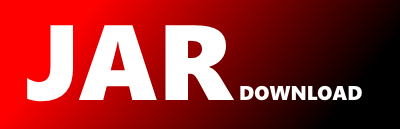
com.github.fluorumlabs.disconnect.highcharts.AnnotationsShapesOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import java.lang.String;
import javax.annotation.Nullable;
import js.lang.Any;
import js.lang.Unknown;
import js.util.collections.Array;
import org.teavm.jso.JSProperty;
/**
* (Highcharts, Highstock, Highmaps) An array of shapes for the annotation. For
* options that apply to multiple shapes, then can be added to the shapeOptions.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes
* @see https://api.highcharts.com/highstock/annotations.shapes
* @see https://api.highcharts.com/highmaps/annotations.shapes
*
*/
public interface AnnotationsShapesOptions extends Any {
/**
* (Highcharts, Highstock, Highmaps) The color of the shape's fill.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.fill
* @see https://api.highcharts.com/highstock/annotations.shapes.fill
* @see https://api.highcharts.com/highmaps/annotations.shapes.fill
*
* @implspec fill?: (ColorString|GradientColorObject);
*
*/
@JSProperty("fill")
@Nullable
Unknown getFill();
/**
* (Highcharts, Highstock, Highmaps) The color of the shape's fill.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.fill
* @see https://api.highcharts.com/highstock/annotations.shapes.fill
* @see https://api.highcharts.com/highmaps/annotations.shapes.fill
*
* @implspec fill?: (ColorString|GradientColorObject);
*
*/
@JSProperty("fill")
void setFill(GradientColorObject value);
/**
* (Highcharts, Highstock, Highmaps) The color of the shape's fill.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.fill
* @see https://api.highcharts.com/highstock/annotations.shapes.fill
* @see https://api.highcharts.com/highmaps/annotations.shapes.fill
*
* @implspec fill?: (ColorString|GradientColorObject);
*
*/
@JSProperty("fill")
void setFill(String value);
/**
* (Highcharts, Highstock, Highmaps) The height of the shape.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.height
* @see https://api.highcharts.com/highstock/annotations.shapes.height
* @see https://api.highcharts.com/highmaps/annotations.shapes.height
*
* @implspec height?: number;
*
*/
@JSProperty("height")
double getHeight();
/**
* (Highcharts, Highstock, Highmaps) The height of the shape.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.height
* @see https://api.highcharts.com/highstock/annotations.shapes.height
* @see https://api.highcharts.com/highmaps/annotations.shapes.height
*
* @implspec height?: number;
*
*/
@JSProperty("height")
void setHeight(double value);
/**
* (Highcharts, Highstock, Highmaps) Id of the marker which will be drawn at
* the final vertex of the path. Custom markers can be defined in defs
* property.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.markerEnd
* @see https://api.highcharts.com/highstock/annotations.shapes.markerEnd
* @see https://api.highcharts.com/highmaps/annotations.shapes.markerEnd
*
* @implspec markerEnd?: string;
*
*/
@JSProperty("markerEnd")
@Nullable
String getMarkerEnd();
/**
* (Highcharts, Highstock, Highmaps) Id of the marker which will be drawn at
* the final vertex of the path. Custom markers can be defined in defs
* property.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.markerEnd
* @see https://api.highcharts.com/highstock/annotations.shapes.markerEnd
* @see https://api.highcharts.com/highmaps/annotations.shapes.markerEnd
*
* @implspec markerEnd?: string;
*
*/
@JSProperty("markerEnd")
void setMarkerEnd(String value);
/**
* (Highcharts, Highstock, Highmaps) Id of the marker which will be drawn at
* the first vertex of the path. Custom markers can be defined in defs
* property.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.markerStart
* @see https://api.highcharts.com/highstock/annotations.shapes.markerStart
* @see https://api.highcharts.com/highmaps/annotations.shapes.markerStart
*
* @implspec markerStart?: string;
*
*/
@JSProperty("markerStart")
@Nullable
String getMarkerStart();
/**
* (Highcharts, Highstock, Highmaps) Id of the marker which will be drawn at
* the first vertex of the path. Custom markers can be defined in defs
* property.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.markerStart
* @see https://api.highcharts.com/highstock/annotations.shapes.markerStart
* @see https://api.highcharts.com/highmaps/annotations.shapes.markerStart
*
* @implspec markerStart?: string;
*
*/
@JSProperty("markerStart")
void setMarkerStart(String value);
/**
* (Highcharts, Highstock, Highmaps) This option defines the point to which
* the shape will be connected. It can be either the point which exists in
* the series - it is referenced by the point's id - or a new point with
* defined x, y properties and optionally axes.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.point
* @see https://api.highcharts.com/highstock/annotations.shapes.point
* @see https://api.highcharts.com/highmaps/annotations.shapes.point
*
* @implspec point?: (string|AnnotationsShapesPointOptions);
*
*/
@JSProperty("point")
@Nullable
Unknown getPoint();
/**
* (Highcharts, Highstock, Highmaps) This option defines the point to which
* the shape will be connected. It can be either the point which exists in
* the series - it is referenced by the point's id - or a new point with
* defined x, y properties and optionally axes.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.point
* @see https://api.highcharts.com/highstock/annotations.shapes.point
* @see https://api.highcharts.com/highmaps/annotations.shapes.point
*
* @implspec point?: (string|AnnotationsShapesPointOptions);
*
*/
@JSProperty("point")
void setPoint(String value);
/**
* (Highcharts, Highstock, Highmaps) This option defines the point to which
* the shape will be connected. It can be either the point which exists in
* the series - it is referenced by the point's id - or a new point with
* defined x, y properties and optionally axes.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.point
* @see https://api.highcharts.com/highstock/annotations.shapes.point
* @see https://api.highcharts.com/highmaps/annotations.shapes.point
*
* @implspec point?: (string|AnnotationsShapesPointOptions);
*
*/
@JSProperty("point")
void setPoint(AnnotationsShapesPointOptions value);
/**
* (Highcharts, Highstock, Highmaps) An array of points for the shape. This
* option is available for shapes which can use multiple points such as
* path. A point can be either a point object or a point's id.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.points
* @see https://api.highcharts.com/highstock/annotations.shapes.points
* @see https://api.highcharts.com/highmaps/annotations.shapes.points
*
* @implspec points?: Array;
*
*/
@JSProperty("points")
@Nullable
Array getPoints();
/**
* (Highcharts, Highstock, Highmaps) An array of points for the shape. This
* option is available for shapes which can use multiple points such as
* path. A point can be either a point object or a point's id.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.points
* @see https://api.highcharts.com/highstock/annotations.shapes.points
* @see https://api.highcharts.com/highmaps/annotations.shapes.points
*
* @implspec points?: Array;
*
*/
@JSProperty("points")
void setPoints(Array value);
/**
* (Highcharts, Highstock, Highmaps) The radius of the shape.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.r
* @see https://api.highcharts.com/highstock/annotations.shapes.r
* @see https://api.highcharts.com/highmaps/annotations.shapes.r
*
* @implspec r?: number;
*
*/
@JSProperty("r")
double getR();
/**
* (Highcharts, Highstock, Highmaps) The radius of the shape.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.r
* @see https://api.highcharts.com/highstock/annotations.shapes.r
* @see https://api.highcharts.com/highmaps/annotations.shapes.r
*
* @implspec r?: number;
*
*/
@JSProperty("r")
void setR(double value);
/**
* (Highcharts, Highstock, Highmaps) The color of the shape's stroke.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.stroke
* @see https://api.highcharts.com/highstock/annotations.shapes.stroke
* @see https://api.highcharts.com/highmaps/annotations.shapes.stroke
*
* @implspec stroke?: ColorString;
*
*/
@JSProperty("stroke")
@Nullable
String getStroke();
/**
* (Highcharts, Highstock, Highmaps) The color of the shape's stroke.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.stroke
* @see https://api.highcharts.com/highstock/annotations.shapes.stroke
* @see https://api.highcharts.com/highmaps/annotations.shapes.stroke
*
* @implspec stroke?: ColorString;
*
*/
@JSProperty("stroke")
void setStroke(String value);
/**
* (Highcharts, Highstock, Highmaps) The pixel stroke width of the shape.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.strokeWidth
* @see https://api.highcharts.com/highstock/annotations.shapes.strokeWidth
* @see https://api.highcharts.com/highmaps/annotations.shapes.strokeWidth
*
* @implspec strokeWidth?: number;
*
*/
@JSProperty("strokeWidth")
double getStrokeWidth();
/**
* (Highcharts, Highstock, Highmaps) The pixel stroke width of the shape.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.strokeWidth
* @see https://api.highcharts.com/highstock/annotations.shapes.strokeWidth
* @see https://api.highcharts.com/highmaps/annotations.shapes.strokeWidth
*
* @implspec strokeWidth?: number;
*
*/
@JSProperty("strokeWidth")
void setStrokeWidth(double value);
/**
* (Highcharts, Highstock, Highmaps) The type of the shape, e.g. circle or
* rectangle.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.type
* @see https://api.highcharts.com/highstock/annotations.shapes.type
* @see https://api.highcharts.com/highmaps/annotations.shapes.type
*
* @implspec type?: string;
*
*/
@JSProperty("type")
@Nullable
String getType();
/**
* (Highcharts, Highstock, Highmaps) The type of the shape, e.g. circle or
* rectangle.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.type
* @see https://api.highcharts.com/highstock/annotations.shapes.type
* @see https://api.highcharts.com/highmaps/annotations.shapes.type
*
* @implspec type?: string;
*
*/
@JSProperty("type")
void setType(String value);
/**
* (Highcharts, Highstock, Highmaps) The width of the shape.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.width
* @see https://api.highcharts.com/highstock/annotations.shapes.width
* @see https://api.highcharts.com/highmaps/annotations.shapes.width
*
* @implspec width?: number;
*
*/
@JSProperty("width")
double getWidth();
/**
* (Highcharts, Highstock, Highmaps) The width of the shape.
*
* @see https://api.highcharts.com/highcharts/annotations.shapes.width
* @see https://api.highcharts.com/highstock/annotations.shapes.width
* @see https://api.highcharts.com/highmaps/annotations.shapes.width
*
* @implspec width?: number;
*
*/
@JSProperty("width")
void setWidth(double value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy