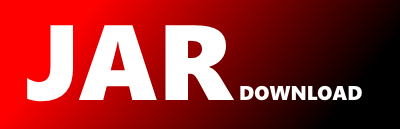
org.jclouds.packet.domain.AutoValue_Project Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.packet.domain;
import java.util.Date;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Project extends Project {
private final String id;
private final String name;
private final Date createdAt;
private final Date updatedAt;
private final Map maxDevices;
private final List members;
private final List memberships;
private final List invitations;
private final Href paymentMethod;
private final List devices;
private final List sshKeys;
private final List volumes;
private final String href;
AutoValue_Project(
String id,
String name,
Date createdAt,
Date updatedAt,
Map maxDevices,
List members,
List memberships,
List invitations,
Href paymentMethod,
List devices,
List sshKeys,
List volumes,
String href) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
if (updatedAt == null) {
throw new NullPointerException("Null updatedAt");
}
this.updatedAt = updatedAt;
if (maxDevices == null) {
throw new NullPointerException("Null maxDevices");
}
this.maxDevices = maxDevices;
if (members == null) {
throw new NullPointerException("Null members");
}
this.members = members;
if (memberships == null) {
throw new NullPointerException("Null memberships");
}
this.memberships = memberships;
if (invitations == null) {
throw new NullPointerException("Null invitations");
}
this.invitations = invitations;
if (paymentMethod == null) {
throw new NullPointerException("Null paymentMethod");
}
this.paymentMethod = paymentMethod;
if (devices == null) {
throw new NullPointerException("Null devices");
}
this.devices = devices;
if (sshKeys == null) {
throw new NullPointerException("Null sshKeys");
}
this.sshKeys = sshKeys;
if (volumes == null) {
throw new NullPointerException("Null volumes");
}
this.volumes = volumes;
if (href == null) {
throw new NullPointerException("Null href");
}
this.href = href;
}
@Override
public String id() {
return id;
}
@Override
public String name() {
return name;
}
@Override
public Date createdAt() {
return createdAt;
}
@Override
public Date updatedAt() {
return updatedAt;
}
@Override
public Map maxDevices() {
return maxDevices;
}
@Override
public List members() {
return members;
}
@Override
public List memberships() {
return memberships;
}
@Override
public List invitations() {
return invitations;
}
@Override
public Href paymentMethod() {
return paymentMethod;
}
@Override
public List devices() {
return devices;
}
@Override
public List sshKeys() {
return sshKeys;
}
@Override
public List volumes() {
return volumes;
}
@Override
public String href() {
return href;
}
@Override
public String toString() {
return "Project{"
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "createdAt=" + createdAt + ", "
+ "updatedAt=" + updatedAt + ", "
+ "maxDevices=" + maxDevices + ", "
+ "members=" + members + ", "
+ "memberships=" + memberships + ", "
+ "invitations=" + invitations + ", "
+ "paymentMethod=" + paymentMethod + ", "
+ "devices=" + devices + ", "
+ "sshKeys=" + sshKeys + ", "
+ "volumes=" + volumes + ", "
+ "href=" + href
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Project) {
Project that = (Project) o;
return (this.id.equals(that.id()))
&& (this.name.equals(that.name()))
&& (this.createdAt.equals(that.createdAt()))
&& (this.updatedAt.equals(that.updatedAt()))
&& (this.maxDevices.equals(that.maxDevices()))
&& (this.members.equals(that.members()))
&& (this.memberships.equals(that.memberships()))
&& (this.invitations.equals(that.invitations()))
&& (this.paymentMethod.equals(that.paymentMethod()))
&& (this.devices.equals(that.devices()))
&& (this.sshKeys.equals(that.sshKeys()))
&& (this.volumes.equals(that.volumes()))
&& (this.href.equals(that.href()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.name.hashCode();
h *= 1000003;
h ^= this.createdAt.hashCode();
h *= 1000003;
h ^= this.updatedAt.hashCode();
h *= 1000003;
h ^= this.maxDevices.hashCode();
h *= 1000003;
h ^= this.members.hashCode();
h *= 1000003;
h ^= this.memberships.hashCode();
h *= 1000003;
h ^= this.invitations.hashCode();
h *= 1000003;
h ^= this.paymentMethod.hashCode();
h *= 1000003;
h ^= this.devices.hashCode();
h *= 1000003;
h ^= this.sshKeys.hashCode();
h *= 1000003;
h ^= this.volumes.hashCode();
h *= 1000003;
h ^= this.href.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy