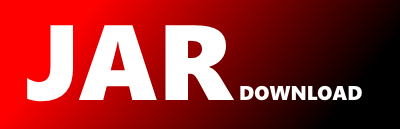
com.github.fierioziy.particlenativeapi.api.ServerConnection Maven / Gradle / Ivy
package com.github.fierioziy.particlenativeapi.api;
import com.github.fierioziy.particlenativeapi.api.utils.PlayerPredicate;
import org.bukkit.Location;
import org.bukkit.entity.Player;
import java.util.Collection;
/**
* Interface used to handle packet sending. All particles lists extends it
* to provide sending packets functionality on top of creating particle packets.
*
* It provides a non-reflective methods that uses NMS PlayerConnection
* to send them.
*/
@SuppressWarnings("unused")
public interface ServerConnection {
/**
* Creates a non-reflective wrapper of
* player's NMS PlayerConnection
instance.
*
* A generated code for this method looks roughly like this:
* {@code
* PlayerConnection createPlayerConnection(Player player) {
* return new PlayerConnection_Impl(player);
* }
* }
*
* If you plan to send more than 4-5 packets to one
* of Player
somewhere, then using this wrapper slightly faster
* than using particles lists due to
* caching NMS PlayerConnection
directly in field.
*
* It is better not to cache it long-term (for ex.
* in HashMap/ArrayList
etc.) and any complications to do it
* anyways will be significantly slower than particles lists.
*
* Obtaining PlayerConnection
from particles lists is fast, really.
*
* @param player a player from which PlayerConnection
should be obtained.
*
* @return a non-reflective PlayerConnection
wrapper of
* this player's NMS PlayerConnection
* @see PlayerConnection
*/
PlayerConnection createPlayerConnection(Player player);
/**
* Sends packet to a Player
.
*
* A generated code for this method looks roughly like this:
* {@code
* void sendPacket(Player player, Object packet) {
* ((CraftPlayer) player).getHandle().playerConnection
* .sendPacket((Packet) packet);
* }
* }
*
* A packet parameter must be an instance of Minecraft packet interface.
* Otherwise, you might get ClassCastException
on packet parameter.
*
* You can use this method to send other packet than instances created using
* this API. Any valid Minecraft packet can be used by this method.
*
* @param player a player to which send a packet object.
* @param packet a valid Minecraft packet created either by this API or
* via reflections.
*
* @throws ClassCastException when provided packet object is not
* an instance of Minecraft packet interface.
*/
void sendPacket(Player player, Object packet);
/**
* Sends packet to each Player
.
*
* A generated code for this method looks roughly like this:
* {@code
* void sendPacket(Collection players, Object packet) {
* Packet nmsPacket = (Packet) packet;
*
* int length = players.size();
* Iterator it = players.iterator();
*
* while (length > 0) {
* ((CraftPlayer) it.next()).getHandle().playerConnection
* .sendPacket(nmsPacket);
* --length;
* }
* }
* }
*
* A packet parameter must be an instance of Minecraft packet interface.
* Otherwise, you might get ClassCastException
on packet parameter.
*
* You can use this method to send other packet than instances created using
* this API. Any valid Minecraft packet can be used by this method.
*
* @param players a Collection
of players to which send a packet object.
* @param packet a valid Minecraft packet created either by this API or
* via reflections.
*
* @throws ClassCastException when provided packet object is not
* an instance of Minecraft packet interface.
*/
void sendPacket(Collection players, Object packet);
/**
* Sends packet to each Player
that matches predicate.
*
* A generated code for this method looks roughly like this:
* {@code
* void sendPacketIf(Collection players, Object packet, PlayerPredicate predicate) {
* Packet nmsPacket = (Packet) packet;
*
* int length = players.size();
* Iterator it = players.iterator();
*
* while (length > 0) {
* CraftPlayer p = (CraftPlayer) it.next();
* if (predicate.shouldSend(p)) {
* p.getHandle().playerConnection
* .sendPacket(nmsPacket);
* }
* --length;
* }
* }
* }
*
* A packet parameter must be an instance of Minecraft packet interface.
* Otherwise, you might get ClassCastException
on packet parameter.
*
* You can use this method to send other packet than instances created using
* this API. Any valid Minecraft packet can be used by this method.
*
* @param players a Collection
of players to which send a packet object.
* @param packet a valid Minecraft packet created either by this API or
* via reflections.
* @param predicate a PlayerPredicate used if packet should be send.
*
* @throws ClassCastException when provided packet object is not
* an instance of Minecraft packet interface.
*/
void sendPacketIf(Collection players, Object packet, PlayerPredicate predicate);
/**
* Sends a packet to every player in given radius.
*
* Technically speaking, gets all players from loc
parameter's world
* and send packet to every player in radius.
*
* A generated code for this method looks (roughly) like this:
* {@code
* void sendPacket(Location loc, double radius, Object packet) {
* radius *= radius;
* Packet nmsPacket = (Packet) packet;
*
* double x = loc.getX();
* double y = loc.getY();
* double z = loc.getZ();
*
* // this initializations are optimized
* int length = loc.getWorld().getPlayers().size();
* Iterator it = loc.getWorld().getPlayers().iterator();
*
* while (length > 0) {
* CraftPlayer p = (CraftPlayer) it.next();
* Location pLoc = p.getLocation();
*
* // pseudo code if statement is optimized
* if (( (pLoc.getX() - x)^2
* + (pLoc.getY() - y)^2
* + (pLoc.getZ() - z)^2) <= radius) {
* p.getHandle().playerConnection.sendPacket(nmsPacket);
* }
*
* --length;
* }
* }
* }
*
* This method should be a little faster than normal for-each loop
* with radius check due to few bytecode optimizations:
*
* - one packet cast per entire loop,
* - duplicating list reference around
*
length
and it
variables,
* - duplicating subtraction results around radius check,
* - using traditional
for
loop with
* cached list size instead of hasNext
interface
* method check on each iteration.
*
*
* A packet parameter must be an instance of Minecraft packet interface.
* Otherwise, you might get ClassCastException
on packet parameter.
*
* You can use this method to send other packet than instances created using
* this API. Any valid Minecraft packet can be used by this method.
*
* @param loc a Location
containing position.
* @param radius a spherical radius around which send packet to
* nearby players.
* @param packet a valid Minecraft packet created either by this API or
* via reflections.
* @throws ClassCastException when provided packet object is not
* an instance of Minecraft packet interface.
*/
void sendPacket(Location loc, double radius, Object packet);
/**
* Sends a packet to every player in given radius
* that matches predicate.
*
* Technically speaking, gets all players from loc
parameter's world
* and send packet to every player in radius that matches predicate.
*
* Predicate is executed after radius check.
*
* A generated code for this method looks (roughly) like this:
* {@code
* void sendPacketIf(Location loc, double radius, Object packet, PlayerPredicate predicate) {
* radius *= radius;
* Packet nmsPacket = (Packet) packet;
*
* double x = loc.getX();
* double y = loc.getY();
* double z = loc.getZ();
*
* // this initializations are optimized
* int length = loc.getWorld().getPlayers().size();
* Iterator it = loc.getWorld().getPlayers().iterator();
*
* while (length > 0) {
* CraftPlayer p = (CraftPlayer) it.next();
* Location pLoc = p.getLocation();
*
* // pseudo code if statement is optimized
* if (( (pLoc.getX() - x)^2
* + (pLoc.getY() - y)^2
* + (pLoc.getZ() - z)^2) <= radius
* && predicate.shouldSend(p)) {
* p.getHandle().playerConnection.sendPacket(nmsPacket);
* }
*
* --length;
* }
* }
* }
*
* This method should be a little faster than normal for-each loop
* with radius check due to few bytecode optimizations:
*
* - one packet cast per entire loop,
* - duplicating list reference around
*
length
and it
variables,
* - duplicating subtraction results around radius check,
* - using traditional
for
loop with
* cached list size instead of hasNext
interface
* method check on each iteration.
*
*
* A packet parameter must be an instance of Minecraft packet interface.
* Otherwise, you might get ClassCastException
on packet parameter.
*
* You can use this method to send other packet than instances created using
* this API. Any valid Minecraft packet can be used by this method.
*
* @param loc a Location
containing position.
* @param radius a spherical radius around which send packet to
* nearby players.
* @param packet a valid Minecraft packet created either by this API or
* via reflections.
* @param predicate a PlayerPredicate used if packet should be send.
*
* @throws ClassCastException when provided packet object is not
* an instance of Minecraft packet interface.
*/
void sendPacketIf(Location loc, double radius, Object packet, PlayerPredicate predicate);
}