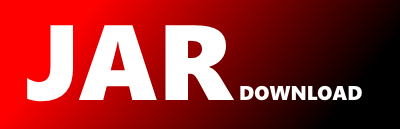
com.github.ferstl.maven.pomenforcers.PedanticPluginManagementLocationEnforcer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pedantic-pom-enforcers Show documentation
Show all versions of pedantic-pom-enforcers Show documentation
The Pedantic POM Enforcers consist of serveral Maven enforcer rules that help you keep your
project setup consistent and organized.
/*
* Copyright (c) 2012 - 2015 by Stefan Ferstl
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.ferstl.maven.pomenforcers;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import org.apache.maven.project.MavenProject;
import com.github.ferstl.maven.pomenforcers.model.ArtifactModel;
import com.github.ferstl.maven.pomenforcers.util.CommaSeparatorUtils;
import com.github.ferstl.maven.pomenforcers.util.EnforcerRuleUtils;
import static com.github.ferstl.maven.pomenforcers.ErrorReport.toList;
import static com.github.ferstl.maven.pomenforcers.model.functions.StringToArtifactTransformer.stringToArtifactModel;
/**
* Enforces that only a well-defined set of POMs may declare plugin management.
*
* ### Example
* <rules>
* <pluginManagemenLocation implementation="com.github.ferstl.maven.pomenforcers.PedanticPluginManagementLocationEnforcer">
* <!-- Only these POMs may declare plugin management -->
* <pluginManagingPoms>com.example.myproject:parent,com.example.myproject:subparent</pluginManagingPoms>
* </pluginManagemenLocation>
* </rules>
*
* @id {@link PedanticEnforcerRule#PLUGIN_MANAGEMENT_LOCATION}
* @since 1.0.0
*/
public class PedanticPluginManagementLocationEnforcer extends AbstractPedanticEnforcer {
private boolean allowParentPoms;
private final Set pluginManagingPoms;
public PedanticPluginManagementLocationEnforcer() {
this.allowParentPoms = false;
this.pluginManagingPoms = new HashSet<>();
}
@Override
protected PedanticEnforcerRule getDescription() {
return PedanticEnforcerRule.PLUGIN_MANAGEMENT_LOCATION;
}
@Override
protected void accept(PedanticEnforcerVisitor visitor) {
visitor.visit(this);
}
@Override
protected void doEnforce(ErrorReport report) {
MavenProject mavenProject = EnforcerRuleUtils.getMavenProject(getHelper());
if (containsPluginManagement() && !isPluginManagementAllowed(mavenProject)) {
report.addLine("Only these POMs are allowed to manage plugins:")
.addLine(toList(Collections.singletonList("All parent POMs, i.e. POMs with pom ")))
.addLine(toList(this.pluginManagingPoms));
}
}
/**
* Indicates whether parent POMs are generally allowed to manage plugins.
* @param allowParentPoms
* @configParam
* @default false
* @since 1.2.0
*/
public void setAllowParentPoms(boolean allowParentPoms) {
this.allowParentPoms = allowParentPoms;
}
/**
* Comma separated list of POMs that may declare <pluginManagement>
. Each POM has
* to be defined in the format groupId:artifactId
.
* @param pluginManagingPoms Comma separated list of POMs that may declare plugin management.
* @configParam
* @default n/a
* @since 1.0.0
*/
public void setPluginManagingPoms(String pluginManagingPoms) {
CommaSeparatorUtils.splitAndAddToCollection(pluginManagingPoms, this.pluginManagingPoms, stringToArtifactModel());
}
private boolean containsPluginManagement() {
return !getProjectModel().getManagedPlugins().isEmpty();
}
private boolean isPluginManagementAllowed(MavenProject project) {
return isPluginManagementAllowedInParentPom(project)
|| isPluginManagingProject(project);
}
private boolean isPluginManagementAllowedInParentPom(MavenProject project) {
return this.allowParentPoms && "pom".equals(project.getPackaging());
}
private boolean isPluginManagingProject(MavenProject project) {
ArtifactModel projectInfo = new ArtifactModel(project.getGroupId(), project.getArtifactId(), project.getVersion());
return this.pluginManagingPoms.isEmpty() || this.pluginManagingPoms.contains(projectInfo);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy