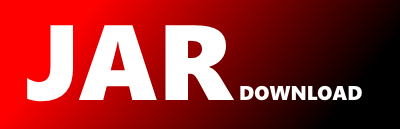
com.github.fierioziy.asm.utils.ParticleNode Maven / Gradle / Ivy
Show all versions of ParticleNativeAPI Show documentation
package com.github.fierioziy.asm.utils;
/**
* A node used by ParticleRegistry
to represent current
* particle name in certain Spigot version using ParticleVersion
enum.
*
* A ParticleNode
has a structure similar to node in doubly linked list
* that consist of reference to previous node and next node.
*
* It is used to find particle name in target Spigot version using particle name
* in current Spigot version.
*/
public class ParticleNode {
private ParticleNode prev = null;
private ParticleNode next = null;
private ParticleVersion version;
private String name;
/**
* Construct node representing certain particle based
* on its name in provided Spigot version.
*
* @param version a ParticleVersion
to which particle name belongs.
* @param name a name of this particle in provided Spigot version.
*/
ParticleNode(ParticleVersion version, String name) {
this.version = version;
this.name = name;
}
/**
* Creates new node that represents same particle name in next Spigot version.
*
* @return a new node representing same particle name in next Spigot version
* that is bound to this node.
*/
ParticleNode followDefault() {
ParticleNode node = new ParticleNode(getNextVersion(), name);
this.next = node;
node.prev = this;
return node;
}
/**
* Creates new node that represents changed particle name in next Spigot version.
*
* @return a new node representing new particle name in next Spigot version
* that is bound to this node.
*/
ParticleNode followChanged(String changedName) {
ParticleNode node = new ParticleNode(getNextVersion(), changedName);
this.next = node;
node.prev = this;
return node;
}
/**
* Creates new node that indicates, that this particle is removed in next Spigot version.
*
* @return a new node representing removed particle in next Spigot version
* that is bound to this node.
*/
ParticleNode followRemoved() {
ParticleNode node = new ParticleNode(getNextVersion(), null);
this.next = node;
node.prev = this;
return node;
}
/**
* Binds this node to the parameter ParticleNode
node.
*
* Roughly speaking, this method indicates, that this particle has been merged
* with another particle in next Spigot version.
*
* @return a parameter ParticleNode
node.
*/
ParticleNode followMerge(ParticleNode node) {
this.next = node;
return node;
}
/**
* Recursively attempts to find node of parameter Spigot version.
*
* @param target an enum representing target Spigot version.
* @return a found node with existing name in target Spigot version
* or new node representing non-existing particle name.
*/
ParticleNode find(ParticleVersion target) {
if (target.ordinal() < version.ordinal()) {
return prev != null ?
prev.find(target) : new ParticleNode(null, null);
}
else if (target.ordinal() > version.ordinal()) {
return next != null ?
next.find(target) : new ParticleNode(null, null);
}
return this;
}
/**
* Gets next spigot version based on this node.
*
* @return a ParticleVersion
enum representing
* next Spigot version.
*/
private ParticleVersion getNextVersion() {
ParticleVersion[] arr = ParticleVersion.values();
int next = version.ordinal() + 1;
if (next >= arr.length) {
throw new IndexOutOfBoundsException(
"ParticleVersion ordinal (name: " + name + " ) exceed bounds ("
+ next + " >= " + arr.length + "."
);
}
return arr[next];
}
/**
* Gets Spigot version from this node.
*
* @return a ParticleVersion
enum representing
* Spigot version of this node.
*/
public ParticleVersion getVersion() {
return version;
}
/**
* Gets particle name in this Spigot version.
*
* @return a particle name.
*/
public String getName() {
return name;
}
}