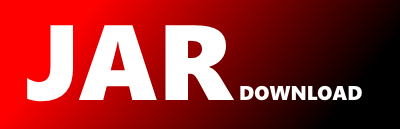
com.github.fge.jsonschema.processing.validation.ObjectSchemaSelector Maven / Gradle / Ivy
/*
* Copyright (c) 2013, Francis Galiegue
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Lesser GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Lesser GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.github.fge.jsonschema.processing.validation;
import com.fasterxml.jackson.databind.JsonNode;
import com.github.fge.jsonschema.ref.JsonPointer;
import com.github.fge.jsonschema.util.RhinoHelper;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import java.util.Collections;
import java.util.List;
public final class ObjectSchemaSelector
{
private static final JsonPointer PROPERTIES
= JsonPointer.empty().append("properties");
private static final JsonPointer PATTERNPROPERTIES
= JsonPointer.empty().append("patternProperties");
private static final JsonPointer ADDITIONALPROPERTIES
= JsonPointer.empty().append("additionalProperties");
private final List properties;
private final List patternProperties;
private final boolean hasAdditional;
ObjectSchemaSelector(final JsonNode digest)
{
hasAdditional = digest.get("hasAdditional").booleanValue();
List list;
list = Lists.newArrayList();
for (final JsonNode node: digest.get("properties"))
list.add(node.textValue());
properties = ImmutableList.copyOf(list);
list = Lists.newArrayList();
for (final JsonNode node: digest.get("patternProperties"))
list.add(node.textValue());
patternProperties = ImmutableList.copyOf(list);
}
Iterable selectSchemas(final String memberName)
{
final List list = Lists.newArrayList();
if (properties.contains(memberName))
list.add(PROPERTIES.append(memberName));
for (final String regex: patternProperties)
if (RhinoHelper.regMatch(regex, memberName))
list.add(PATTERNPROPERTIES.append(regex));
if (!list.isEmpty())
return ImmutableList.copyOf(list);
return hasAdditional
? ImmutableList.of(ADDITIONALPROPERTIES)
: Collections.emptyList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy