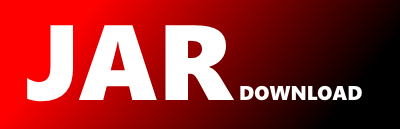
org.parboiled.support.ParsingResult Maven / Gradle / Ivy
/*
* Copyright (C) 2009-2011 Mathias Doenitz
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.parboiled.support;
import com.github.parboiled1.grappa.buffers.InputBuffer;
import com.github.parboiled1.grappa.internal.NonFinalForTesting;
import com.github.parboiled1.grappa.stack.ValueStack;
import com.google.common.base.Preconditions;
import org.parboiled.Node;
import org.parboiled.errors.ParseError;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.List;
/**
* A simple container encapsulating the result of a parsing run.
*/
@NonFinalForTesting
public class ParsingResult
{
/**
* DO NOT USE DIRECTLY!
*
* Use {@link #isSuccess()} instead
*/
private final boolean matched;
/**
* DO NOT USE DIRECTLY!
*
* Use {@link #getParseTree()} instead.
*/
private final Node parseTreeRoot;
/**
* DO NOT USE DIRECTLY!
*
* Use {@link #getTopStackValue()} instead
*/
private final V resultValue;
/**
* DO NOT USE DIRECTLY!
*
* Use {@link #getValueStack()} instead
*/
private final ValueStack valueStack;
/**
* DO NOT USE DIRECTLY!
*
* Use {@link #getParseErrors()} instead
*/
private final List parseErrors;
/**
* DO NOT USE DIRECTLY!
*
* Use {@link #getInputBuffer()} instead
*/
private final InputBuffer inputBuffer;
/**
* Creates a new ParsingResult.
*
* @param matched true if the rule matched the input
* @param parseTreeRoot the parse tree root node
* @param valueStack the value stack of the parsing run
* @param parseErrors the list of parse errors
* @param inputBuffer the input buffer
*/
public ParsingResult(final boolean matched, final Node parseTreeRoot,
@Nonnull final ValueStack valueStack,
@Nonnull final List parseErrors,
@Nonnull final InputBuffer inputBuffer)
{
this.matched = matched;
this.parseTreeRoot = parseTreeRoot;
this.valueStack = Preconditions.checkNotNull(valueStack);
resultValue = valueStack.isEmpty() ? null : valueStack.peek();
this.parseErrors = Preconditions.checkNotNull(parseErrors);
this.inputBuffer = Preconditions.checkNotNull(inputBuffer);
}
/**
* Return true if this parse result is a match
*
* @return see description
*/
public boolean isSuccess()
{
return matched;
}
/**
* Gets the generated parse tree, if any
*
* @return the tree, or (unfortunately) {@code null} if no tree
*/
@Nullable
public Node getParseTree()
{
return parseTreeRoot;
}
/**
* Gets the value at the top of the stack, if any
*
* Note that unfortunately the top of the stack can also be null, so this
* method is not reliable.
*
* @return see description
*/
@Nullable
public V getTopStackValue()
{
return resultValue;
}
/**
* Get the value stack
*
* @return the value stack used during the parsing run
*/
@Nonnull
public ValueStack getValueStack()
{
return valueStack;
}
/**
* Get the parse errors generated by the parsing process, if any
*
* @return see description
* @see #isSuccess()
*/
@Nonnull
public List getParseErrors()
{
return parseErrors;
}
/**
* Get the input buffer used by the parsing run
*
* @return see description
*/
@Nonnull
public InputBuffer getInputBuffer()
{
return inputBuffer;
}
/**
* Has this result collected any parse errors?
*
* note: this method does not guarantee that the result
* is a success; for this use {@link #isSuccess()} instead.
*
* @return true if the parse error list is not empty
*/
// TODO: not clear whether parseErrors can be null
public boolean hasCollectedParseErrors()
{
return !parseErrors.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy