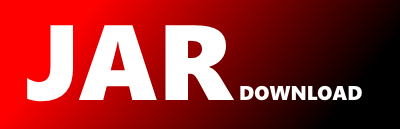
com.github.fge.jsonschema.library.Keyword Maven / Gradle / Ivy
/*
* Copyright (c) 2013, Francis Galiegue
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Lesser GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Lesser GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.github.fge.jsonschema.library;
import com.github.fge.jsonschema.exceptions.unchecked.ValidationConfigurationError;
import com.github.fge.jsonschema.keyword.digest.Digester;
import com.github.fge.jsonschema.keyword.syntax.SyntaxChecker;
import com.github.fge.jsonschema.keyword.validator.KeywordValidator;
import com.github.fge.jsonschema.report.ProcessingMessage;
import com.github.fge.jsonschema.util.Frozen;
import java.lang.reflect.Constructor;
import static com.github.fge.jsonschema.messages.ValidationConfigurationMessages.*;
/**
* Frozen keyword
*
* @see KeywordBuilder
*/
public final class Keyword
implements Frozen
{
/**
* Name of this keyword
*/
final String name;
/**
* Syntax checker
*/
final SyntaxChecker syntaxChecker;
/**
* Digester
*/
final Digester digester;
/**
* {@link KeywordValidator} constructor
*/
final Constructor extends KeywordValidator> constructor;
/**
* Instantiate a new keyword builder
*
* @param name the name for this keyword
* @return a new {@link KeywordBuilder}
* @throws ValidationConfigurationError provided name is null
* @see KeywordBuilder#KeywordBuilder(String)
*/
public static KeywordBuilder newBuilder(final String name)
{
return new KeywordBuilder(name);
}
/**
* Build a frozen keyword out of a thawed one
*
* @param builder the keyword builder to build from
* @see KeywordBuilder#freeze()
* @throws ValidationConfigurationError no syntax checker defined, or a
* validator class is defined but no digester has been found
*/
Keyword(final KeywordBuilder builder)
{
name = builder.name;
syntaxChecker = builder.syntaxChecker;
if (syntaxChecker == null)
throw new ValidationConfigurationError(new ProcessingMessage()
.message(NO_CHECKER));
if (builder.constructor != null && builder.digester == null)
throw new ValidationConfigurationError(new ProcessingMessage()
.message(MALFORMED_KEYWORD));
digester = builder.digester;
constructor = builder.constructor;
}
/**
* Create a thawed version of this keyword
*
* @return a {@link KeywordBuilder}
* @see KeywordBuilder#KeywordBuilder(Keyword)
*/
@Override
public KeywordBuilder thaw()
{
return new KeywordBuilder(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy