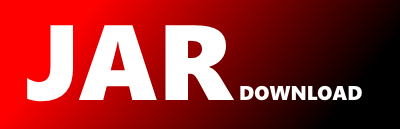
org.jclouds.profitbricks.domain.AutoValue_Nic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.profitbricks.domain;
import java.util.List;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Nic extends Nic {
private final String id;
private final String name;
private final String dataCenterId;
private final Integer lanId;
private final Boolean internetAccess;
private final String serverId;
private final List ips;
private final String macAddress;
private final Firewall firewall;
private final Boolean dhcpActive;
private final String gatewayIp;
private final ProvisioningState state;
private AutoValue_Nic(
@Nullable String id,
@Nullable String name,
@Nullable String dataCenterId,
@Nullable Integer lanId,
@Nullable Boolean internetAccess,
@Nullable String serverId,
@Nullable List ips,
@Nullable String macAddress,
@Nullable Firewall firewall,
@Nullable Boolean dhcpActive,
@Nullable String gatewayIp,
@Nullable ProvisioningState state) {
this.id = id;
this.name = name;
this.dataCenterId = dataCenterId;
this.lanId = lanId;
this.internetAccess = internetAccess;
this.serverId = serverId;
this.ips = ips;
this.macAddress = macAddress;
this.firewall = firewall;
this.dhcpActive = dhcpActive;
this.gatewayIp = gatewayIp;
this.state = state;
}
@Nullable
@Override
public String id() {
return id;
}
@Nullable
@Override
public String name() {
return name;
}
@Nullable
@Override
public String dataCenterId() {
return dataCenterId;
}
@Nullable
@Override
public Integer lanId() {
return lanId;
}
@Nullable
@Override
public Boolean internetAccess() {
return internetAccess;
}
@Nullable
@Override
public String serverId() {
return serverId;
}
@Nullable
@Override
public List ips() {
return ips;
}
@Nullable
@Override
public String macAddress() {
return macAddress;
}
@Nullable
@Override
public Firewall firewall() {
return firewall;
}
@Nullable
@Override
public Boolean dhcpActive() {
return dhcpActive;
}
@Nullable
@Override
public String gatewayIp() {
return gatewayIp;
}
@Nullable
@Override
public ProvisioningState state() {
return state;
}
@Override
public String toString() {
return "Nic{"
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "dataCenterId=" + dataCenterId + ", "
+ "lanId=" + lanId + ", "
+ "internetAccess=" + internetAccess + ", "
+ "serverId=" + serverId + ", "
+ "ips=" + ips + ", "
+ "macAddress=" + macAddress + ", "
+ "firewall=" + firewall + ", "
+ "dhcpActive=" + dhcpActive + ", "
+ "gatewayIp=" + gatewayIp + ", "
+ "state=" + state
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Nic) {
Nic that = (Nic) o;
return ((this.id == null) ? (that.id() == null) : this.id.equals(that.id()))
&& ((this.name == null) ? (that.name() == null) : this.name.equals(that.name()))
&& ((this.dataCenterId == null) ? (that.dataCenterId() == null) : this.dataCenterId.equals(that.dataCenterId()))
&& ((this.lanId == null) ? (that.lanId() == null) : this.lanId.equals(that.lanId()))
&& ((this.internetAccess == null) ? (that.internetAccess() == null) : this.internetAccess.equals(that.internetAccess()))
&& ((this.serverId == null) ? (that.serverId() == null) : this.serverId.equals(that.serverId()))
&& ((this.ips == null) ? (that.ips() == null) : this.ips.equals(that.ips()))
&& ((this.macAddress == null) ? (that.macAddress() == null) : this.macAddress.equals(that.macAddress()))
&& ((this.firewall == null) ? (that.firewall() == null) : this.firewall.equals(that.firewall()))
&& ((this.dhcpActive == null) ? (that.dhcpActive() == null) : this.dhcpActive.equals(that.dhcpActive()))
&& ((this.gatewayIp == null) ? (that.gatewayIp() == null) : this.gatewayIp.equals(that.gatewayIp()))
&& ((this.state == null) ? (that.state() == null) : this.state.equals(that.state()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= (id == null) ? 0 : this.id.hashCode();
h *= 1000003;
h ^= (name == null) ? 0 : this.name.hashCode();
h *= 1000003;
h ^= (dataCenterId == null) ? 0 : this.dataCenterId.hashCode();
h *= 1000003;
h ^= (lanId == null) ? 0 : this.lanId.hashCode();
h *= 1000003;
h ^= (internetAccess == null) ? 0 : this.internetAccess.hashCode();
h *= 1000003;
h ^= (serverId == null) ? 0 : this.serverId.hashCode();
h *= 1000003;
h ^= (ips == null) ? 0 : this.ips.hashCode();
h *= 1000003;
h ^= (macAddress == null) ? 0 : this.macAddress.hashCode();
h *= 1000003;
h ^= (firewall == null) ? 0 : this.firewall.hashCode();
h *= 1000003;
h ^= (dhcpActive == null) ? 0 : this.dhcpActive.hashCode();
h *= 1000003;
h ^= (gatewayIp == null) ? 0 : this.gatewayIp.hashCode();
h *= 1000003;
h ^= (state == null) ? 0 : this.state.hashCode();
return h;
}
@Override
public Nic.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends Nic.Builder {
private String id;
private String name;
private String dataCenterId;
private Integer lanId;
private Boolean internetAccess;
private String serverId;
private List ips;
private String macAddress;
private Firewall firewall;
private Boolean dhcpActive;
private String gatewayIp;
private ProvisioningState state;
Builder() {
}
private Builder(Nic source) {
this.id = source.id();
this.name = source.name();
this.dataCenterId = source.dataCenterId();
this.lanId = source.lanId();
this.internetAccess = source.internetAccess();
this.serverId = source.serverId();
this.ips = source.ips();
this.macAddress = source.macAddress();
this.firewall = source.firewall();
this.dhcpActive = source.dhcpActive();
this.gatewayIp = source.gatewayIp();
this.state = source.state();
}
@Override
public Nic.Builder id(@Nullable String id) {
this.id = id;
return this;
}
@Override
public Nic.Builder name(@Nullable String name) {
this.name = name;
return this;
}
@Override
public Nic.Builder dataCenterId(@Nullable String dataCenterId) {
this.dataCenterId = dataCenterId;
return this;
}
@Override
public Nic.Builder lanId(@Nullable Integer lanId) {
this.lanId = lanId;
return this;
}
@Override
public Nic.Builder internetAccess(@Nullable Boolean internetAccess) {
this.internetAccess = internetAccess;
return this;
}
@Override
public Nic.Builder serverId(@Nullable String serverId) {
this.serverId = serverId;
return this;
}
@Override
public Nic.Builder ips(@Nullable List ips) {
this.ips = ips;
return this;
}
@Override
public Nic.Builder macAddress(@Nullable String macAddress) {
this.macAddress = macAddress;
return this;
}
@Override
public Nic.Builder firewall(@Nullable Firewall firewall) {
this.firewall = firewall;
return this;
}
@Override
public Nic.Builder dhcpActive(@Nullable Boolean dhcpActive) {
this.dhcpActive = dhcpActive;
return this;
}
@Override
public Nic.Builder gatewayIp(@Nullable String gatewayIp) {
this.gatewayIp = gatewayIp;
return this;
}
@Override
public Nic.Builder state(@Nullable ProvisioningState state) {
this.state = state;
return this;
}
@Override
Nic autoBuild() {
return new AutoValue_Nic(
this.id,
this.name,
this.dataCenterId,
this.lanId,
this.internetAccess,
this.serverId,
this.ips,
this.macAddress,
this.firewall,
this.dhcpActive,
this.gatewayIp,
this.state);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy