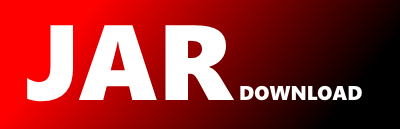
com.github.fluorumlabs.disconnect.highcharts.ExportingCsvOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import java.lang.FunctionalInterface;
import java.lang.String;
import javax.annotation.Nullable;
import js.lang.Any;
import org.teavm.jso.JSFunctor;
import org.teavm.jso.JSProperty;
/**
* (Highcharts, Highstock, Highmaps) Options for exporting data to CSV or ExCel,
* or displaying the data in a HTML table or a JavaScript structure. Requires
* the export-data.js
module. This module adds data export options to the
* export menu and provides functions like Chart.getCSV
, Chart.getTable
,
* Chart.getDataRows
and Chart.viewData
.
*
* The XLS converter is limited and only creates a HTML string that is passed
* for download, which works but creates a warning before opening. The
* workaround for this is to use a third party XLSX converter, as demonstrated
* in the sample below.
*
* @see https://api.highcharts.com/highcharts/exporting.csv
* @see https://api.highcharts.com/highstock/exporting.csv
* @see https://api.highcharts.com/highmaps/exporting.csv
*
*/
public interface ExportingCsvOptions extends Any {
/**
* (Highcharts, Highstock, Highmaps) Formatter callback for the column
* headers. Parameters are:
*
*
* -
*
item
- The series or axis object)
*
*
* -
*
key
- The point key, for example y or z
*
*
* -
*
keyLength
- The amount of value keys for this item, for example a
* range series has the keys low
and high
so the key length is 2.
*
*
*
* If useMultiLevelHeaders is true, columnHeaderFormatter by default returns
* an object with columnTitle and topLevelColumnTitle for each key. Columns
* with the same topLevelColumnTitle have their titles merged into a single
* cell with colspan for table/Excel export.
*
* If useMultiLevelHeaders
is false, or for CSV export, it returns the
* series name, followed by the key if there is more than one key.
*
* For the axis it returns the axis title or "Category" or "DateTime" by
* default.
*
* Return false
to use Highcharts' proposed header.
*
* @see https://api.highcharts.com/highcharts/exporting.csv.columnHeaderFormatter
* @see https://api.highcharts.com/highstock/exporting.csv.columnHeaderFormatter
* @see https://api.highcharts.com/highmaps/exporting.csv.columnHeaderFormatter
*
* @implspec columnHeaderFormatter?: (() => void|null);
*
*/
@JSProperty("columnHeaderFormatter")
@Nullable
ColumnHeaderFormatter getColumnHeaderFormatter();
/**
* (Highcharts, Highstock, Highmaps) Formatter callback for the column
* headers. Parameters are:
*
*
* -
*
item
- The series or axis object)
*
*
* -
*
key
- The point key, for example y or z
*
*
* -
*
keyLength
- The amount of value keys for this item, for example a
* range series has the keys low
and high
so the key length is 2.
*
*
*
* If useMultiLevelHeaders is true, columnHeaderFormatter by default returns
* an object with columnTitle and topLevelColumnTitle for each key. Columns
* with the same topLevelColumnTitle have their titles merged into a single
* cell with colspan for table/Excel export.
*
* If useMultiLevelHeaders
is false, or for CSV export, it returns the
* series name, followed by the key if there is more than one key.
*
* For the axis it returns the axis title or "Category" or "DateTime" by
* default.
*
* Return false
to use Highcharts' proposed header.
*
* @see https://api.highcharts.com/highcharts/exporting.csv.columnHeaderFormatter
* @see https://api.highcharts.com/highstock/exporting.csv.columnHeaderFormatter
* @see https://api.highcharts.com/highmaps/exporting.csv.columnHeaderFormatter
*
* @implspec columnHeaderFormatter?: (() => void|null);
*
*/
@JSProperty("columnHeaderFormatter")
void setColumnHeaderFormatter(ColumnHeaderFormatter value);
/**
* (Highcharts, Highstock, Highmaps) Which date format to use for exported
* dates on a datetime X axis. See Highcharts.dateFormat
.
*
* @see https://api.highcharts.com/highcharts/exporting.csv.dateFormat
* @see https://api.highcharts.com/highstock/exporting.csv.dateFormat
* @see https://api.highcharts.com/highmaps/exporting.csv.dateFormat
*
* @implspec dateFormat?: string;
*
*/
@JSProperty("dateFormat")
@Nullable
String getDateFormat();
/**
* (Highcharts, Highstock, Highmaps) Which date format to use for exported
* dates on a datetime X axis. See Highcharts.dateFormat
.
*
* @see https://api.highcharts.com/highcharts/exporting.csv.dateFormat
* @see https://api.highcharts.com/highstock/exporting.csv.dateFormat
* @see https://api.highcharts.com/highmaps/exporting.csv.dateFormat
*
* @implspec dateFormat?: string;
*
*/
@JSProperty("dateFormat")
void setDateFormat(String value);
/**
* (Highcharts, Highstock, Highmaps) Which decimal point to use for exported
* CSV. Defaults to the same as the browser locale, typically .
(English)
* or ,
(German, French etc).
*
* @see https://api.highcharts.com/highcharts/exporting.csv.decimalPoint
* @see https://api.highcharts.com/highstock/exporting.csv.decimalPoint
* @see https://api.highcharts.com/highmaps/exporting.csv.decimalPoint
*
* @implspec decimalPoint?: (string|null);
*
*/
@JSProperty("decimalPoint")
@Nullable
String getDecimalPoint();
/**
* (Highcharts, Highstock, Highmaps) Which decimal point to use for exported
* CSV. Defaults to the same as the browser locale, typically .
(English)
* or ,
(German, French etc).
*
* @see https://api.highcharts.com/highcharts/exporting.csv.decimalPoint
* @see https://api.highcharts.com/highstock/exporting.csv.decimalPoint
* @see https://api.highcharts.com/highmaps/exporting.csv.decimalPoint
*
* @implspec decimalPoint?: (string|null);
*
*/
@JSProperty("decimalPoint")
void setDecimalPoint(String value);
/**
* (Highcharts, Highstock, Highmaps) The item delimiter in the exported
* data. Use ;
for direct exporting to Excel. Defaults to a best guess
* based on the browser locale. If the locale decimal point is ,
, the
* itemDelimiter
defaults to ;
, otherwise the itemDelimiter
defaults
* to ,
.
*
* @see https://api.highcharts.com/highcharts/exporting.csv.itemDelimiter
* @see https://api.highcharts.com/highstock/exporting.csv.itemDelimiter
* @see https://api.highcharts.com/highmaps/exporting.csv.itemDelimiter
*
* @implspec itemDelimiter?: (string|null);
*
*/
@JSProperty("itemDelimiter")
@Nullable
String getItemDelimiter();
/**
* (Highcharts, Highstock, Highmaps) The item delimiter in the exported
* data. Use ;
for direct exporting to Excel. Defaults to a best guess
* based on the browser locale. If the locale decimal point is ,
, the
* itemDelimiter
defaults to ;
, otherwise the itemDelimiter
defaults
* to ,
.
*
* @see https://api.highcharts.com/highcharts/exporting.csv.itemDelimiter
* @see https://api.highcharts.com/highstock/exporting.csv.itemDelimiter
* @see https://api.highcharts.com/highmaps/exporting.csv.itemDelimiter
*
* @implspec itemDelimiter?: (string|null);
*
*/
@JSProperty("itemDelimiter")
void setItemDelimiter(String value);
/**
* (Highcharts, Highstock, Highmaps) The line delimiter in the exported
* data, defaults to a newline.
*
* @see https://api.highcharts.com/highcharts/exporting.csv.lineDelimiter
* @see https://api.highcharts.com/highstock/exporting.csv.lineDelimiter
* @see https://api.highcharts.com/highmaps/exporting.csv.lineDelimiter
*
* @implspec lineDelimiter?: string;
*
*/
@JSProperty("lineDelimiter")
@Nullable
String getLineDelimiter();
/**
* (Highcharts, Highstock, Highmaps) The line delimiter in the exported
* data, defaults to a newline.
*
* @see https://api.highcharts.com/highcharts/exporting.csv.lineDelimiter
* @see https://api.highcharts.com/highstock/exporting.csv.lineDelimiter
* @see https://api.highcharts.com/highmaps/exporting.csv.lineDelimiter
*
* @implspec lineDelimiter?: string;
*
*/
@JSProperty("lineDelimiter")
void setLineDelimiter(String value);
/**
*/
@JSFunctor
@FunctionalInterface
interface ColumnHeaderFormatter extends Any {
void apply();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy