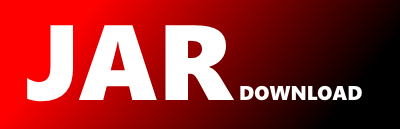
org.jclouds.softlayer.domain.AutoValue_Subnet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-shaded Show documentation
Show all versions of jclouds-shaded Show documentation
Provides a shaded jclouds with relocated guava and guice
The newest version!
package org.jclouds.softlayer.domain;
import java.util.Date;
import javax.annotation.Generated;
import org.jclouds.javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Subnet extends Subnet {
private final String boradcastAddress;
private final int cidr;
private final String gateway;
private final long id;
private final boolean isCustomerOwned;
private final boolean isCustomerRoutable;
private final Date modifyDate;
private final String netmask;
private final String networkIdentifier;
private final long networkVlanId;
private final String note;
private final String sortOrder;
private final String subnetType;
private final String totalIpAddresses;
private final String usableIpAddressCount;
private final int version;
private final String addressSpace;
AutoValue_Subnet(
String boradcastAddress,
int cidr,
String gateway,
long id,
boolean isCustomerOwned,
boolean isCustomerRoutable,
@Nullable Date modifyDate,
String netmask,
String networkIdentifier,
long networkVlanId,
@Nullable String note,
String sortOrder,
@Nullable String subnetType,
String totalIpAddresses,
String usableIpAddressCount,
int version,
@Nullable String addressSpace) {
if (boradcastAddress == null) {
throw new NullPointerException("Null boradcastAddress");
}
this.boradcastAddress = boradcastAddress;
this.cidr = cidr;
if (gateway == null) {
throw new NullPointerException("Null gateway");
}
this.gateway = gateway;
this.id = id;
this.isCustomerOwned = isCustomerOwned;
this.isCustomerRoutable = isCustomerRoutable;
this.modifyDate = modifyDate;
if (netmask == null) {
throw new NullPointerException("Null netmask");
}
this.netmask = netmask;
if (networkIdentifier == null) {
throw new NullPointerException("Null networkIdentifier");
}
this.networkIdentifier = networkIdentifier;
this.networkVlanId = networkVlanId;
this.note = note;
if (sortOrder == null) {
throw new NullPointerException("Null sortOrder");
}
this.sortOrder = sortOrder;
this.subnetType = subnetType;
if (totalIpAddresses == null) {
throw new NullPointerException("Null totalIpAddresses");
}
this.totalIpAddresses = totalIpAddresses;
if (usableIpAddressCount == null) {
throw new NullPointerException("Null usableIpAddressCount");
}
this.usableIpAddressCount = usableIpAddressCount;
this.version = version;
this.addressSpace = addressSpace;
}
@Override
public String boradcastAddress() {
return boradcastAddress;
}
@Override
public int cidr() {
return cidr;
}
@Override
public String gateway() {
return gateway;
}
@Override
public long id() {
return id;
}
@Override
public boolean isCustomerOwned() {
return isCustomerOwned;
}
@Override
public boolean isCustomerRoutable() {
return isCustomerRoutable;
}
@Nullable
@Override
public Date modifyDate() {
return modifyDate;
}
@Override
public String netmask() {
return netmask;
}
@Override
public String networkIdentifier() {
return networkIdentifier;
}
@Override
public long networkVlanId() {
return networkVlanId;
}
@Nullable
@Override
public String note() {
return note;
}
@Override
public String sortOrder() {
return sortOrder;
}
@Nullable
@Override
public String subnetType() {
return subnetType;
}
@Override
public String totalIpAddresses() {
return totalIpAddresses;
}
@Override
public String usableIpAddressCount() {
return usableIpAddressCount;
}
@Override
public int version() {
return version;
}
@Nullable
@Override
public String addressSpace() {
return addressSpace;
}
@Override
public String toString() {
return "Subnet{"
+ "boradcastAddress=" + boradcastAddress + ", "
+ "cidr=" + cidr + ", "
+ "gateway=" + gateway + ", "
+ "id=" + id + ", "
+ "isCustomerOwned=" + isCustomerOwned + ", "
+ "isCustomerRoutable=" + isCustomerRoutable + ", "
+ "modifyDate=" + modifyDate + ", "
+ "netmask=" + netmask + ", "
+ "networkIdentifier=" + networkIdentifier + ", "
+ "networkVlanId=" + networkVlanId + ", "
+ "note=" + note + ", "
+ "sortOrder=" + sortOrder + ", "
+ "subnetType=" + subnetType + ", "
+ "totalIpAddresses=" + totalIpAddresses + ", "
+ "usableIpAddressCount=" + usableIpAddressCount + ", "
+ "version=" + version + ", "
+ "addressSpace=" + addressSpace
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Subnet) {
Subnet that = (Subnet) o;
return (this.boradcastAddress.equals(that.boradcastAddress()))
&& (this.cidr == that.cidr())
&& (this.gateway.equals(that.gateway()))
&& (this.id == that.id())
&& (this.isCustomerOwned == that.isCustomerOwned())
&& (this.isCustomerRoutable == that.isCustomerRoutable())
&& ((this.modifyDate == null) ? (that.modifyDate() == null) : this.modifyDate.equals(that.modifyDate()))
&& (this.netmask.equals(that.netmask()))
&& (this.networkIdentifier.equals(that.networkIdentifier()))
&& (this.networkVlanId == that.networkVlanId())
&& ((this.note == null) ? (that.note() == null) : this.note.equals(that.note()))
&& (this.sortOrder.equals(that.sortOrder()))
&& ((this.subnetType == null) ? (that.subnetType() == null) : this.subnetType.equals(that.subnetType()))
&& (this.totalIpAddresses.equals(that.totalIpAddresses()))
&& (this.usableIpAddressCount.equals(that.usableIpAddressCount()))
&& (this.version == that.version())
&& ((this.addressSpace == null) ? (that.addressSpace() == null) : this.addressSpace.equals(that.addressSpace()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.boradcastAddress.hashCode();
h *= 1000003;
h ^= this.cidr;
h *= 1000003;
h ^= this.gateway.hashCode();
h *= 1000003;
h ^= (this.id >>> 32) ^ this.id;
h *= 1000003;
h ^= this.isCustomerOwned ? 1231 : 1237;
h *= 1000003;
h ^= this.isCustomerRoutable ? 1231 : 1237;
h *= 1000003;
h ^= (modifyDate == null) ? 0 : this.modifyDate.hashCode();
h *= 1000003;
h ^= this.netmask.hashCode();
h *= 1000003;
h ^= this.networkIdentifier.hashCode();
h *= 1000003;
h ^= (this.networkVlanId >>> 32) ^ this.networkVlanId;
h *= 1000003;
h ^= (note == null) ? 0 : this.note.hashCode();
h *= 1000003;
h ^= this.sortOrder.hashCode();
h *= 1000003;
h ^= (subnetType == null) ? 0 : this.subnetType.hashCode();
h *= 1000003;
h ^= this.totalIpAddresses.hashCode();
h *= 1000003;
h ^= this.usableIpAddressCount.hashCode();
h *= 1000003;
h ^= this.version;
h *= 1000003;
h ^= (addressSpace == null) ? 0 : this.addressSpace.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy