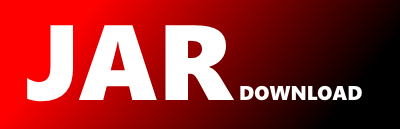
org.jclouds.packet.domain.AutoValue_SshKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.packet.domain;
import java.util.Date;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_SshKey extends SshKey {
private final String id;
private final String label;
private final String key;
private final String fingerprint;
private final Date createdAt;
private final Date updatedAt;
private final SshKey.Owner owner;
private final String href;
AutoValue_SshKey(
String id,
String label,
String key,
String fingerprint,
Date createdAt,
Date updatedAt,
@Nullable SshKey.Owner owner,
String href) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
if (label == null) {
throw new NullPointerException("Null label");
}
this.label = label;
if (key == null) {
throw new NullPointerException("Null key");
}
this.key = key;
if (fingerprint == null) {
throw new NullPointerException("Null fingerprint");
}
this.fingerprint = fingerprint;
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
if (updatedAt == null) {
throw new NullPointerException("Null updatedAt");
}
this.updatedAt = updatedAt;
this.owner = owner;
if (href == null) {
throw new NullPointerException("Null href");
}
this.href = href;
}
@Override
public String id() {
return id;
}
@Override
public String label() {
return label;
}
@Override
public String key() {
return key;
}
@Override
public String fingerprint() {
return fingerprint;
}
@Override
public Date createdAt() {
return createdAt;
}
@Override
public Date updatedAt() {
return updatedAt;
}
@Nullable
@Override
public SshKey.Owner owner() {
return owner;
}
@Override
public String href() {
return href;
}
@Override
public String toString() {
return "SshKey{"
+ "id=" + id + ", "
+ "label=" + label + ", "
+ "key=" + key + ", "
+ "fingerprint=" + fingerprint + ", "
+ "createdAt=" + createdAt + ", "
+ "updatedAt=" + updatedAt + ", "
+ "owner=" + owner + ", "
+ "href=" + href
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof SshKey) {
SshKey that = (SshKey) o;
return (this.id.equals(that.id()))
&& (this.label.equals(that.label()))
&& (this.key.equals(that.key()))
&& (this.fingerprint.equals(that.fingerprint()))
&& (this.createdAt.equals(that.createdAt()))
&& (this.updatedAt.equals(that.updatedAt()))
&& ((this.owner == null) ? (that.owner() == null) : this.owner.equals(that.owner()))
&& (this.href.equals(that.href()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= this.label.hashCode();
h *= 1000003;
h ^= this.key.hashCode();
h *= 1000003;
h ^= this.fingerprint.hashCode();
h *= 1000003;
h ^= this.createdAt.hashCode();
h *= 1000003;
h ^= this.updatedAt.hashCode();
h *= 1000003;
h ^= (owner == null) ? 0 : this.owner.hashCode();
h *= 1000003;
h ^= this.href.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy