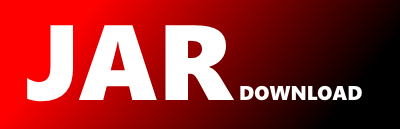
com.github.fluorumlabs.disconnect.highcharts.DrilldownOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import javax.annotation.Nullable;
import js.lang.Any;
import js.lang.Unknown;
import js.util.collections.Array;
import org.teavm.jso.JSProperty;
/**
* (Highcharts, Highstock, Highmaps) Options for drill down, the concept of
* inspecting increasingly high resolution data through clicking on chart items
* like columns or pie slices.
*
* The drilldown feature requires the drilldown.js file to be loaded, found in
* the modules directory of the download package, or online at
* code.highcharts.com/modules/drilldown.js.
*
* @see https://api.highcharts.com/highcharts/drilldown
* @see https://api.highcharts.com/highstock/drilldown
* @see https://api.highcharts.com/highmaps/drilldown
*
*/
public interface DrilldownOptions extends Any {
/**
* (Highcharts, Highmaps) Additional styles to apply to the X axis label for
* a point that has drilldown data. By default it is underlined and blue to
* invite to interaction.
*
* In styled mode, active label styles can be set with the
* .highcharts-drilldown-axis-label
class.
*
* @see https://api.highcharts.com/highcharts/drilldown.activeAxisLabelStyle
* @see https://api.highcharts.com/highmaps/drilldown.activeAxisLabelStyle
*
* @implspec activeAxisLabelStyle?: CSSObject;
*
*/
@JSProperty("activeAxisLabelStyle")
@Nullable
CSSObject getActiveAxisLabelStyle();
/**
* (Highcharts, Highmaps) Additional styles to apply to the X axis label for
* a point that has drilldown data. By default it is underlined and blue to
* invite to interaction.
*
* In styled mode, active label styles can be set with the
* .highcharts-drilldown-axis-label
class.
*
* @see https://api.highcharts.com/highcharts/drilldown.activeAxisLabelStyle
* @see https://api.highcharts.com/highmaps/drilldown.activeAxisLabelStyle
*
* @implspec activeAxisLabelStyle?: CSSObject;
*
*/
@JSProperty("activeAxisLabelStyle")
void setActiveAxisLabelStyle(CSSObject value);
/**
* (Highcharts, Highmaps) Additional styles to apply to the data label of a
* point that has drilldown data. By default it is underlined and blue to
* invite to interaction.
*
* In styled mode, active data label styles can be applied with the
* .highcharts-drilldown-data-label
class.
*
* @see https://api.highcharts.com/highcharts/drilldown.activeDataLabelStyle
* @see https://api.highcharts.com/highmaps/drilldown.activeDataLabelStyle
*
* @implspec activeDataLabelStyle?: (CSSObject|DrilldownActiveDataLabelStyleOptions);
*
*/
@JSProperty("activeDataLabelStyle")
@Nullable
Unknown getActiveDataLabelStyle();
/**
* (Highcharts, Highmaps) Additional styles to apply to the data label of a
* point that has drilldown data. By default it is underlined and blue to
* invite to interaction.
*
* In styled mode, active data label styles can be applied with the
* .highcharts-drilldown-data-label
class.
*
* @see https://api.highcharts.com/highcharts/drilldown.activeDataLabelStyle
* @see https://api.highcharts.com/highmaps/drilldown.activeDataLabelStyle
*
* @implspec activeDataLabelStyle?: (CSSObject|DrilldownActiveDataLabelStyleOptions);
*
*/
@JSProperty("activeDataLabelStyle")
void setActiveDataLabelStyle(CSSObject value);
/**
* (Highcharts, Highmaps) Additional styles to apply to the data label of a
* point that has drilldown data. By default it is underlined and blue to
* invite to interaction.
*
* In styled mode, active data label styles can be applied with the
* .highcharts-drilldown-data-label
class.
*
* @see https://api.highcharts.com/highcharts/drilldown.activeDataLabelStyle
* @see https://api.highcharts.com/highmaps/drilldown.activeDataLabelStyle
*
* @implspec activeDataLabelStyle?: (CSSObject|DrilldownActiveDataLabelStyleOptions);
*
*/
@JSProperty("activeDataLabelStyle")
void setActiveDataLabelStyle(DrilldownActiveDataLabelStyleOptions value);
/**
* (Highcharts) When this option is false, clicking a single point will
* drill down all points in the same category, equivalent to clicking the X
* axis label.
*
* @see https://api.highcharts.com/highcharts/drilldown.allowPointDrilldown
*
* @implspec allowPointDrilldown?: boolean;
*
*/
@JSProperty("allowPointDrilldown")
boolean getAllowPointDrilldown();
/**
* (Highcharts) When this option is false, clicking a single point will
* drill down all points in the same category, equivalent to clicking the X
* axis label.
*
* @see https://api.highcharts.com/highcharts/drilldown.allowPointDrilldown
*
* @implspec allowPointDrilldown?: boolean;
*
*/
@JSProperty("allowPointDrilldown")
void setAllowPointDrilldown(boolean value);
/**
* (Highcharts, Highmaps) Set the animation for all drilldown animations.
* Animation of a drilldown occurs when drilling between a column point and
* a column series, or a pie slice and a full pie series. Drilldown can
* still be used between series and points of different types, but animation
* will not occur.
*
* The animation can either be set as a boolean or a configuration object.
* If true
, it will use the 'swing' jQuery easing and a duration of 500
* ms. If used as a configuration object, the following properties are
* supported:
*
*
* -
*
duration
: The duration of the animation in milliseconds.
*
*
* -
*
easing
: A string reference to an easing function set on the Math
* object. See the easing demo.
*
*
*
* @see https://api.highcharts.com/highcharts/drilldown.animation
* @see https://api.highcharts.com/highmaps/drilldown.animation
*
* @implspec animation?: (boolean|AnimationOptionsObject);
*
*/
@JSProperty("animation")
@Nullable
Unknown getAnimation();
/**
* (Highcharts, Highmaps) Set the animation for all drilldown animations.
* Animation of a drilldown occurs when drilling between a column point and
* a column series, or a pie slice and a full pie series. Drilldown can
* still be used between series and points of different types, but animation
* will not occur.
*
* The animation can either be set as a boolean or a configuration object.
* If true
, it will use the 'swing' jQuery easing and a duration of 500
* ms. If used as a configuration object, the following properties are
* supported:
*
*
* -
*
duration
: The duration of the animation in milliseconds.
*
*
* -
*
easing
: A string reference to an easing function set on the Math
* object. See the easing demo.
*
*
*
* @see https://api.highcharts.com/highcharts/drilldown.animation
* @see https://api.highcharts.com/highmaps/drilldown.animation
*
* @implspec animation?: (boolean|AnimationOptionsObject);
*
*/
@JSProperty("animation")
void setAnimation(boolean value);
/**
* (Highcharts, Highmaps) Set the animation for all drilldown animations.
* Animation of a drilldown occurs when drilling between a column point and
* a column series, or a pie slice and a full pie series. Drilldown can
* still be used between series and points of different types, but animation
* will not occur.
*
* The animation can either be set as a boolean or a configuration object.
* If true
, it will use the 'swing' jQuery easing and a duration of 500
* ms. If used as a configuration object, the following properties are
* supported:
*
*
* -
*
duration
: The duration of the animation in milliseconds.
*
*
* -
*
easing
: A string reference to an easing function set on the Math
* object. See the easing demo.
*
*
*
* @see https://api.highcharts.com/highcharts/drilldown.animation
* @see https://api.highcharts.com/highmaps/drilldown.animation
*
* @implspec animation?: (boolean|AnimationOptionsObject);
*
*/
@JSProperty("animation")
void setAnimation(AnimationOptionsObject value);
/**
* (Highcharts, Highmaps) Options for the drill up button that appears when
* drilling down on a series. The text for the button is defined in
* lang.drillUpText.
*
* @see https://api.highcharts.com/highcharts/drilldown.drillUpButton
* @see https://api.highcharts.com/highmaps/drilldown.drillUpButton
*
* @implspec drillUpButton?: DrilldownDrillUpButtonOptions;
*
*/
@JSProperty("drillUpButton")
@Nullable
DrilldownDrillUpButtonOptions getDrillUpButton();
/**
* (Highcharts, Highmaps) Options for the drill up button that appears when
* drilling down on a series. The text for the button is defined in
* lang.drillUpText.
*
* @see https://api.highcharts.com/highcharts/drilldown.drillUpButton
* @see https://api.highcharts.com/highmaps/drilldown.drillUpButton
*
* @implspec drillUpButton?: DrilldownDrillUpButtonOptions;
*
*/
@JSProperty("drillUpButton")
void setDrillUpButton(DrilldownDrillUpButtonOptions value);
/**
* (Highcharts, Highmaps) An array of series configurations for the drill
* down. Each series configuration uses the same syntax as the series option
* set. These drilldown series are hidden by default. The drilldown series
* is linked to the parent series' point by its id
.
*
* @see https://api.highcharts.com/highcharts/drilldown.series
* @see https://api.highcharts.com/highmaps/drilldown.series
*
* @implspec series?: Array;
*
*/
@JSProperty("series")
@Nullable
Array getSeries();
/**
* (Highcharts, Highmaps) An array of series configurations for the drill
* down. Each series configuration uses the same syntax as the series option
* set. These drilldown series are hidden by default. The drilldown series
* is linked to the parent series' point by its id
.
*
* @see https://api.highcharts.com/highcharts/drilldown.series
* @see https://api.highcharts.com/highmaps/drilldown.series
*
* @implspec series?: Array;
*
*/
@JSProperty("series")
void setSeries(Array value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy