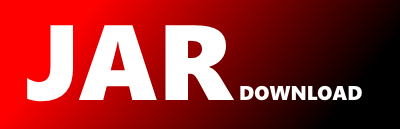
com.github.ferstl.maven.pomenforcers.CompoundPedanticEnforcer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pedantic-pom-enforcers Show documentation
Show all versions of pedantic-pom-enforcers Show documentation
The Pedantic POM Enforcers consist of serveral Maven enforcer rules that help you keep your
project setup consistent and organized.
/*
* Copyright (c) 2012 - 2015 by Stefan Ferstl
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.ferstl.maven.pomenforcers;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import com.github.ferstl.maven.pomenforcers.util.CommaSeparatorUtils;
import com.google.common.base.Strings;
import com.google.common.collect.Sets;
import static com.github.ferstl.maven.pomenforcers.PedanticEnforcerRule.stringToEnforcerRule;
/**
* The compound enforcer aggregates any combination of the available pedantic
* enforcer rules. Besides that it is easier to configure than the single rules,
* it is also more efficient because it has to parse the POM file of each Maven
* module only once.
*
*
* ### Example
* <rules>
* <compound implementation="com.github.ferstl.maven.pomenforcers.CompoundPedanticEnforcer">
* <enforcers>POM_SECTION_ORDER,MODULE_ORDER,DEPENDENCY_MANAGEMENT_ORDER,DEPENDENCY_ORDER,DEPENDENCY_CONFIGURATION,DEPENDENCY_SCOPE,DEPENDENCY_MANAGEMENT_LOCATION,PLUGIN_MANAGEMENT_ORDER,PLUGIN_CONFIGURATION,PLUGIN_MANAGEMENT_LOCATION</enforcers>
*
* <!-- POM_SECTION configuration -->
* <pomSectionPriorities>groupId,artifactId,version,packaging</pomSectionPriorities>
*
* <!-- MODULE_ORDER configuration -->
* <moduleOrderIgnores>>dist-deb,dist-rpm</moduleOrderIgnores>
*
* <!-- DEPENDENCY_ORDER configuration -->
* <dependenciesOrderBy>scope,groupId,artifactId</dependenciesOrderBy>
* <dependenciesScopePriorities>compile,runtime,provided</dependenciesScopePriorities>
* <dependenciesGroupIdPriorities>com.myproject,com.mylibs</dependenciesGroupIdPriorities>
* <dependenciesArtifactIdPriorities>commons-,utils-</dependenciesArtifactIdPriorities>
*
* <!-- DEPENDENCY_MANAGEMENT_ORDER configuration -->
* <dependencyManagementOrderBy>scope,groupId,artifactId</dependencyManagementOrderBy>
* <dependencyManagementScopePriorities>compile,runtime,provided</dependencyManagementScopePriorities>
* <dependencyManagementGroupIdPriorities>com.myproject,com.mylibs</dependencyManagementGroupIdPriorities>
* <dependencyManagementArtifactIdPriorities>commons-,utils-</dependencyManagementArtifactIdPriorities>
*
* <!-- DEPENDENCY_CONFIGURATION configuration -->
* <manageDependencyVersions>true</manageDependencyVersions>
* <allowUnmangedProjectVersions>true</allowUnmangedProjectVersions>
* <manageDependencyExclusions>true</manageDependencyExclusions>
*
* <!-- DEPENDENCY_SCOPE configuration -->
* <compileDependencies>com.example:mylib1,com.example:mylib2</compileDependencies>
* <providedDependencies>javax.servlet:servlet-api</providedDependencies>
* <runtimeDependencies>com.example:myruntimelib</runtimeDependencies>
* <systemDependencies>com.sun:tools</systemDependencies>
* <testDependencies>org.junit:junit,org.hamcrest:hamcrest-library</testDependencies>
* <importDependencies>org.jboss:jboss-as-client</importDependencies>
*
* <!-- DEPENDENCY_MANAGEMENT_LOCATION configuration -->
* <allowParentPomsForDependencyManagement>true</allowParentPomsForDependencyManagement>
* <dependencyManagingPoms>com.example.myproject:parent,com.example.myproject:subparent</dependencyManagingPoms>
*
* <!-- PLUGIN_MANAGEMENT_ORDER configuration -->
* <pluginManagementOrderBy>groupId,artifactId</pluginManagementOrderBy>
* <pluginManagementGroupIdPriorities>com.myproject.plugins,com.myproject.testplugins</pluginManagementGroupIdPriorities>
* <pluginManagementArtifactIdPriorities>mytest-,myintegrationtest-</pluginManagementArtifactIdPriorities>
*
* <!-- PLUGIN_CONFIGURATION configuration -->
* <managePluginVersions>true</managePluginVersions>
* <managePluginConfigurations>true</managePluginConfigurations>
* <managePluginDependencies>true</managePluginDependencies>
*
* <!-- PLUGIN_MANAGEMENT_LOCATION configuration -->
* <allowParentPomsForPluginManagement>true</allowParentPomsForPluginManagement>
* <pluginManagingPoms>com.myproject:parent-pom</pluginManagingPoms>
* </compound>
* </rules>
*
* @id n/a
* @since 1.0.0
*/
public class CompoundPedanticEnforcer extends AbstractPedanticEnforcer {
/**
* See {@link PedanticPomSectionOrderEnforcer#setSectionPriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String pomSectionPriorities;
/**
* See {@link PedanticModuleOrderEnforcer#setIgnoredModules(String)}.
* @configParam
* @since 1.0.0
*/
private String moduleOrderIgnores;
/**
* See {@link PedanticDependencyOrderEnforcer#setOrderBy(String)}.
* @configParam
* @since 1.0.0
*/
private String dependenciesOrderBy;
/**
* See {@link PedanticDependencyOrderEnforcer#setGroupIdPriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String dependenciesGroupIdPriorities;
/**
* See {@link PedanticDependencyOrderEnforcer#setArtifactIdPriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String dependenciesArtifactIdPriorities;
/**
* See {@link PedanticDependencyOrderEnforcer#setScopePriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String dependenciesScopePriorities;
/**
* See {@link PedanticDependencyManagementOrderEnforcer#setOrderBy(String)}.
* @configParam
* @since 1.0.0
*/
private String dependencyManagementOrderBy;
/**
* See
* {@link PedanticDependencyManagementOrderEnforcer#setGroupIdPriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String dependencyManagementGroupIdPriorities;
/**
* See
* {@link PedanticDependencyManagementOrderEnforcer#setArtifactIdPriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String dependencyManagementArtifactIdPriorities;
/**
* See
* {@link PedanticDependencyManagementOrderEnforcer#setScopePriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String dependencyManagementScopePriorities;
/**
* See {@link PedanticDependencyManagementLocationEnforcer#setAllowParentPoms(boolean)}.
* @configParam
* @since 1.2.0
*/
private Boolean allowParentPomsForDependencyManagement;
/**
* See {@link PedanticDependencyManagementLocationEnforcer#setDependencyManagingPoms(String)}.
* @configParam
* @since 1.0.0
*/
private String dependencyManagingPoms;
/**
* See
* {@link PedanticDependencyConfigurationEnforcer#setManageVersions(boolean)}.
* @configParam
* @since 1.0.0
*/
private Boolean manageDependencyVersions;
/**
* See
* {@link PedanticDependencyConfigurationEnforcer#setAllowUnmanagedProjectVersions(boolean)}.
* @configParam
* @since 1.0.0
*/
private Boolean allowUnmangedProjectVersions;
/**
* See
* {@link PedanticDependencyConfigurationEnforcer#setManageExclusions(boolean)}.
* @configParam
* @since 1.0.0
*/
private Boolean manageDependencyExclusions;
/**
* See {@link PedanticDependencyScopeEnforcer#setCompileDependencies(String)}.
* @configParam
* @since 1.0.0
*/
private String compileDependencies;
/**
* See {@link PedanticDependencyScopeEnforcer#setProvidedDependencies(String)}.
* @configParam
* @since 1.0.0
*/
private String providedDependencies;
/**
* See {@link PedanticDependencyScopeEnforcer#setRuntimeDependencies(String)}.
* @configParam
* @since 1.0.0
*/
private String runtimeDependencies;
/**
* See {@link PedanticDependencyScopeEnforcer#setSystemDependencies(String)}.
* @configParam
* @since 1.0.0
*/
private String systemDependencies;
/**
* See {@link PedanticDependencyScopeEnforcer#setTestDependencies(String)}.
* @configParam
* @since 1.0.0
*/
private String testDependencies;
/**
* See {@link PedanticDependencyScopeEnforcer#setImportDependencies(String)}.
* @configParam
* @since 1.0.0
*/
private String importDependencies;
/**
* See {@link PedanticPluginManagementOrderEnforcer#setOrderBy(String)}.
* @configParam
* @since 1.0.0
*/
private String pluginManagementOrderBy;
/**
* See
* {@link PedanticPluginManagementOrderEnforcer#setGroupIdPriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String pluginManagementGroupIdPriorities;
/**
* See
* {@link PedanticPluginManagementOrderEnforcer#setArtifactIdPriorities(String)}.
* @configParam
* @since 1.0.0
*/
private String pluginManagementArtifactIdPriorities;
/**
* See {@link PedanticPluginManagementLocationEnforcer#setAllowParentPoms(boolean)}.
* @configParam
* @since 1.2.0
*/
private Boolean allowParentPomsForPluginManagement;
/**
* See
* {@link PedanticPluginManagementLocationEnforcer#setPluginManagingPoms(String)}.
* @configParam
* @since 1.0.0
*/
private String pluginManagingPoms;
/**
* See {@link PedanticPluginConfigurationEnforcer#managePluginVersions}.
* @configParam
* @since 1.0.0
*/
private Boolean managePluginVersions;
/**
* See {@link PedanticPluginConfigurationEnforcer#managePluginConfigurations}
* @configParam
* @since 1.0.0
*/
private Boolean managePluginConfigurations;
/**
* See {@link PedanticPluginConfigurationEnforcer#managePluginDependencies}
* @configParam
* @since 1.0.0
*/
private Boolean managePluginDependencies;
/** Collection of enforcers to execute. */
private final Collection enforcers;
private final PropertyInitializationVisitor propertyInitializer;
public CompoundPedanticEnforcer() {
this.enforcers = Sets.newLinkedHashSet();
this.propertyInitializer = new PropertyInitializationVisitor();
}
public void setEnforcers(String enforcers) {
CommaSeparatorUtils.splitAndAddToCollection(enforcers, this.enforcers, stringToEnforcerRule());
}
@Override
protected PedanticEnforcerRule getDescription() {
return PedanticEnforcerRule.COMPOUND;
}
@Override
protected void accept(PedanticEnforcerVisitor visitor) {
visitor.visit(this);
}
@Override
protected void doEnforce(ErrorReport report) {
report.useLargeTitle();
List ruleErrors = new ArrayList<>();
for (PedanticEnforcerRule pedanticEnforcer : this.enforcers) {
AbstractPedanticEnforcer rule = pedanticEnforcer.createEnforcerRule();
rule.initialize(getHelper(), getPom(), getProjectModel());
rule.accept(this.propertyInitializer);
ErrorReport ruleReport = new ErrorReport(rule.getDescription());
rule.doEnforce(ruleReport);
if (ruleReport.hasErrors()) {
ruleErrors.add(ruleReport);
}
}
collectErrors(report, ruleErrors);
}
private void collectErrors(ErrorReport compundReport, List ruleErrors) {
if (!ruleErrors.isEmpty()) {
compundReport
.useLargeTitle()
.addLine("Please fix these problems:")
.emptyLine();
for (ErrorReport ruleError : ruleErrors) {
compundReport.addLine(ruleError.toString()).emptyLine().emptyLine();
}
}
}
private class PropertyInitializationVisitor implements PedanticEnforcerVisitor {
@Override
public void visit(PedanticPomSectionOrderEnforcer enforcer) {
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.pomSectionPriorities)) {
enforcer.setSectionPriorities(CompoundPedanticEnforcer.this.pomSectionPriorities);
}
}
@Override
public void visit(PedanticModuleOrderEnforcer enforcer) {
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.moduleOrderIgnores)) {
enforcer.setIgnoredModules(CompoundPedanticEnforcer.this.moduleOrderIgnores);
}
}
@Override
public void visit(PedanticDependencyManagementOrderEnforcer enforcer) {
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependencyManagementOrderBy)) {
enforcer.setOrderBy(CompoundPedanticEnforcer.this.dependencyManagementOrderBy);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependencyManagementGroupIdPriorities)) {
enforcer.setGroupIdPriorities(CompoundPedanticEnforcer.this.dependencyManagementGroupIdPriorities);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependencyManagementArtifactIdPriorities)) {
enforcer.setArtifactIdPriorities(CompoundPedanticEnforcer.this.dependencyManagementArtifactIdPriorities);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependencyManagementScopePriorities)) {
enforcer.setScopePriorities(CompoundPedanticEnforcer.this.dependencyManagementScopePriorities);
}
}
@Override
public void visit(PedanticDependencyManagementLocationEnforcer enforcer) {
if(CompoundPedanticEnforcer.this.allowParentPomsForDependencyManagement != null) {
enforcer.setAllowParentPoms(CompoundPedanticEnforcer.this.allowParentPomsForDependencyManagement);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependencyManagingPoms)) {
enforcer.setDependencyManagingPoms(CompoundPedanticEnforcer.this.dependencyManagingPoms);
}
}
@Override
public void visit(PedanticDependencyOrderEnforcer enforcer) {
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependenciesOrderBy)) {
enforcer.setOrderBy(CompoundPedanticEnforcer.this.dependenciesOrderBy);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependenciesGroupIdPriorities)) {
enforcer.setGroupIdPriorities(CompoundPedanticEnforcer.this.dependenciesGroupIdPriorities);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependenciesArtifactIdPriorities)) {
enforcer.setArtifactIdPriorities(CompoundPedanticEnforcer.this.dependenciesArtifactIdPriorities);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.dependenciesScopePriorities)) {
enforcer.setScopePriorities(CompoundPedanticEnforcer.this.dependenciesScopePriorities);
}
}
@Override
public void visit(PedanticDependencyConfigurationEnforcer dependencyConfigurationEnforcer) {
if (CompoundPedanticEnforcer.this.manageDependencyVersions != null) {
dependencyConfigurationEnforcer.setManageVersions(CompoundPedanticEnforcer.this.manageDependencyVersions);
}
if (CompoundPedanticEnforcer.this.allowUnmangedProjectVersions != null) {
dependencyConfigurationEnforcer.setAllowUnmanagedProjectVersions(
CompoundPedanticEnforcer.this.allowUnmangedProjectVersions);
}
if (CompoundPedanticEnforcer.this.manageDependencyExclusions != null) {
dependencyConfigurationEnforcer.setManageExclusions(CompoundPedanticEnforcer.this.manageDependencyExclusions);
}
}
@Override
public void visit(PedanticDependencyScopeEnforcer dependencyScopeEnforcer) {
if (CompoundPedanticEnforcer.this.compileDependencies != null) {
dependencyScopeEnforcer.setCompileDependencies(CompoundPedanticEnforcer.this.compileDependencies);
}
if (CompoundPedanticEnforcer.this.providedDependencies != null) {
dependencyScopeEnforcer.setProvidedDependencies(CompoundPedanticEnforcer.this.providedDependencies);
}
if (CompoundPedanticEnforcer.this.runtimeDependencies != null) {
dependencyScopeEnforcer.setRuntimeDependencies(CompoundPedanticEnforcer.this.runtimeDependencies);
}
if (CompoundPedanticEnforcer.this.systemDependencies != null) {
dependencyScopeEnforcer.setSystemDependencies(CompoundPedanticEnforcer.this.systemDependencies);
}
if (CompoundPedanticEnforcer.this.testDependencies != null) {
dependencyScopeEnforcer.setTestDependencies(CompoundPedanticEnforcer.this.testDependencies);
}
if (CompoundPedanticEnforcer.this.importDependencies != null) {
dependencyScopeEnforcer.setImportDependencies(CompoundPedanticEnforcer.this.importDependencies);
}
}
@Override
public void visit(PedanticPluginManagementOrderEnforcer enforcer) {
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.pluginManagementOrderBy)) {
enforcer.setOrderBy(CompoundPedanticEnforcer.this.pluginManagementOrderBy);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.pluginManagementGroupIdPriorities)) {
enforcer.setGroupIdPriorities(CompoundPedanticEnforcer.this.pluginManagementGroupIdPriorities);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.pluginManagementArtifactIdPriorities)) {
enforcer.setArtifactIdPriorities(CompoundPedanticEnforcer.this.pluginManagementArtifactIdPriorities);
}
}
@Override
public void visit(PedanticPluginConfigurationEnforcer enforcer) {
if (CompoundPedanticEnforcer.this.managePluginVersions != null) {
enforcer.setManageVersions(CompoundPedanticEnforcer.this.managePluginVersions);
}
if (CompoundPedanticEnforcer.this.managePluginConfigurations != null) {
enforcer.setManageConfigurations(CompoundPedanticEnforcer.this.managePluginConfigurations);
}
if (CompoundPedanticEnforcer.this.managePluginDependencies != null) {
enforcer.setManageDependencies(CompoundPedanticEnforcer.this.managePluginDependencies);
}
}
@Override
public void visit(PedanticPluginManagementLocationEnforcer enforcer) {
if (CompoundPedanticEnforcer.this.allowParentPomsForPluginManagement != null) {
enforcer.setAllowParentPoms(CompoundPedanticEnforcer.this.allowParentPomsForPluginManagement);
}
if (!Strings.isNullOrEmpty(CompoundPedanticEnforcer.this.pluginManagingPoms)) {
enforcer.setPluginManagingPoms(CompoundPedanticEnforcer.this.pluginManagingPoms);
}
}
@Override
public void visit(CompoundPedanticEnforcer enforcer) {
// nothing to do.
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy