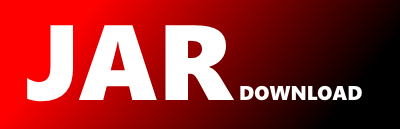
com.github.fgiannesini.libsass.gradle.plugin.tasks.CompileLibSassWithWatchTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libsass-gradle-plugin Show documentation
Show all versions of libsass-gradle-plugin Show documentation
A gradle plugin to compile scss with libsass.
package com.github.fgiannesini.libsass.gradle.plugin.tasks;
import java.io.IOException;
import java.nio.file.FileSystems;
import java.nio.file.Path;
import java.nio.file.StandardWatchEventKinds;
import java.nio.file.WatchEvent;
import java.nio.file.WatchKey;
import java.nio.file.WatchService;
import org.gradle.api.DefaultTask;
import org.gradle.api.logging.Logger;
import org.gradle.api.tasks.TaskAction;
import io.bit3.jsass.CompilationException;
/**
*
* Continuous compilation by watching files modifications in a directory
*
*/
public class CompileLibSassWithWatchTask extends DefaultTask {
@Override
public String getDescription() {
return "Continous compilation of sass/scss files to css (with optional source map) with LibSass";
}
@TaskAction
public void compileLibSass() throws CompilationException {
final Logger logger = this.getLogger();
final CompileLibSassTaskDelegate compileLibSassTaskDelegate = new CompileLibSassTaskDelegate(
this.getProject(), logger);
final Path watchedDirectory = this
.getWatchedDirectory(compileLibSassTaskDelegate);
try {
final WatchService watcher = FileSystems.getDefault()
.newWatchService();
watchedDirectory.register(watcher,
StandardWatchEventKinds.ENTRY_CREATE,
StandardWatchEventKinds.ENTRY_DELETE,
StandardWatchEventKinds.ENTRY_MODIFY);
logger.info("Watch Service registered for dir: "
+ watchedDirectory.getFileName());
while (true) {
WatchKey key;
try {
key = watcher.take();
} catch (final InterruptedException ex) {
logger.error("Wath Service interrupted", ex);
return;
}
for (final WatchEvent> event : key.pollEvents()) {
final WatchEvent.Kind> kind = event.kind();
@SuppressWarnings("unchecked")
final WatchEvent ev = (WatchEvent) event;
final Path fileName = ev.context();
logger.info(kind.name() + ": " + fileName);
try {
compileLibSassTaskDelegate.compile();
} catch (final CompilationException ce) {
logger.error(
"Error during compilation, see above for details");
}
}
final boolean valid = key.reset();
if (!valid) {
break;
}
}
} catch (final IOException ex) {
logger.error("Problem on Watch Service", ex);
}
}
/**
* Check and build watched directory path
*
* @param compileLibSassTaskDelegate
* @return
*/
private Path getWatchedDirectory(
final CompileLibSassTaskDelegate compileLibSassTaskDelegate) {
final Path watchedDirectory = compileLibSassTaskDelegate
.getWatchedDirectoryPath();
if (watchedDirectory == null) {
throw new IllegalArgumentException(
"watchedDirectoryPath must be set");
}
if (!watchedDirectory.toFile().exists()) {
throw new IllegalArgumentException(
watchedDirectory.toAbsolutePath().toString()
+ "doesn't exist");
}
return watchedDirectory;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy