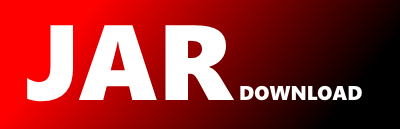
com.github.ferstl.maven.pomenforcers.PedanticDependencyConfigurationEnforcer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pedantic-pom-enforcers Show documentation
Show all versions of pedantic-pom-enforcers Show documentation
The Pedantic POM Enforcers consist of serveral Maven enforcer rules that help you keep your
project setup consistent and organized.
/*
* Copyright (c) 2013 by Stefan Ferstl
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.ferstl.maven.pomenforcers;
import java.util.Collection;
import java.util.List;
import com.github.ferstl.maven.pomenforcers.model.DependencyModel;
import com.google.common.base.Predicate;
import com.google.common.collect.Collections2;
import static com.github.ferstl.maven.pomenforcers.ErrorReport.toList;
/**
* This enforcer makes sure that dependency versions and exclusions are declared in the
* <dependencyManagement>
section.
*
*
* ### Example
* <rules>
* <dependencConfiguration implementation="com.github.ferstl.maven.pomenforcers.PedanticDependencyConfigurationEnforcer">
* <!-- Manage dependency versions in dependency management -->
* <manageVersions>true</manageVersions>
* <!-- allow ${project.version} outside dependency management -->
* <allowUnmanagedProjectVersions>true</allowUnmanagedProjectVersions>
* <!-- all dependency exclusions must be defined in dependency managment -->
* <manageExclusions>true</manageExclusions>
* </dependencyConfiguration>
* </rules>
*
*
* @id {@link PedanticEnforcerRule#DEPENDENCY_ORDER}
*/
public class PedanticDependencyConfigurationEnforcer extends AbstractPedanticEnforcer {
/** If enabled, dependency versions have to be declared in <dependencyManagement>
. */
private boolean manageVersions = true;
/** Allow ${project.version}
or ${version}
as dependency version. */
private boolean allowUnmangedProjectVersions = true;
/** If enabled, dependency exclusions have to be declared in <dependencyManagement>
. */
private boolean manageExclusions = true;
/**
* If set to true
, all dependency versions have to be defined in the dependency management.
* @param manageVersions Manage dependency versions in the dependency management.
* @configParam
* @default true
*/
public void setManageVersions(boolean manageVersions) {
this.manageVersions = manageVersions;
}
/**
* If set to true
, ${project.version}
may be used within
* the dependencies section.
* @param allowUnmangedProjectVersions Allow project versions outside of the dependencies section.
* @configParam
* @default true
*/
public void setAllowUnmanagedProjectVersions(boolean allowUnmangedProjectVersions) {
this.allowUnmangedProjectVersions = allowUnmangedProjectVersions;
}
/**
* If set to true
, all dependency exclusions must be declared in the dependency management.
* @param manageExclusions Manage exclusion in dependency management.
* @configParam
* @default true
*/
public void setManageExclusions(boolean manageExclusions) {
this.manageExclusions = manageExclusions;
}
@Override
protected PedanticEnforcerRule getDescription() {
return PedanticEnforcerRule.DEPENDENCY_CONFIGURATION;
}
@Override
protected void accept(PedanticEnforcerVisitor visitor) {
visitor.visit(this);
}
@Override
protected void doEnforce(ErrorReport report) {
if (this.manageVersions) {
enforceManagedVersions(report);
}
if (this.manageExclusions) {
enforceManagedExclusion(report);
}
}
private void enforceManagedVersions(ErrorReport report) {
Collection versionedDependencies = searchForDependencies(DependencyPredicate.WITH_VERSION);
// Filter all project versions if allowed
if (this.allowUnmangedProjectVersions) {
versionedDependencies = Collections2.filter(versionedDependencies, DependencyPredicate.WITH_PROJECT_VERSION);
}
if (!versionedDependencies.isEmpty()) {
report.addLine("Dependency versions have to be declared in :")
.addLine(toList(versionedDependencies));
}
}
private void enforceManagedExclusion(ErrorReport report) {
Collection depsWithExclusions = searchForDependencies(DependencyPredicate.WITH_EXCLUSION);
if (!depsWithExclusions.isEmpty()) {
report.addLine("Dependency exclusions have to be declared in :")
.addLine(toList(depsWithExclusions));
}
}
private Collection searchForDependencies(Predicate predicate) {
List dependencies = getProjectModel().getDependencies();
return Collections2.filter(dependencies, predicate);
}
private static enum DependencyPredicate implements Predicate {
WITH_VERSION {
@Override
public boolean apply(DependencyModel input) {
return input.getVersion() != null;
}
},
WITH_PROJECT_VERSION {
@Override
public boolean apply(DependencyModel input) {
return !"${project.version}".equals(input.getVersion())
&& !"${version}".equals(input.getVersion());
}
},
WITH_EXCLUSION {
@Override
public boolean apply(DependencyModel input) {
return !input.getExclusions().isEmpty();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy