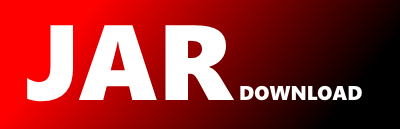
com.github.fhuss.storm.cassandra.CassandraContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of storm-cassandra Show documentation
Show all versions of storm-cassandra Show documentation
Apache Storm API Implementation for Cassandra CQL
The newest version!
package com.github.fhuss.storm.cassandra;
import com.datastax.driver.core.Cluster;
import com.github.fhuss.storm.cassandra.client.CassandraConf;
import com.github.fhuss.storm.cassandra.client.ClusterFactory;
import com.github.fhuss.storm.cassandra.client.SimpleClient;
import com.github.fhuss.storm.cassandra.client.SimpleClientProvider;
import com.github.fhuss.storm.cassandra.client.impl.DefaultClient;
import com.github.fhuss.storm.cassandra.context.BaseBeanFactory;
import com.github.fhuss.storm.cassandra.context.WorkerCtx;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Map;
/**
* @author fhussonnois
*/
public class CassandraContext extends WorkerCtx implements SimpleClientProvider {
/**
* Creates a new {@link CassandraContext} instance.
*/
public CassandraContext() {
register(SimpleClient.class, new ClientFactory());
register(CassandraConf.class, new CassandraConfFactory());
register(Cluster.class, new ClusterFactory());
}
/**
* {@inheritDoc}
*/
@Override
public SimpleClient getClient(Map config) {
SimpleClient client = getWorkerBean(SimpleClient.class, config);
if (client.isClose() )
client = getWorkerBean(SimpleClient.class, config, true);
return client;
}
/**
* Simple class to make {@link CassandraConf} from a Storm topology configuration.
*/
public static final class CassandraConfFactory extends BaseBeanFactory {
/**
* {@inheritDoc}
*/
@Override
protected CassandraConf make(Map stormConf) {
return new CassandraConf(stormConf);
}
}
/**
* Simple class to make {@link ClientFactory} from a Storm topology configuration.
*/
public static final class ClientFactory extends BaseBeanFactory {
private static final Logger LOG = LoggerFactory.getLogger(ClientFactory.class);
/**
* {@inheritDoc}
*/
@Override
protected SimpleClient make(Map stormConf) {
Cluster cluster = this.context.getWorkerBean(Cluster.class, stormConf);
if( cluster.isClosed() ) {
LOG.warn("Cluster is closed - trigger new initialization!");
cluster = this.context.getWorkerBean(Cluster.class, stormConf, true);
}
CassandraConf config = this.context.getWorkerBean(CassandraConf.class, stormConf);
return new DefaultClient(cluster, config.getKeyspace());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy