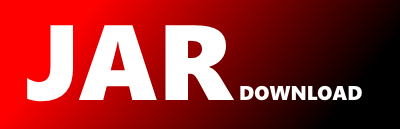
com.github.filosganga.geogson.jts.AbstractJtsCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geogson-jts Show documentation
Show all versions of geogson-jts Show documentation
GeoJSON support for Google Gson - JTS
package com.github.filosganga.geogson.jts;
import static com.google.common.collect.Iterables.transform;
import com.github.filosganga.geogson.codec.Codec;
import com.github.filosganga.geogson.model.Coordinates;
import com.github.filosganga.geogson.model.Geometry;
import com.github.filosganga.geogson.model.LineString;
import com.github.filosganga.geogson.model.LinearRing;
import com.github.filosganga.geogson.model.Point;
import com.github.filosganga.geogson.model.Polygon;
import com.github.filosganga.geogson.model.positions.SinglePosition;
import com.google.common.base.Function;
import com.google.common.collect.FluentIterable;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.GeometryFactory;
/**
* @author Filippo De Luca - [email protected]
*/
public abstract class AbstractJtsCodec> implements Codec {
/**
* {@link GeometryFactory} defining a PrecisionModel and a SRID
*/
protected final GeometryFactory geometryFactory;
/**
* Get the {@link GeometryFactory} of this {@link AbstractJtsCodec} (gotten
* via constructor)
*
* @return the {@link GeometryFactory} defining a PrecisionModel and a SRID
*/
public GeometryFactory getGeometryFactory() {
return this.geometryFactory;
}
/**
* Create a codec with a given {@link GeometryFactory}
*
* @param geometryFactory
* a {@link GeometryFactory} defining a PrecisionModel and a SRID
*/
public AbstractJtsCodec(GeometryFactory geometryFactory) {
this.geometryFactory = geometryFactory;
}
// Polygon ---
protected Function toJtsPolygonFn() {
return new Function() {
@Override
public com.vividsolutions.jts.geom.Polygon apply(Polygon input) {
return toJtsPolygon(input);
}
};
}
protected com.vividsolutions.jts.geom.Polygon toJtsPolygon(Polygon src) {
return this.geometryFactory.createPolygon(
toJtsLinearRing(src.perimeter()),
FluentIterable.from(src.holes())
.transform(toJtsLinearRingFn())
.toArray(com.vividsolutions.jts.geom.LinearRing.class)
);
}
public Function fromJtsPolygonFn() {
return new Function() {
@Override
public Polygon apply(com.vividsolutions.jts.geom.Polygon input) {
return fromJtsPolygon(input);
}
};
}
protected Polygon fromJtsPolygon(com.vividsolutions.jts.geom.Polygon src) {
return Polygon.of(fromJtsLineString(src.getExteriorRing()).toLinearRing(),
FluentIterable.from(JtsLineStringIterable.forHolesOf(src))
.transform(fromJtsLineStringFn())
.transform(LinearRing.toLinearRingFn())
);
}
// LinearRing ---
protected Function toJtsLinearRingFn() {
return new Function() {
@Override
public com.vividsolutions.jts.geom.LinearRing apply(LinearRing input) {
return toJtsLinearRing(input);
}
};
}
protected com.vividsolutions.jts.geom.LinearRing toJtsLinearRing(LinearRing src) {
return this.geometryFactory.createLinearRing(
FluentIterable.from(src.positions().children())
.transform(SinglePosition.coordinatesFn())
.transform(toJtsCoordinateFn())
.toArray(Coordinate.class)
);
}
protected Function fromJtsLinearRingFn() {
return new Function() {
@Override
public LinearRing apply(com.vividsolutions.jts.geom.LinearRing input) {
return fromJtsLinearRing(input);
}
};
}
protected LinearRing fromJtsLinearRing(com.vividsolutions.jts.geom.LinearRing src) {
return LinearRing.of(transform(JtsPointIterable.of(src), fromJtsPointFn()));
}
// LineString ---
protected Function toJtsLineStringFn() {
return new Function() {
@Override
public com.vividsolutions.jts.geom.LineString apply(LineString input) {
return toJtsLineString(input);
}
};
}
protected com.vividsolutions.jts.geom.LineString toJtsLineString(LineString src) {
return this.geometryFactory.createLineString(
FluentIterable.from(src.positions().children())
.transform(SinglePosition.coordinatesFn())
.transform(toJtsCoordinateFn())
.toArray(Coordinate.class)
);
}
protected Function fromJtsLineStringFn() {
return new Function() {
@Override
public LineString apply(com.vividsolutions.jts.geom.LineString input) {
return fromJtsLineString(input);
}
};
}
protected LineString fromJtsLineString(com.vividsolutions.jts.geom.LineString src) {
return LineString.of(transform(JtsPointIterable.of(src), fromJtsPointFn()));
}
// Point
protected static com.vividsolutions.jts.geom.Point toJtsPoint(Point src) {
return new GeometryFactory().createPoint(new Coordinate(src.lon(), src.lat()));
}
protected static Function fromJtsPointFn() {
return new Function() {
@Override
public Point apply(com.vividsolutions.jts.geom.Point input) {
return fromJtsPoint(input);
}
};
}
protected static Point fromJtsPoint(com.vividsolutions.jts.geom.Point src) {
return Point.from(fromJtsCoordinate(src.getCoordinate()));
}
protected static Function toJtsCoordinateFn() {
return ToJtsCoordinate.INSTANCE;
}
protected static Coordinates fromJtsCoordinate(com.vividsolutions.jts.geom.Coordinate src) {
return Coordinates.of(src.x, src.y);
}
private enum ToJtsCoordinate implements Function {
INSTANCE;
@Override
public Coordinate apply(com.github.filosganga.geogson.model.Coordinates input) {
return new Coordinate(input.getLon(), input.getLat());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy