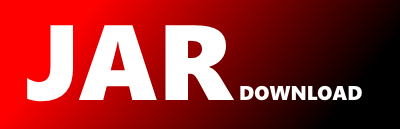
org.jclouds.packet.domain.AutoValue_IpAddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.packet.domain;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_IpAddress extends IpAddress {
private final String id;
private final Integer addressFamily;
private final String netmask;
private final Boolean publicAddress;
private final Integer cidr;
private final Boolean management;
private final Boolean manageable;
private final Href assignedTo;
private final String network;
private final String address;
private final String gateway;
private final String href;
AutoValue_IpAddress(
String id,
Integer addressFamily,
String netmask,
Boolean publicAddress,
Integer cidr,
Boolean management,
Boolean manageable,
Href assignedTo,
String network,
String address,
String gateway,
String href) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (addressFamily == null) {
throw new NullPointerException("Null addressFamily");
}
this.addressFamily = addressFamily;
if (netmask == null) {
throw new NullPointerException("Null netmask");
}
this.netmask = netmask;
if (publicAddress == null) {
throw new NullPointerException("Null publicAddress");
}
this.publicAddress = publicAddress;
if (cidr == null) {
throw new NullPointerException("Null cidr");
}
this.cidr = cidr;
if (management == null) {
throw new NullPointerException("Null management");
}
this.management = management;
if (manageable == null) {
throw new NullPointerException("Null manageable");
}
this.manageable = manageable;
if (assignedTo == null) {
throw new NullPointerException("Null assignedTo");
}
this.assignedTo = assignedTo;
if (network == null) {
throw new NullPointerException("Null network");
}
this.network = network;
if (address == null) {
throw new NullPointerException("Null address");
}
this.address = address;
if (gateway == null) {
throw new NullPointerException("Null gateway");
}
this.gateway = gateway;
if (href == null) {
throw new NullPointerException("Null href");
}
this.href = href;
}
@Override
public String id() {
return id;
}
@Override
public Integer addressFamily() {
return addressFamily;
}
@Override
public String netmask() {
return netmask;
}
@Override
public Boolean publicAddress() {
return publicAddress;
}
@Override
public Integer cidr() {
return cidr;
}
@Override
public Boolean management() {
return management;
}
@Override
public Boolean manageable() {
return manageable;
}
@Override
public Href assignedTo() {
return assignedTo;
}
@Override
public String network() {
return network;
}
@Override
public String address() {
return address;
}
@Override
public String gateway() {
return gateway;
}
@Override
public String href() {
return href;
}
@Override
public String toString() {
return "IpAddress{"
+ "id=" + id + ", "
+ "addressFamily=" + addressFamily + ", "
+ "netmask=" + netmask + ", "
+ "publicAddress=" + publicAddress + ", "
+ "cidr=" + cidr + ", "
+ "management=" + management + ", "
+ "manageable=" + manageable + ", "
+ "assignedTo=" + assignedTo + ", "
+ "network=" + network + ", "
+ "address=" + address + ", "
+ "gateway=" + gateway + ", "
+ "href=" + href
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof IpAddress) {
IpAddress that = (IpAddress) o;
return (this.id.equals(that.id()))
&& (this.addressFamily.equals(that.addressFamily()))
&& (this.netmask.equals(that.netmask()))
&& (this.publicAddress.equals(that.publicAddress()))
&& (this.cidr.equals(that.cidr()))
&& (this.management.equals(that.management()))
&& (this.manageable.equals(that.manageable()))
&& (this.assignedTo.equals(that.assignedTo()))
&& (this.network.equals(that.network()))
&& (this.address.equals(that.address()))
&& (this.gateway.equals(that.gateway()))
&& (this.href.equals(that.href()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.addressFamily.hashCode();
h *= 1000003;
h ^= this.netmask.hashCode();
h *= 1000003;
h ^= this.publicAddress.hashCode();
h *= 1000003;
h ^= this.cidr.hashCode();
h *= 1000003;
h ^= this.management.hashCode();
h *= 1000003;
h ^= this.manageable.hashCode();
h *= 1000003;
h ^= this.assignedTo.hashCode();
h *= 1000003;
h ^= this.network.hashCode();
h *= 1000003;
h ^= this.address.hashCode();
h *= 1000003;
h ^= this.gateway.hashCode();
h *= 1000003;
h ^= this.href.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy