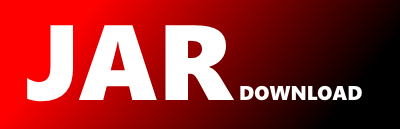
com.github.fluorumlabs.disconnect.highcharts.BoostDebugOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import js.lang.Any;
import org.teavm.jso.JSProperty;
/**
* (Highcharts, Highstock) Debugging options for boost. Useful for benchmarking,
* and general timing.
*
* @see https://api.highcharts.com/highcharts/boost.debug
* @see https://api.highcharts.com/highstock/boost.debug
*
*/
public interface BoostDebugOptions extends Any {
/**
* (Highcharts, Highstock) Show the number of points skipped through
* culling.
*
* When set to true, the number of points skipped in series processing is
* outputted. Points are skipped if they are closer than 1 pixel from each
* other.
*
* @see https://api.highcharts.com/highcharts/boost.debug.showSkipSummary
* @see https://api.highcharts.com/highstock/boost.debug.showSkipSummary
*
* @implspec showSkipSummary?: boolean;
*
*/
@JSProperty("showSkipSummary")
boolean getShowSkipSummary();
/**
* (Highcharts, Highstock) Show the number of points skipped through
* culling.
*
* When set to true, the number of points skipped in series processing is
* outputted. Points are skipped if they are closer than 1 pixel from each
* other.
*
* @see https://api.highcharts.com/highcharts/boost.debug.showSkipSummary
* @see https://api.highcharts.com/highstock/boost.debug.showSkipSummary
*
* @implspec showSkipSummary?: boolean;
*
*/
@JSProperty("showSkipSummary")
void setShowSkipSummary(boolean value);
/**
* (Highcharts, Highstock) Time the WebGL to SVG buffer copy
*
* After rendering, the result is copied to an image which is injected into
* the SVG.
*
* If this property is set to true, the time it takes for the buffer copy to
* complete is outputted.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeBufferCopy
* @see https://api.highcharts.com/highstock/boost.debug.timeBufferCopy
*
* @implspec timeBufferCopy?: boolean;
*
*/
@JSProperty("timeBufferCopy")
boolean getTimeBufferCopy();
/**
* (Highcharts, Highstock) Time the WebGL to SVG buffer copy
*
* After rendering, the result is copied to an image which is injected into
* the SVG.
*
* If this property is set to true, the time it takes for the buffer copy to
* complete is outputted.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeBufferCopy
* @see https://api.highcharts.com/highstock/boost.debug.timeBufferCopy
*
* @implspec timeBufferCopy?: boolean;
*
*/
@JSProperty("timeBufferCopy")
void setTimeBufferCopy(boolean value);
/**
* (Highcharts, Highstock) Time the building of the k-d tree.
*
* This outputs the time spent building the k-d tree used for markers etc.
*
* Note that the k-d tree is built async, and runs post-rendering.
* Following, it does not affect the performance of the rendering itself.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeKDTree
* @see https://api.highcharts.com/highstock/boost.debug.timeKDTree
*
* @implspec timeKDTree?: boolean;
*
*/
@JSProperty("timeKDTree")
boolean getTimeKDTree();
/**
* (Highcharts, Highstock) Time the building of the k-d tree.
*
* This outputs the time spent building the k-d tree used for markers etc.
*
* Note that the k-d tree is built async, and runs post-rendering.
* Following, it does not affect the performance of the rendering itself.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeKDTree
* @see https://api.highcharts.com/highstock/boost.debug.timeKDTree
*
* @implspec timeKDTree?: boolean;
*
*/
@JSProperty("timeKDTree")
void setTimeKDTree(boolean value);
/**
* (Highcharts, Highstock) Time the series rendering.
*
* This outputs the time spent on actual rendering in the console when set
* to true.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeRendering
* @see https://api.highcharts.com/highstock/boost.debug.timeRendering
*
* @implspec timeRendering?: boolean;
*
*/
@JSProperty("timeRendering")
boolean getTimeRendering();
/**
* (Highcharts, Highstock) Time the series rendering.
*
* This outputs the time spent on actual rendering in the console when set
* to true.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeRendering
* @see https://api.highcharts.com/highstock/boost.debug.timeRendering
*
* @implspec timeRendering?: boolean;
*
*/
@JSProperty("timeRendering")
void setTimeRendering(boolean value);
/**
* (Highcharts, Highstock) Time the series processing.
*
* This outputs the time spent on transforming the series data to vertex
* buffers when set to true.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeSeriesProcessing
* @see https://api.highcharts.com/highstock/boost.debug.timeSeriesProcessing
*
* @implspec timeSeriesProcessing?: boolean;
*
*/
@JSProperty("timeSeriesProcessing")
boolean getTimeSeriesProcessing();
/**
* (Highcharts, Highstock) Time the series processing.
*
* This outputs the time spent on transforming the series data to vertex
* buffers when set to true.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeSeriesProcessing
* @see https://api.highcharts.com/highstock/boost.debug.timeSeriesProcessing
*
* @implspec timeSeriesProcessing?: boolean;
*
*/
@JSProperty("timeSeriesProcessing")
void setTimeSeriesProcessing(boolean value);
/**
* (Highcharts, Highstock) Time the the WebGL setup.
*
* This outputs the time spent on setting up the WebGL context, creating
* shaders, and textures.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeSetup
* @see https://api.highcharts.com/highstock/boost.debug.timeSetup
*
* @implspec timeSetup?: boolean;
*
*/
@JSProperty("timeSetup")
boolean getTimeSetup();
/**
* (Highcharts, Highstock) Time the the WebGL setup.
*
* This outputs the time spent on setting up the WebGL context, creating
* shaders, and textures.
*
* @see https://api.highcharts.com/highcharts/boost.debug.timeSetup
* @see https://api.highcharts.com/highstock/boost.debug.timeSetup
*
* @implspec timeSetup?: boolean;
*
*/
@JSProperty("timeSetup")
void setTimeSetup(boolean value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy