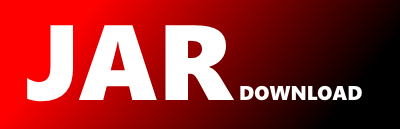
com.github.fierioziy.ParticleNativeAPI Maven / Gradle / Ivy
Show all versions of ParticleNativeAPI Show documentation
package com.github.fierioziy;
import com.github.fierioziy.api.Particles_1_13;
import com.github.fierioziy.api.Particles_1_8;
import com.github.fierioziy.api.PlayerConnection;
import com.github.fierioziy.api.ServerConnection;
import com.github.fierioziy.asm.ParticlesASM;
import com.github.fierioziy.asm.ServerConnectionASM;
import com.github.fierioziy.utils.TempClassLoader;
import org.bukkit.plugin.java.JavaPlugin;
import org.objectweb.asm.Type;
/**
* A main JavaPlugin
instance.
*
* It is responsible for providing class implementation for
* particle type list. If any error occurs during class
* generation, a isValid
method will return false
* and any API access to this instance will
* throw IllegalStateException
.
*
* Therefore, before accessing any particles list you must
* check, if plugin API is valid
* by a isValid
method call.
*/
public class ParticleNativeAPI extends JavaPlugin {
private static ParticleNativeAPI INSTANCE = null;
private boolean isValid = false;
/**
* A class loader instance used to define class implementations
* by a bytecode generated using ASM.
*/
private TempClassLoader cl;
/*
* Dynamically generated implementations of interfaces.
*/
private ServerConnection serverConnection;
private Particles_1_8 particles_1_8;
private Particles_1_13 particles_1_13;
@Override
public void onLoad() {
INSTANCE = this;
isValid = false;
cl = new TempClassLoader(this.getClassLoader());
try {
String packageVersion = getServer().getClass().getPackage().getName().split("\\.")[3];
ServerConnectionASM scASM = new ServerConnectionASM(packageVersion);
define(PlayerConnection.class, scASM.createPlayerConnection());
serverConnection = defineAndGet(
ServerConnection.class,
scASM.createServerConnection()
);
ParticlesASM ptASM = new ParticlesASM(packageVersion, cl);
particles_1_8 = defineAndGet(
Particles_1_8.class,
ptASM.createParticles_1_8()
);
particles_1_13 = defineAndGet(
Particles_1_13.class,
ptASM.createParticles_1_13()
);
isValid = true;
} catch (Exception e) {
isValid = false;
getLogger().severe("Failed to dynamically create class library using ASM.");
getLogger().severe("This API might not be compatible with current server version.");
getLogger().severe("If you suspect it is a bug, report it on issue tracker on plugin's github page with provided stacktrace:");
e.printStackTrace();
}
}
@Override
public void onEnable() {
if (!isValid) {
this.setEnabled(false);
}
}
@Override
public void onDisable() {
INSTANCE = null;
isValid = false;
cl = null;
serverConnection = null;
particles_1_8 = null;
particles_1_13 = null;
}
/**
* Gets this plugin's instance
* @return a ParticleNativeAPI
plugin's instance.
*/
public static ParticleNativeAPI getPlugin() {
return INSTANCE;
}
/**
* Checks if an API has been properly generated and
* is ready for use.
*
* @return true if API has been successfully created, false otherwise.
*/
public boolean isValid() {
return isValid;
}
private T defineAndGet(Class clazz, byte[] code) throws Exception {
return clazz.cast(
define(clazz, code).getConstructor().newInstance()
);
}
private Class> define(Class> clazz, byte[] code) {
return cl.defineClass(
Type.getType(clazz).getClassName() + "_Impl",
code
);
}
/**
* Gets instance of interface holding particle types
* prior to 1.13.
*
* All particle list interfaces holds same particle types
* where possible (for ex. FLAME particle from this instance should also be present
* in other particle list version if it is same particle type or if particle type
* handling haven't changed significantly.
*
* You should check if API has been successfully generated
* using isValid
method.
*
* Otherwise, this method might throw IllegalStateException
if class
* generation failed.
*
* @return a valid Particles_1_8
instance.
* @throws IllegalStateException if error occured during class generation.
*/
public Particles_1_8 getParticles_1_8() {
if (!isValid) {
throw new IllegalStateException("Tried to obtain invalid Particles_1_8 instance.");
}
return particles_1_8;
}
/**
* Gets instance of interface holding particle types
* since 1.13.
*
* All particle list interfaces holds same particle types
* where possible (for ex. FLAME particle from this instance should also be present
* in other particle list version if it is same particle type or if particle type
* handling haven't changed significantly.
*
* You should check if API has been successfully generated
* using isValid
method.
* Otherwise, this method will throw IllegalStateException
if class
* generation failed.
*
* @return a valid Particles_1_13
instance.
* @throws IllegalStateException if error occured during class generation.
*/
public Particles_1_13 getParticles_1_13() {
if (!isValid) {
throw new IllegalStateException("Tried to obtain invalid Particles_1_13 instance.");
}
return particles_1_13;
}
/**
* Gets instance of ServerConnection
.
*
* You should check if API has been successfully generated
* using isValid
method.
* Otherwise, this method will throw IllegalStateException
if class
* generation failed.
*
* @return a valid ServerConnection
instance.
* @throws IllegalStateException if error occured during class generation.
*/
public ServerConnection getServerConnection() {
if (!isValid) {
throw new IllegalStateException("Tried to obtain invalid ServerConnection instance.");
}
return serverConnection;
}
}