> aliases) {
this.extensions = extensions;
this.aliases = aliases == null ? ImmutableMap.> of() : ImmutableMap.copyOf(aliases);
}
private boolean checkExtension(String invocationArg, URI namespace,
Set aliasesForNamespace, String alias, String name) {
if (any(extensions.getUnchecked(invocationArg), namespaceOrAliasEquals(namespace, aliasesForNamespace)))
return true;
// Could not find extension by namespace or namespace alias. Try to find it by alias next:
if ( !"".equals(alias)) {
if (any(extensions.getUnchecked(invocationArg), aliasEquals(alias)))
return true;
}
// Could not find extension by namespace or namespace alias or alias. Try to find it by name next:
if ( !"".equals(name)) {
if (any(extensions.getUnchecked(invocationArg), nameEquals(name)))
return true;
}
return false;
}
@Override
public Optional