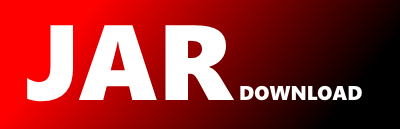
com.github.fluorumlabs.disconnect.highcharts.ExportingButtonsContextButtonOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import java.lang.FunctionalInterface;
import java.lang.String;
import javax.annotation.Nullable;
import js.extras.JsEnum;
import js.lang.Any;
import org.teavm.jso.JSFunctor;
import org.teavm.jso.JSProperty;
/**
* (Highcharts, Highstock, Highmaps) Options for the export button.
*
* In styled mode, export button styles can be applied with the
* .highcharts-contextbutton
class.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton
*
*/
public interface ExportingButtonsContextButtonOptions extends Any {
/**
* (Highcharts, Highstock, Highmaps) This option is deprecated, use titleKey
* instead.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton._titleKey
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton._titleKey
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton._titleKey
*
* @implspec _titleKey?: string;
*
*/
@JSProperty("_titleKey")
@Nullable
String get_titleKey();
/**
* (Highcharts, Highstock, Highmaps) This option is deprecated, use titleKey
* instead.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton._titleKey
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton._titleKey
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton._titleKey
*
* @implspec _titleKey?: string;
*
*/
@JSProperty("_titleKey")
void set_titleKey(String value);
/**
* (Highcharts, Highstock, Highmaps) Alignment for the buttons.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.align
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.align
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.align
*
* @implspec align?: ("center"|"left"|"right");
*
*/
@JSProperty("align")
@Nullable
Align getAlign();
/**
* (Highcharts, Highstock, Highmaps) Alignment for the buttons.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.align
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.align
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.align
*
* @implspec align?: ("center"|"left"|"right");
*
*/
@JSProperty("align")
void setAlign(Align value);
/**
* (Highcharts, Highstock, Highmaps) The pixel spacing between buttons.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.buttonSpacing
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.buttonSpacing
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.buttonSpacing
*
* @implspec buttonSpacing?: number;
*
*/
@JSProperty("buttonSpacing")
double getButtonSpacing();
/**
* (Highcharts, Highstock, Highmaps) The pixel spacing between buttons.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.buttonSpacing
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.buttonSpacing
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.buttonSpacing
*
* @implspec buttonSpacing?: number;
*
*/
@JSProperty("buttonSpacing")
void setButtonSpacing(double value);
/**
* (Highcharts, Highstock, Highmaps) The class name of the context button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.className
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.className
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.className
*
* @implspec className?: string;
*
*/
@JSProperty("className")
@Nullable
String getClassName();
/**
* (Highcharts, Highstock, Highmaps) The class name of the context button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.className
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.className
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.className
*
* @implspec className?: string;
*
*/
@JSProperty("className")
void setClassName(String value);
/**
* (Highcharts, Highstock, Highmaps) Whether to enable buttons.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.enabled
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.enabled
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.enabled
*
* @implspec enabled?: boolean;
*
*/
@JSProperty("enabled")
boolean getEnabled();
/**
* (Highcharts, Highstock, Highmaps) Whether to enable buttons.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.enabled
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.enabled
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.enabled
*
* @implspec enabled?: boolean;
*
*/
@JSProperty("enabled")
void setEnabled(boolean value);
/**
* (Highcharts, Highstock, Highmaps) Pixel height of the buttons.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.height
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.height
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.height
*
* @implspec height?: number;
*
*/
@JSProperty("height")
double getHeight();
/**
* (Highcharts, Highstock, Highmaps) Pixel height of the buttons.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.height
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.height
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.height
*
* @implspec height?: number;
*
*/
@JSProperty("height")
void setHeight(double value);
/**
* (Highcharts, Highstock, Highmaps) The class name of the menu appearing
* from the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.menuClassName
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.menuClassName
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.menuClassName
*
* @implspec menuClassName?: string;
*
*/
@JSProperty("menuClassName")
@Nullable
String getMenuClassName();
/**
* (Highcharts, Highstock, Highmaps) The class name of the menu appearing
* from the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.menuClassName
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.menuClassName
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.menuClassName
*
* @implspec menuClassName?: string;
*
*/
@JSProperty("menuClassName")
void setMenuClassName(String value);
/**
* (Highcharts, Highstock, Highmaps) A collection of strings pointing to
* config options for the menu items. The config options are defined in the
* menuItemDefinitions
option.
*
* By default, there is the "Print" menu item plus one menu item for each of
* the available export types.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.menuItems
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.menuItems
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.menuItems
*
* @implspec menuItems?: Array;
*
*/
@JSProperty("menuItems")
@Nullable
String[] getMenuItems();
/**
* (Highcharts, Highstock, Highmaps) A collection of strings pointing to
* config options for the menu items. The config options are defined in the
* menuItemDefinitions
option.
*
* By default, there is the "Print" menu item plus one menu item for each of
* the available export types.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.menuItems
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.menuItems
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.menuItems
*
* @implspec menuItems?: Array;
*
*/
@JSProperty("menuItems")
void setMenuItems(String[] value);
/**
* (Highcharts, Highstock, Highmaps) A click handler callback to use on the
* button directly instead of the popup menu.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.onclick
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.onclick
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.onclick
*
* @implspec onclick?: () => void;
*
*/
@JSProperty("onclick")
@Nullable
Onclick getOnclick();
/**
* (Highcharts, Highstock, Highmaps) A click handler callback to use on the
* button directly instead of the popup menu.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.onclick
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.onclick
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.onclick
*
* @implspec onclick?: () => void;
*
*/
@JSProperty("onclick")
void setOnclick(Onclick value);
/**
* (Highcharts, Highstock, Highmaps) The symbol for the button. Points to a
* definition function in the Highcharts.Renderer.symbols
collection. The
* default exportIcon
function is part of the exporting module.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbol
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbol
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbol
*
* @implspec symbol?: ("circle"|"diamond"|"menu"|"square"|"triangle"|"triangle-down"|"exportIcon");
*
*/
@JSProperty("symbol")
@Nullable
Symbol getSymbol();
/**
* (Highcharts, Highstock, Highmaps) The symbol for the button. Points to a
* definition function in the Highcharts.Renderer.symbols
collection. The
* default exportIcon
function is part of the exporting module.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbol
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbol
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbol
*
* @implspec symbol?: ("circle"|"diamond"|"menu"|"square"|"triangle"|"triangle-down"|"exportIcon");
*
*/
@JSProperty("symbol")
void setSymbol(Symbol value);
/**
* (Highcharts, Highstock, Highmaps) See
* navigation.buttonOptions.symbolFill.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolFill
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolFill
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolFill
*
* @implspec symbolFill?: ColorString;
*
*/
@JSProperty("symbolFill")
@Nullable
String getSymbolFill();
/**
* (Highcharts, Highstock, Highmaps) See
* navigation.buttonOptions.symbolFill.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolFill
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolFill
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolFill
*
* @implspec symbolFill?: ColorString;
*
*/
@JSProperty("symbolFill")
void setSymbolFill(String value);
/**
* (Highcharts, Highstock, Highmaps) The pixel size of the symbol on the
* button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolSize
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolSize
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolSize
*
* @implspec symbolSize?: number;
*
*/
@JSProperty("symbolSize")
double getSymbolSize();
/**
* (Highcharts, Highstock, Highmaps) The pixel size of the symbol on the
* button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolSize
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolSize
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolSize
*
* @implspec symbolSize?: number;
*
*/
@JSProperty("symbolSize")
void setSymbolSize(double value);
/**
* (Highcharts, Highstock, Highmaps) The color of the symbol's stroke or
* line.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolStroke
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolStroke
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolStroke
*
* @implspec symbolStroke?: ColorString;
*
*/
@JSProperty("symbolStroke")
@Nullable
String getSymbolStroke();
/**
* (Highcharts, Highstock, Highmaps) The color of the symbol's stroke or
* line.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolStroke
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolStroke
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolStroke
*
* @implspec symbolStroke?: ColorString;
*
*/
@JSProperty("symbolStroke")
void setSymbolStroke(String value);
/**
* (Highcharts, Highstock, Highmaps) The pixel stroke width of the symbol on
* the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolStrokeWidth
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolStrokeWidth
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolStrokeWidth
*
* @implspec symbolStrokeWidth?: number;
*
*/
@JSProperty("symbolStrokeWidth")
double getSymbolStrokeWidth();
/**
* (Highcharts, Highstock, Highmaps) The pixel stroke width of the symbol on
* the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolStrokeWidth
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolStrokeWidth
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolStrokeWidth
*
* @implspec symbolStrokeWidth?: number;
*
*/
@JSProperty("symbolStrokeWidth")
void setSymbolStrokeWidth(double value);
/**
* (Highcharts, Highstock, Highmaps) The x position of the center of the
* symbol inside the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolX
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolX
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolX
*
* @implspec symbolX?: number;
*
*/
@JSProperty("symbolX")
double getSymbolX();
/**
* (Highcharts, Highstock, Highmaps) The x position of the center of the
* symbol inside the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolX
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolX
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolX
*
* @implspec symbolX?: number;
*
*/
@JSProperty("symbolX")
void setSymbolX(double value);
/**
* (Highcharts, Highstock, Highmaps) The y position of the center of the
* symbol inside the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolY
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolY
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolY
*
* @implspec symbolY?: number;
*
*/
@JSProperty("symbolY")
double getSymbolY();
/**
* (Highcharts, Highstock, Highmaps) The y position of the center of the
* symbol inside the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.symbolY
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.symbolY
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.symbolY
*
* @implspec symbolY?: number;
*
*/
@JSProperty("symbolY")
void setSymbolY(double value);
/**
* (Highcharts, Highstock, Highmaps) A text string to add to the individual
* button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.text
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.text
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.text
*
* @implspec text?: string;
*
*/
@JSProperty("text")
@Nullable
String getText();
/**
* (Highcharts, Highstock, Highmaps) A text string to add to the individual
* button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.text
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.text
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.text
*
* @implspec text?: string;
*
*/
@JSProperty("text")
void setText(String value);
/**
* (Highcharts, Highstock, Highmaps) A configuration object for the button
* theme. The object accepts SVG properties like stroke-width
, stroke
* and fill
. Tri-state button styles are supported by the states.hover
* and states.select
objects.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.theme
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.theme
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.theme
*
* @implspec theme?: ExportingButtonsContextButtonThemeOptions;
*
*/
@JSProperty("theme")
@Nullable
ExportingButtonsContextButtonThemeOptions getTheme();
/**
* (Highcharts, Highstock, Highmaps) A configuration object for the button
* theme. The object accepts SVG properties like stroke-width
, stroke
* and fill
. Tri-state button styles are supported by the states.hover
* and states.select
objects.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.theme
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.theme
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.theme
*
* @implspec theme?: ExportingButtonsContextButtonThemeOptions;
*
*/
@JSProperty("theme")
void setTheme(ExportingButtonsContextButtonThemeOptions value);
/**
* (Highcharts, Highstock, Highmaps) The key to a lang option setting that
* is used for the button's title tooltip. When the key is
* contextButtonTitle
, it refers to lang.contextButtonTitle that defaults
* to "Chart context menu".
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.titleKey
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.titleKey
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.titleKey
*
* @implspec titleKey?: string;
*
*/
@JSProperty("titleKey")
@Nullable
String getTitleKey();
/**
* (Highcharts, Highstock, Highmaps) The key to a lang option setting that
* is used for the button's title tooltip. When the key is
* contextButtonTitle
, it refers to lang.contextButtonTitle that defaults
* to "Chart context menu".
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.titleKey
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.titleKey
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.titleKey
*
* @implspec titleKey?: string;
*
*/
@JSProperty("titleKey")
void setTitleKey(String value);
/**
* (Highcharts, Highstock, Highmaps) The vertical alignment of the buttons.
* Can be one of "top", "middle" or "bottom".
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.verticalAlign
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.verticalAlign
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.verticalAlign
*
* @implspec verticalAlign?: ("bottom"|"middle"|"top");
*
*/
@JSProperty("verticalAlign")
@Nullable
VerticalAlign getVerticalAlign();
/**
* (Highcharts, Highstock, Highmaps) The vertical alignment of the buttons.
* Can be one of "top", "middle" or "bottom".
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.verticalAlign
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.verticalAlign
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.verticalAlign
*
* @implspec verticalAlign?: ("bottom"|"middle"|"top");
*
*/
@JSProperty("verticalAlign")
void setVerticalAlign(VerticalAlign value);
/**
* (Highcharts, Highstock, Highmaps) The pixel width of the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.width
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.width
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.width
*
* @implspec width?: number;
*
*/
@JSProperty("width")
double getWidth();
/**
* (Highcharts, Highstock, Highmaps) The pixel width of the button.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.width
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.width
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.width
*
* @implspec width?: number;
*
*/
@JSProperty("width")
void setWidth(double value);
/**
* (Highcharts, Highstock, Highmaps) The horizontal position of the button
* relative to the align
option.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.x
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.x
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.x
*
* @implspec x?: number;
*
*/
@JSProperty("x")
double getX();
/**
* (Highcharts, Highstock, Highmaps) The horizontal position of the button
* relative to the align
option.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.x
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.x
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.x
*
* @implspec x?: number;
*
*/
@JSProperty("x")
void setX(double value);
/**
* (Highcharts, Highstock, Highmaps) The vertical offset of the button's
* position relative to its verticalAlign
.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.y
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.y
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.y
*
* @implspec y?: number;
*
*/
@JSProperty("y")
double getY();
/**
* (Highcharts, Highstock, Highmaps) The vertical offset of the button's
* position relative to its verticalAlign
.
*
* @see https://api.highcharts.com/highcharts/exporting.buttons.contextButton.y
* @see https://api.highcharts.com/highstock/exporting.buttons.contextButton.y
* @see https://api.highcharts.com/highmaps/exporting.buttons.contextButton.y
*
* @implspec y?: number;
*
*/
@JSProperty("y")
void setY(double value);
/**
*/
abstract class Align extends JsEnum {
public static final Align CENTER = JsEnum.of("center");
public static final Align LEFT = JsEnum.of("left");
public static final Align RIGHT = JsEnum.of("right");
}
/**
*/
@JSFunctor
@FunctionalInterface
interface Onclick extends Any {
void apply();
}
/**
*/
abstract class Symbol extends JsEnum {
public static final Symbol CIRCLE = JsEnum.of("circle");
public static final Symbol DIAMOND = JsEnum.of("diamond");
public static final Symbol MENU = JsEnum.of("menu");
public static final Symbol SQUARE = JsEnum.of("square");
public static final Symbol TRIANGLE = JsEnum.of("triangle");
public static final Symbol TRIANGLE_DOWN = JsEnum.of("triangle-down");
public static final Symbol EXPORTICON = JsEnum.of("exportIcon");
}
/**
*/
abstract class VerticalAlign extends JsEnum {
public static final VerticalAlign BOTTOM = JsEnum.of("bottom");
public static final VerticalAlign MIDDLE = JsEnum.of("middle");
public static final VerticalAlign TOP = JsEnum.of("top");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy