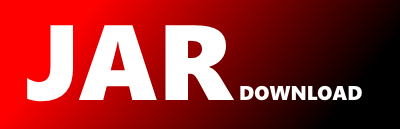
com.github.firelcw.hander.ResponseHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of easy-http Show documentation
Show all versions of easy-http Show documentation
A simple HTTP client for Java
package com.github.firelcw.hander;
import com.github.firelcw.codec.Decoder;
import com.github.firelcw.codec.SimpleDecoder;
import com.github.firelcw.exception.EasyHttpException;
import com.github.firelcw.interceptor.InterceptorOperations;
import com.github.firelcw.model.HttpRequest;
import com.github.firelcw.model.HttpResponse;
import com.github.firelcw.proxy.HttpInvocationHandler;
import com.github.firelcw.util.TypeUtils;
import java.lang.reflect.Type;
/**
* 响应处理器
* @author liaochongwei
* @date 2020/7/31 13:17
*/
public class ResponseHandler implements Handler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy