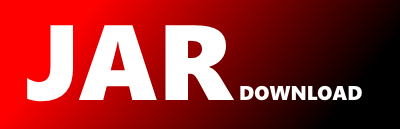
org.jclouds.profitbricks.domain.AutoValue_Server_Request_UpdatePayload Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.profitbricks.domain;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Server_Request_UpdatePayload extends Server.Request.UpdatePayload {
private final String id;
private final Integer cores;
private final Integer ram;
private final String name;
private final String bootFromStorageId;
private final String bootFromImageId;
private final AvailabilityZone availabilityZone;
private final OsType osType;
private final Boolean isCpuHotPlug;
private final Boolean isCpuHotUnPlug;
private final Boolean isRamHotPlug;
private final Boolean isRamHotUnPlug;
private final Boolean isNicHotPlug;
private final Boolean isNicHotUnPlug;
private final Boolean isDiscVirtioHotPlug;
private final Boolean isDiscVirtioHotUnPlug;
private AutoValue_Server_Request_UpdatePayload(
String id,
@Nullable Integer cores,
@Nullable Integer ram,
@Nullable String name,
@Nullable String bootFromStorageId,
@Nullable String bootFromImageId,
@Nullable AvailabilityZone availabilityZone,
@Nullable OsType osType,
@Nullable Boolean isCpuHotPlug,
@Nullable Boolean isCpuHotUnPlug,
@Nullable Boolean isRamHotPlug,
@Nullable Boolean isRamHotUnPlug,
@Nullable Boolean isNicHotPlug,
@Nullable Boolean isNicHotUnPlug,
@Nullable Boolean isDiscVirtioHotPlug,
@Nullable Boolean isDiscVirtioHotUnPlug) {
this.id = id;
this.cores = cores;
this.ram = ram;
this.name = name;
this.bootFromStorageId = bootFromStorageId;
this.bootFromImageId = bootFromImageId;
this.availabilityZone = availabilityZone;
this.osType = osType;
this.isCpuHotPlug = isCpuHotPlug;
this.isCpuHotUnPlug = isCpuHotUnPlug;
this.isRamHotPlug = isRamHotPlug;
this.isRamHotUnPlug = isRamHotUnPlug;
this.isNicHotPlug = isNicHotPlug;
this.isNicHotUnPlug = isNicHotUnPlug;
this.isDiscVirtioHotPlug = isDiscVirtioHotPlug;
this.isDiscVirtioHotUnPlug = isDiscVirtioHotUnPlug;
}
@Override
public String id() {
return id;
}
@Nullable
@Override
public Integer cores() {
return cores;
}
@Nullable
@Override
public Integer ram() {
return ram;
}
@Nullable
@Override
public String name() {
return name;
}
@Nullable
@Override
public String bootFromStorageId() {
return bootFromStorageId;
}
@Nullable
@Override
public String bootFromImageId() {
return bootFromImageId;
}
@Nullable
@Override
public AvailabilityZone availabilityZone() {
return availabilityZone;
}
@Nullable
@Override
public OsType osType() {
return osType;
}
@Nullable
@Override
public Boolean isCpuHotPlug() {
return isCpuHotPlug;
}
@Nullable
@Override
public Boolean isCpuHotUnPlug() {
return isCpuHotUnPlug;
}
@Nullable
@Override
public Boolean isRamHotPlug() {
return isRamHotPlug;
}
@Nullable
@Override
public Boolean isRamHotUnPlug() {
return isRamHotUnPlug;
}
@Nullable
@Override
public Boolean isNicHotPlug() {
return isNicHotPlug;
}
@Nullable
@Override
public Boolean isNicHotUnPlug() {
return isNicHotUnPlug;
}
@Nullable
@Override
public Boolean isDiscVirtioHotPlug() {
return isDiscVirtioHotPlug;
}
@Nullable
@Override
public Boolean isDiscVirtioHotUnPlug() {
return isDiscVirtioHotUnPlug;
}
@Override
public String toString() {
return "UpdatePayload{"
+ "id=" + id + ", "
+ "cores=" + cores + ", "
+ "ram=" + ram + ", "
+ "name=" + name + ", "
+ "bootFromStorageId=" + bootFromStorageId + ", "
+ "bootFromImageId=" + bootFromImageId + ", "
+ "availabilityZone=" + availabilityZone + ", "
+ "osType=" + osType + ", "
+ "isCpuHotPlug=" + isCpuHotPlug + ", "
+ "isCpuHotUnPlug=" + isCpuHotUnPlug + ", "
+ "isRamHotPlug=" + isRamHotPlug + ", "
+ "isRamHotUnPlug=" + isRamHotUnPlug + ", "
+ "isNicHotPlug=" + isNicHotPlug + ", "
+ "isNicHotUnPlug=" + isNicHotUnPlug + ", "
+ "isDiscVirtioHotPlug=" + isDiscVirtioHotPlug + ", "
+ "isDiscVirtioHotUnPlug=" + isDiscVirtioHotUnPlug
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Server.Request.UpdatePayload) {
Server.Request.UpdatePayload that = (Server.Request.UpdatePayload) o;
return (this.id.equals(that.id()))
&& ((this.cores == null) ? (that.cores() == null) : this.cores.equals(that.cores()))
&& ((this.ram == null) ? (that.ram() == null) : this.ram.equals(that.ram()))
&& ((this.name == null) ? (that.name() == null) : this.name.equals(that.name()))
&& ((this.bootFromStorageId == null) ? (that.bootFromStorageId() == null) : this.bootFromStorageId.equals(that.bootFromStorageId()))
&& ((this.bootFromImageId == null) ? (that.bootFromImageId() == null) : this.bootFromImageId.equals(that.bootFromImageId()))
&& ((this.availabilityZone == null) ? (that.availabilityZone() == null) : this.availabilityZone.equals(that.availabilityZone()))
&& ((this.osType == null) ? (that.osType() == null) : this.osType.equals(that.osType()))
&& ((this.isCpuHotPlug == null) ? (that.isCpuHotPlug() == null) : this.isCpuHotPlug.equals(that.isCpuHotPlug()))
&& ((this.isCpuHotUnPlug == null) ? (that.isCpuHotUnPlug() == null) : this.isCpuHotUnPlug.equals(that.isCpuHotUnPlug()))
&& ((this.isRamHotPlug == null) ? (that.isRamHotPlug() == null) : this.isRamHotPlug.equals(that.isRamHotPlug()))
&& ((this.isRamHotUnPlug == null) ? (that.isRamHotUnPlug() == null) : this.isRamHotUnPlug.equals(that.isRamHotUnPlug()))
&& ((this.isNicHotPlug == null) ? (that.isNicHotPlug() == null) : this.isNicHotPlug.equals(that.isNicHotPlug()))
&& ((this.isNicHotUnPlug == null) ? (that.isNicHotUnPlug() == null) : this.isNicHotUnPlug.equals(that.isNicHotUnPlug()))
&& ((this.isDiscVirtioHotPlug == null) ? (that.isDiscVirtioHotPlug() == null) : this.isDiscVirtioHotPlug.equals(that.isDiscVirtioHotPlug()))
&& ((this.isDiscVirtioHotUnPlug == null) ? (that.isDiscVirtioHotUnPlug() == null) : this.isDiscVirtioHotUnPlug.equals(that.isDiscVirtioHotUnPlug()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.id.hashCode();
h *= 1000003;
h ^= (cores == null) ? 0 : this.cores.hashCode();
h *= 1000003;
h ^= (ram == null) ? 0 : this.ram.hashCode();
h *= 1000003;
h ^= (name == null) ? 0 : this.name.hashCode();
h *= 1000003;
h ^= (bootFromStorageId == null) ? 0 : this.bootFromStorageId.hashCode();
h *= 1000003;
h ^= (bootFromImageId == null) ? 0 : this.bootFromImageId.hashCode();
h *= 1000003;
h ^= (availabilityZone == null) ? 0 : this.availabilityZone.hashCode();
h *= 1000003;
h ^= (osType == null) ? 0 : this.osType.hashCode();
h *= 1000003;
h ^= (isCpuHotPlug == null) ? 0 : this.isCpuHotPlug.hashCode();
h *= 1000003;
h ^= (isCpuHotUnPlug == null) ? 0 : this.isCpuHotUnPlug.hashCode();
h *= 1000003;
h ^= (isRamHotPlug == null) ? 0 : this.isRamHotPlug.hashCode();
h *= 1000003;
h ^= (isRamHotUnPlug == null) ? 0 : this.isRamHotUnPlug.hashCode();
h *= 1000003;
h ^= (isNicHotPlug == null) ? 0 : this.isNicHotPlug.hashCode();
h *= 1000003;
h ^= (isNicHotUnPlug == null) ? 0 : this.isNicHotUnPlug.hashCode();
h *= 1000003;
h ^= (isDiscVirtioHotPlug == null) ? 0 : this.isDiscVirtioHotPlug.hashCode();
h *= 1000003;
h ^= (isDiscVirtioHotUnPlug == null) ? 0 : this.isDiscVirtioHotUnPlug.hashCode();
return h;
}
static final class Builder extends Server.Request.UpdatePayload.Builder {
private String id;
private Integer cores;
private Integer ram;
private String name;
private String bootFromStorageId;
private String bootFromImageId;
private AvailabilityZone availabilityZone;
private OsType osType;
private Boolean isCpuHotPlug;
private Boolean isCpuHotUnPlug;
private Boolean isRamHotPlug;
private Boolean isRamHotUnPlug;
private Boolean isNicHotPlug;
private Boolean isNicHotUnPlug;
private Boolean isDiscVirtioHotPlug;
private Boolean isDiscVirtioHotUnPlug;
Builder() {
}
@Override
public Server.Request.UpdatePayload.Builder id(String id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder cores(@Nullable Integer cores) {
this.cores = cores;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder ram(@Nullable Integer ram) {
this.ram = ram;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder name(@Nullable String name) {
this.name = name;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder bootFromStorageId(@Nullable String bootFromStorageId) {
this.bootFromStorageId = bootFromStorageId;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder bootFromImageId(@Nullable String bootFromImageId) {
this.bootFromImageId = bootFromImageId;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder availabilityZone(@Nullable AvailabilityZone availabilityZone) {
this.availabilityZone = availabilityZone;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder osType(@Nullable OsType osType) {
this.osType = osType;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder isCpuHotPlug(@Nullable Boolean isCpuHotPlug) {
this.isCpuHotPlug = isCpuHotPlug;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder isCpuHotUnPlug(@Nullable Boolean isCpuHotUnPlug) {
this.isCpuHotUnPlug = isCpuHotUnPlug;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder isRamHotPlug(@Nullable Boolean isRamHotPlug) {
this.isRamHotPlug = isRamHotPlug;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder isRamHotUnPlug(@Nullable Boolean isRamHotUnPlug) {
this.isRamHotUnPlug = isRamHotUnPlug;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder isNicHotPlug(@Nullable Boolean isNicHotPlug) {
this.isNicHotPlug = isNicHotPlug;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder isNicHotUnPlug(@Nullable Boolean isNicHotUnPlug) {
this.isNicHotUnPlug = isNicHotUnPlug;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder isDiscVirtioHotPlug(@Nullable Boolean isDiscVirtioHotPlug) {
this.isDiscVirtioHotPlug = isDiscVirtioHotPlug;
return this;
}
@Override
public Server.Request.UpdatePayload.Builder isDiscVirtioHotUnPlug(@Nullable Boolean isDiscVirtioHotUnPlug) {
this.isDiscVirtioHotUnPlug = isDiscVirtioHotUnPlug;
return this;
}
@Override
Server.Request.UpdatePayload autoBuild() {
String missing = "";
if (this.id == null) {
missing += " id";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Server_Request_UpdatePayload(
this.id,
this.cores,
this.ram,
this.name,
this.bootFromStorageId,
this.bootFromImageId,
this.availabilityZone,
this.osType,
this.isCpuHotPlug,
this.isCpuHotUnPlug,
this.isRamHotPlug,
this.isRamHotUnPlug,
this.isNicHotPlug,
this.isNicHotUnPlug,
this.isDiscVirtioHotPlug,
this.isDiscVirtioHotUnPlug);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy