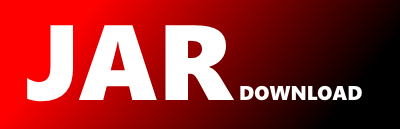
com.github.fierioziy.api.PlayerConnection Maven / Gradle / Ivy
Show all versions of ParticleNativeAPI Show documentation
package com.github.fierioziy.api;
/**
* Interface used to handle packet sending.
*
* It it a non-reflective wrapper of NMS PlayerConnection
* that is used to send Minecraft packets.
*
* Roughly speaking, it stores and uses a certain player's NMS PlayerConnection
.
*
* If you plan to send more than 4-5 packets to one
* of Players somewhere, then using this wrapper will be
* more beneficial (faster) than using ServerConnection
due
* to caching NMS PlayerConnection directly in field.
*
* It is better not to cache it long-term (for ex.
* in HashMap/ArrayList etc.) and any complications to do it
* anyways will be significantly slower than ServerConnection
.
*
* Obtaining PlayerConnection
from ServerConnection
is fast, really.
*
* It is instantiated by ServerConnection
instance and should
* only be obtained from it.
* @see ServerConnection
*/
public interface PlayerConnection {
/**
* Sends packet to a Player using its NMS PlayerConnection
.
*
* A generated code for this method looks roughly like this:
* {@code
* void sendPacket(Object packet) {
* playerConnection.sendPacket((Packet) packet);
* }
* }
*
* If you plan to send more than 4-5 packets to this player, then
* using this method will be more beneficial (faster) than
* using ServerConnection
due to caching NMS PlayerConnection
* directly in field.
*
* It is better not to use this wrapper long-term (for ex.
* in HashMap/ArrayList etc.) and any complications to do it
* anyways will be significantly slower than ServerConnection
.
*
* Obtaining PlayerConnection
from ServerConnection
is fast, really.
*
* A packet parameter must be an instance of Minecraft packet interface.
* Otherwise, you might get ClassCastException
on packet parameter.
*
* You can use this method to send other packet than instances created using
* this API. Any valid Minecraft packet can be used by this method.
*
* @param packet a valid Minecraft packet created either by this API or
* via reflections.
* @throws ClassCastException when provided packet object is not
* an instance of Minecraft packet interface
*/
void sendPacket(Object packet);
}