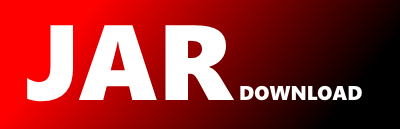
com.github.fluorumlabs.disconnect.highcharts.PlotOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import js.lang.Any;
import org.teavm.jso.JSProperty;
import javax.annotation.Nullable;
/**
* (Highcharts, Highstock, Highmaps) The plotOptions is a wrapper object for
* config objects for each series type. The config objects for each series can
* also be overridden for each series item as given in the series array.
*
* Configuration options for the series are given in three levels. Options for
* all series in a chart are given in the plotOptions.series object. Then
* options for all series of a specific type are given in the plotOptions of
* that type, for example plotOptions.line
. Next, options for one single
* series are given in the series array.
*
* @see https://api.highcharts.com/highcharts/plotOptions
* @see https://api.highcharts.com/highstock/plotOptions
* @see https://api.highcharts.com/highmaps/plotOptions
*
*/
public interface PlotOptions extends Any {
/**
* (Highstock) Accumulation Distribution (AD). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
ad
series are defined in plotOptions.ad.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.ad
*
* @implspec ad?: PlotAdOptions;
*
*/
@JSProperty("ad")
@Nullable
PlotAdOptions getAd();
/**
* (Highstock) Accumulation Distribution (AD). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
ad
series are defined in plotOptions.ad.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.ad
*
* @implspec ad?: PlotAdOptions;
*
*/
@JSProperty("ad")
void setAd(PlotAdOptions value);
/**
* (Highcharts, Highstock) The area series type.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
area
series are defined in plotOptions.area.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.area
* @see https://api.highcharts.com/highstock/plotOptions.area
*
* @implspec area?: PlotAreaOptions;
*
*/
@JSProperty("area")
@Nullable
PlotAreaOptions getArea();
/**
* (Highcharts, Highstock) The area series type.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
area
series are defined in plotOptions.area.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.area
* @see https://api.highcharts.com/highstock/plotOptions.area
*
* @implspec area?: PlotAreaOptions;
*
*/
@JSProperty("area")
void setArea(PlotAreaOptions value);
/**
* (Highcharts, Highstock) The area range series is a carteseian series with
* higher and lower values for each point along an X axis, where the area
* between the values is shaded. Requires highcharts-more.js
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
arearange
series are defined in
* plotOptions.arearange.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.arearange
* @see https://api.highcharts.com/highstock/plotOptions.arearange
*
* @implspec arearange?: PlotArearangeOptions;
*
*/
@JSProperty("arearange")
@Nullable
PlotArearangeOptions getArearange();
/**
* (Highcharts, Highstock) The area range series is a carteseian series with
* higher and lower values for each point along an X axis, where the area
* between the values is shaded. Requires highcharts-more.js
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
arearange
series are defined in
* plotOptions.arearange.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.arearange
* @see https://api.highcharts.com/highstock/plotOptions.arearange
*
* @implspec arearange?: PlotArearangeOptions;
*
*/
@JSProperty("arearange")
void setArearange(PlotArearangeOptions value);
/**
* (Highcharts, Highstock) The area spline series is an area series where
* the graph between the points is smoothed into a spline.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
areaspline
series are defined in
* plotOptions.areaspline.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.areaspline
* @see https://api.highcharts.com/highstock/plotOptions.areaspline
*
* @implspec areaspline?: PlotAreasplineOptions;
*
*/
@JSProperty("areaspline")
@Nullable
PlotAreasplineOptions getAreaspline();
/**
* (Highcharts, Highstock) The area spline series is an area series where
* the graph between the points is smoothed into a spline.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
areaspline
series are defined in
* plotOptions.areaspline.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.areaspline
* @see https://api.highcharts.com/highstock/plotOptions.areaspline
*
* @implspec areaspline?: PlotAreasplineOptions;
*
*/
@JSProperty("areaspline")
void setAreaspline(PlotAreasplineOptions value);
/**
* (Highcharts, Highstock) The area spline range is a cartesian series type
* with higher and lower Y values along an X axis. The area inside the range
* is colored, and the graph outlining the area is a smoothed spline.
* Requires highcharts-more.js
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
areasplinerange
series are defined in
* plotOptions.areasplinerange.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.areasplinerange
* @see https://api.highcharts.com/highstock/plotOptions.areasplinerange
*
* @implspec areasplinerange?: PlotAreasplinerangeOptions;
*
*/
@JSProperty("areasplinerange")
@Nullable
PlotAreasplinerangeOptions getAreasplinerange();
/**
* (Highcharts, Highstock) The area spline range is a cartesian series type
* with higher and lower Y values along an X axis. The area inside the range
* is colored, and the graph outlining the area is a smoothed spline.
* Requires highcharts-more.js
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
areasplinerange
series are defined in
* plotOptions.areasplinerange.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.areasplinerange
* @see https://api.highcharts.com/highstock/plotOptions.areasplinerange
*
* @implspec areasplinerange?: PlotAreasplinerangeOptions;
*
*/
@JSProperty("areasplinerange")
void setAreasplinerange(PlotAreasplinerangeOptions value);
/**
* (Highstock) Average true range indicator (ATR). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
atr
series are defined in plotOptions.atr.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.atr
*
* @implspec atr?: PlotAtrOptions;
*
*/
@JSProperty("atr")
@Nullable
PlotAtrOptions getAtr();
/**
* (Highstock) Average true range indicator (ATR). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
atr
series are defined in plotOptions.atr.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.atr
*
* @implspec atr?: PlotAtrOptions;
*
*/
@JSProperty("atr")
void setAtr(PlotAtrOptions value);
/**
* (Highcharts) A bar series is a special type of column series where the
* columns are horizontal.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bar
series are defined in plotOptions.bar.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.bar
*
* @implspec bar?: PlotBarOptions;
*
*/
@JSProperty("bar")
@Nullable
PlotBarOptions getBar();
/**
* (Highcharts) A bar series is a special type of column series where the
* columns are horizontal.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bar
series are defined in plotOptions.bar.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.bar
*
* @implspec bar?: PlotBarOptions;
*
*/
@JSProperty("bar")
void setBar(PlotBarOptions value);
/**
* (Highstock) Bollinger bands (BB). This series requires the linkedTo
* option to be set and should be loaded after the
* stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bb
series are defined in plotOptions.bb.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.bb
*
* @implspec bb?: PlotBbOptions;
*
*/
@JSProperty("bb")
@Nullable
PlotBbOptions getBb();
/**
* (Highstock) Bollinger bands (BB). This series requires the linkedTo
* option to be set and should be loaded after the
* stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bb
series are defined in plotOptions.bb.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.bb
*
* @implspec bb?: PlotBbOptions;
*
*/
@JSProperty("bb")
void setBb(PlotBbOptions value);
/**
* (Highcharts) A bell curve is an areaspline series which represents the
* probability density function of the normal distribution. It calculates
* mean and standard deviation of the base series data and plots the curve
* according to the calculated parameters.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bellcurve
series are defined in
* plotOptions.bellcurve.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.bellcurve
*
* @implspec bellcurve?: PlotBellcurveOptions;
*
*/
@JSProperty("bellcurve")
@Nullable
PlotBellcurveOptions getBellcurve();
/**
* (Highcharts) A bell curve is an areaspline series which represents the
* probability density function of the normal distribution. It calculates
* mean and standard deviation of the base series data and plots the curve
* according to the calculated parameters.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bellcurve
series are defined in
* plotOptions.bellcurve.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.bellcurve
*
* @implspec bellcurve?: PlotBellcurveOptions;
*
*/
@JSProperty("bellcurve")
void setBellcurve(PlotBellcurveOptions value);
/**
* (Highcharts) A box plot is a convenient way of depicting groups of data
* through their five-number summaries: the smallest observation (sample
* minimum), lower quartile (Q1), median (Q2), upper quartile (Q3), and
* largest observation (sample maximum).
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
boxplot
series are defined in plotOptions.boxplot.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.boxplot
*
* @implspec boxplot?: PlotBoxplotOptions;
*
*/
@JSProperty("boxplot")
@Nullable
PlotBoxplotOptions getBoxplot();
/**
* (Highcharts) A box plot is a convenient way of depicting groups of data
* through their five-number summaries: the smallest observation (sample
* minimum), lower quartile (Q1), median (Q2), upper quartile (Q3), and
* largest observation (sample maximum).
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
boxplot
series are defined in plotOptions.boxplot.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.boxplot
*
* @implspec boxplot?: PlotBoxplotOptions;
*
*/
@JSProperty("boxplot")
void setBoxplot(PlotBoxplotOptions value);
/**
* (Highcharts, Highstock) A bubble series is a three dimensional series
* type where each point renders an X, Y and Z value. Each points is drawn
* as a bubble where the position along the X and Y axes mark the X and Y
* values, and the size of the bubble relates to the Z value. Requires
* highcharts-more.js
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bubble
series are defined in plotOptions.bubble.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.bubble
* @see https://api.highcharts.com/highstock/plotOptions.bubble
*
* @implspec bubble?: PlotBubbleOptions;
*
*/
@JSProperty("bubble")
@Nullable
PlotBubbleOptions getBubble();
/**
* (Highcharts, Highstock) A bubble series is a three dimensional series
* type where each point renders an X, Y and Z value. Each points is drawn
* as a bubble where the position along the X and Y axes mark the X and Y
* values, and the size of the bubble relates to the Z value. Requires
* highcharts-more.js
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bubble
series are defined in plotOptions.bubble.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.bubble
* @see https://api.highcharts.com/highstock/plotOptions.bubble
*
* @implspec bubble?: PlotBubbleOptions;
*
*/
@JSProperty("bubble")
void setBubble(PlotBubbleOptions value);
/**
* (Highcharts) A bullet graph is a variation of a bar graph. The bullet
* graph features a single measure, compares it to a target, and displays it
* in the context of qualitative ranges of performance that could be set
* using plotBands on yAxis.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bullet
series are defined in plotOptions.bullet.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.bullet
*
* @implspec bullet?: PlotBulletOptions;
*
*/
@JSProperty("bullet")
@Nullable
PlotBulletOptions getBullet();
/**
* (Highcharts) A bullet graph is a variation of a bar graph. The bullet
* graph features a single measure, compares it to a target, and displays it
* in the context of qualitative ranges of performance that could be set
* using plotBands on yAxis.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
bullet
series are defined in plotOptions.bullet.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.bullet
*
* @implspec bullet?: PlotBulletOptions;
*
*/
@JSProperty("bullet")
void setBullet(PlotBulletOptions value);
/**
* (Highstock) A candlestick chart is a style of financial chart used to
* describe price movements over time.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
candlestick
series are defined in
* plotOptions.candlestick.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.candlestick
*
* @implspec candlestick?: PlotCandlestickOptions;
*
*/
@JSProperty("candlestick")
@Nullable
PlotCandlestickOptions getCandlestick();
/**
* (Highstock) A candlestick chart is a style of financial chart used to
* describe price movements over time.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
candlestick
series are defined in
* plotOptions.candlestick.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.candlestick
*
* @implspec candlestick?: PlotCandlestickOptions;
*
*/
@JSProperty("candlestick")
void setCandlestick(PlotCandlestickOptions value);
/**
* (Highstock) Commodity Channel Index (CCI). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
cci
series are defined in plotOptions.cci.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.cci
*
* @implspec cci?: PlotCciOptions;
*
*/
@JSProperty("cci")
@Nullable
PlotCciOptions getCci();
/**
* (Highstock) Commodity Channel Index (CCI). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
cci
series are defined in plotOptions.cci.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.cci
*
* @implspec cci?: PlotCciOptions;
*
*/
@JSProperty("cci")
void setCci(PlotCciOptions value);
/**
* (Highstock) Chaikin Money Flow indicator (cmf).
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
cmf
series are defined in plotOptions.cmf.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.cmf
*
* @implspec cmf?: PlotCmfOptions;
*
*/
@JSProperty("cmf")
@Nullable
PlotCmfOptions getCmf();
/**
* (Highstock) Chaikin Money Flow indicator (cmf).
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
cmf
series are defined in plotOptions.cmf.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.cmf
*
* @implspec cmf?: PlotCmfOptions;
*
*/
@JSProperty("cmf")
void setCmf(PlotCmfOptions value);
/**
* (Highcharts, Highstock) Column series display one column per value along
* an X axis.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
column
series are defined in plotOptions.column.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.column
* @see https://api.highcharts.com/highstock/plotOptions.column
*
* @implspec column?: PlotColumnOptions;
*
*/
@JSProperty("column")
@Nullable
PlotColumnOptions getColumn();
/**
* (Highcharts, Highstock) Column series display one column per value along
* an X axis.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
column
series are defined in plotOptions.column.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.column
* @see https://api.highcharts.com/highstock/plotOptions.column
*
* @implspec column?: PlotColumnOptions;
*
*/
@JSProperty("column")
void setColumn(PlotColumnOptions value);
/**
* (Highcharts, Highstock) The column range is a cartesian series type with
* higher and lower Y values along an X axis. Requires highcharts-more.js
.
* To display horizontal bars, set chart.inverted to true
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
columnrange
series are defined in
* plotOptions.columnrange.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.columnrange
* @see https://api.highcharts.com/highstock/plotOptions.columnrange
*
* @implspec columnrange?: PlotColumnrangeOptions;
*
*/
@JSProperty("columnrange")
@Nullable
PlotColumnrangeOptions getColumnrange();
/**
* (Highcharts, Highstock) The column range is a cartesian series type with
* higher and lower Y values along an X axis. Requires highcharts-more.js
.
* To display horizontal bars, set chart.inverted to true
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
columnrange
series are defined in
* plotOptions.columnrange.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.columnrange
* @see https://api.highcharts.com/highstock/plotOptions.columnrange
*
* @implspec columnrange?: PlotColumnrangeOptions;
*
*/
@JSProperty("columnrange")
void setColumnrange(PlotColumnrangeOptions value);
/**
* (Highstock) Exponential moving average indicator (EMA). This series
* requires the linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
ema
series are defined in plotOptions.ema.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.ema
*
* @implspec ema?: PlotEmaOptions;
*
*/
@JSProperty("ema")
@Nullable
PlotEmaOptions getEma();
/**
* (Highstock) Exponential moving average indicator (EMA). This series
* requires the linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
ema
series are defined in plotOptions.ema.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.ema
*
* @implspec ema?: PlotEmaOptions;
*
*/
@JSProperty("ema")
void setEma(PlotEmaOptions value);
/**
* (Highcharts, Highstock) Error bars are a graphical representation of the
* variability of data and are used on graphs to indicate the error, or
* uncertainty in a reported measurement.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
errorbar
series are defined in plotOptions.errorbar.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.errorbar
* @see https://api.highcharts.com/highstock/plotOptions.errorbar
*
* @implspec errorbar?: PlotErrorbarOptions;
*
*/
@JSProperty("errorbar")
@Nullable
PlotErrorbarOptions getErrorbar();
/**
* (Highcharts, Highstock) Error bars are a graphical representation of the
* variability of data and are used on graphs to indicate the error, or
* uncertainty in a reported measurement.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
errorbar
series are defined in plotOptions.errorbar.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.errorbar
* @see https://api.highcharts.com/highstock/plotOptions.errorbar
*
* @implspec errorbar?: PlotErrorbarOptions;
*
*/
@JSProperty("errorbar")
void setErrorbar(PlotErrorbarOptions value);
/**
* (Highstock) Flags are used to mark events in stock charts. They can be
* added on the timeline, or attached to a specific series.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
flags
series are defined in plotOptions.flags.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.flags
*
* @implspec flags?: PlotFlagsOptions;
*
*/
@JSProperty("flags")
@Nullable
PlotFlagsOptions getFlags();
/**
* (Highstock) Flags are used to mark events in stock charts. They can be
* added on the timeline, or attached to a specific series.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
flags
series are defined in plotOptions.flags.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.flags
*
* @implspec flags?: PlotFlagsOptions;
*
*/
@JSProperty("flags")
void setFlags(PlotFlagsOptions value);
/**
* (Highcharts) Funnel charts are a type of chart often used to visualize
* stages in a sales project, where the top are the initial stages with the
* most clients. It requires that the modules/funnel.js file is loaded.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
funnel
series are defined in plotOptions.funnel.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.funnel
*
* @implspec funnel?: PlotFunnelOptions;
*
*/
@JSProperty("funnel")
@Nullable
PlotFunnelOptions getFunnel();
/**
* (Highcharts) Funnel charts are a type of chart often used to visualize
* stages in a sales project, where the top are the initial stages with the
* most clients. It requires that the modules/funnel.js file is loaded.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
funnel
series are defined in plotOptions.funnel.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.funnel
*
* @implspec funnel?: PlotFunnelOptions;
*
*/
@JSProperty("funnel")
void setFunnel(PlotFunnelOptions value);
/**
* (Gantt) A gantt
series. If the type option is not specified, it is
* inherited from chart.type.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
gantt
series are defined in plotOptions.gantt.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/gantt/plotOptions.gantt
*
* @implspec gantt?: PlotGanttOptions;
*
*/
@JSProperty("gantt")
@Nullable
PlotGanttOptions getGantt();
/**
* (Gantt) A gantt
series. If the type option is not specified, it is
* inherited from chart.type.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
gantt
series are defined in plotOptions.gantt.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/gantt/plotOptions.gantt
*
* @implspec gantt?: PlotGanttOptions;
*
*/
@JSProperty("gantt")
void setGantt(PlotGanttOptions value);
/**
* (Highcharts) Gauges are circular plots displaying one or more values with
* a dial pointing to values along the perimeter.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
gauge
series are defined in plotOptions.gauge.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.gauge
*
* @implspec gauge?: PlotGaugeOptions;
*
*/
@JSProperty("gauge")
@Nullable
PlotGaugeOptions getGauge();
/**
* (Highcharts) Gauges are circular plots displaying one or more values with
* a dial pointing to values along the perimeter.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
gauge
series are defined in plotOptions.gauge.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.gauge
*
* @implspec gauge?: PlotGaugeOptions;
*
*/
@JSProperty("gauge")
void setGauge(PlotGaugeOptions value);
/**
* (Highcharts, Highmaps) A heatmap is a graphical representation of data
* where the individual values contained in a matrix are represented as
* colors.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
heatmap
series are defined in plotOptions.heatmap.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.heatmap
* @see https://api.highcharts.com/highmaps/plotOptions.heatmap
*
* @implspec heatmap?: PlotHeatmapOptions;
*
*/
@JSProperty("heatmap")
@Nullable
PlotHeatmapOptions getHeatmap();
/**
* (Highcharts, Highmaps) A heatmap is a graphical representation of data
* where the individual values contained in a matrix are represented as
* colors.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
heatmap
series are defined in plotOptions.heatmap.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.heatmap
* @see https://api.highcharts.com/highmaps/plotOptions.heatmap
*
* @implspec heatmap?: PlotHeatmapOptions;
*
*/
@JSProperty("heatmap")
void setHeatmap(PlotHeatmapOptions value);
/**
* (Highcharts) A histogram is a column series which represents the
* distribution of the data set in the base series. Histogram splits data
* into bins and shows their frequencies.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
histogram
series are defined in
* plotOptions.histogram.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.histogram
*
* @implspec histogram?: PlotHistogramOptions;
*
*/
@JSProperty("histogram")
@Nullable
PlotHistogramOptions getHistogram();
/**
* (Highcharts) A histogram is a column series which represents the
* distribution of the data set in the base series. Histogram splits data
* into bins and shows their frequencies.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
histogram
series are defined in
* plotOptions.histogram.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.histogram
*
* @implspec histogram?: PlotHistogramOptions;
*
*/
@JSProperty("histogram")
void setHistogram(PlotHistogramOptions value);
/**
* (Highstock) Ichimoku Kinko Hyo (IKH). This series requires linkedTo
* option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
ikh
series are defined in plotOptions.ikh.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.ikh
*
* @implspec ikh?: PlotIkhOptions;
*
*/
@JSProperty("ikh")
@Nullable
PlotIkhOptions getIkh();
/**
* (Highstock) Ichimoku Kinko Hyo (IKH). This series requires linkedTo
* option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
ikh
series are defined in plotOptions.ikh.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.ikh
*
* @implspec ikh?: PlotIkhOptions;
*
*/
@JSProperty("ikh")
void setIkh(PlotIkhOptions value);
/**
* (Highcharts, Highstock) A line series displays information as a series of
* data points connected by straight line segments.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
line
series are defined in plotOptions.line.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.line
* @see https://api.highcharts.com/highstock/plotOptions.line
*
* @implspec line?: PlotLineOptions;
*
*/
@JSProperty("line")
@Nullable
PlotLineOptions getLine();
/**
* (Highcharts, Highstock) A line series displays information as a series of
* data points connected by straight line segments.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
line
series are defined in plotOptions.line.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.line
* @see https://api.highcharts.com/highstock/plotOptions.line
*
* @implspec line?: PlotLineOptions;
*
*/
@JSProperty("line")
void setLine(PlotLineOptions value);
/**
* (Highstock) Moving Average Convergence Divergence (MACD). This series
* requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
macd
series are defined in plotOptions.macd.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.macd
*
* @implspec macd?: PlotMacdOptions;
*
*/
@JSProperty("macd")
@Nullable
PlotMacdOptions getMacd();
/**
* (Highstock) Moving Average Convergence Divergence (MACD). This series
* requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
macd
series are defined in plotOptions.macd.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.macd
*
* @implspec macd?: PlotMacdOptions;
*
*/
@JSProperty("macd")
void setMacd(PlotMacdOptions value);
/**
* (Highmaps) The map series is used for basic choropleth maps, where each
* map area has a color based on its value.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
map
series are defined in plotOptions.map.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highmaps/plotOptions.map
*
* @implspec map?: PlotMapOptions;
*
*/
@JSProperty("map")
@Nullable
PlotMapOptions getMap();
/**
* (Highmaps) The map series is used for basic choropleth maps, where each
* map area has a color based on its value.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
map
series are defined in plotOptions.map.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highmaps/plotOptions.map
*
* @implspec map?: PlotMapOptions;
*
*/
@JSProperty("map")
void setMap(PlotMapOptions value);
/**
* (Highmaps) A map bubble series is a bubble series laid out on top of a
* map series, where each bubble is tied to a specific map area.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
mapbubble
series are defined in
* plotOptions.mapbubble.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highmaps/plotOptions.mapbubble
*
* @implspec mapbubble?: PlotMapbubbleOptions;
*
*/
@JSProperty("mapbubble")
@Nullable
PlotMapbubbleOptions getMapbubble();
/**
* (Highmaps) A map bubble series is a bubble series laid out on top of a
* map series, where each bubble is tied to a specific map area.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
mapbubble
series are defined in
* plotOptions.mapbubble.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highmaps/plotOptions.mapbubble
*
* @implspec mapbubble?: PlotMapbubbleOptions;
*
*/
@JSProperty("mapbubble")
void setMapbubble(PlotMapbubbleOptions value);
/**
* (Highmaps) A mapline series is a special case of the map series where the
* value colors are applied to the strokes rather than the fills. It can
* also be used for freeform drawing, like dividers, in the map.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
mapline
series are defined in plotOptions.mapline.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highmaps/plotOptions.mapline
*
* @implspec mapline?: PlotMaplineOptions;
*
*/
@JSProperty("mapline")
@Nullable
PlotMaplineOptions getMapline();
/**
* (Highmaps) A mapline series is a special case of the map series where the
* value colors are applied to the strokes rather than the fills. It can
* also be used for freeform drawing, like dividers, in the map.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
mapline
series are defined in plotOptions.mapline.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highmaps/plotOptions.mapline
*
* @implspec mapline?: PlotMaplineOptions;
*
*/
@JSProperty("mapline")
void setMapline(PlotMaplineOptions value);
/**
* (Highmaps) A mappoint series is a special form of scatter series where
* the points can be laid out in map coordinates on top of a map.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
mappoint
series are defined in plotOptions.mappoint.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highmaps/plotOptions.mappoint
*
* @implspec mappoint?: PlotMappointOptions;
*
*/
@JSProperty("mappoint")
@Nullable
PlotMapPointOptions getMappoint();
/**
* (Highmaps) A mappoint series is a special form of scatter series where
* the points can be laid out in map coordinates on top of a map.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
mappoint
series are defined in plotOptions.mappoint.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highmaps/plotOptions.mappoint
*
* @implspec mappoint?: PlotMappointOptions;
*
*/
@JSProperty("mappoint")
void setMappoint(PlotMapPointOptions value);
/**
* (Highstock) Money Flow Index. This series requires linkedTo
option to
* be set and should be loaded after the stock/indicators/indicators.js
* file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
mfi
series are defined in plotOptions.mfi.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.mfi
*
* @implspec mfi?: PlotMfiOptions;
*
*/
@JSProperty("mfi")
@Nullable
PlotMfiOptions getMfi();
/**
* (Highstock) Money Flow Index. This series requires linkedTo
option to
* be set and should be loaded after the stock/indicators/indicators.js
* file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
mfi
series are defined in plotOptions.mfi.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.mfi
*
* @implspec mfi?: PlotMfiOptions;
*
*/
@JSProperty("mfi")
void setMfi(PlotMfiOptions value);
/**
* (Highstock) Momentum. This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
momentum
series are defined in plotOptions.momentum.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.momentum
*
* @implspec momentum?: PlotMomentumOptions;
*
*/
@JSProperty("momentum")
@Nullable
PlotMomentumOptions getMomentum();
/**
* (Highstock) Momentum. This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
momentum
series are defined in plotOptions.momentum.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.momentum
*
* @implspec momentum?: PlotMomentumOptions;
*
*/
@JSProperty("momentum")
void setMomentum(PlotMomentumOptions value);
/**
* (Highstock) An OHLC chart is a style of financial chart used to describe
* price movements over time. It displays open, high, low and close values
* per data point.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
ohlc
series are defined in plotOptions.ohlc.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.ohlc
*
* @implspec ohlc?: PlotOhlcOptions;
*
*/
@JSProperty("ohlc")
@Nullable
PlotOhlcOptions getOhlc();
/**
* (Highstock) An OHLC chart is a style of financial chart used to describe
* price movements over time. It displays open, high, low and close values
* per data point.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
ohlc
series are defined in plotOptions.ohlc.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.ohlc
*
* @implspec ohlc?: PlotOhlcOptions;
*
*/
@JSProperty("ohlc")
void setOhlc(PlotOhlcOptions value);
/**
* (Highcharts) A pareto diagram is a type of chart that contains both bars
* and a line graph, where individual values are represented in descending
* order by bars, and the cumulative total is represented by the line.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
pareto
series are defined in plotOptions.pareto.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.pareto
*
* @implspec pareto?: PlotParetoOptions;
*
*/
@JSProperty("pareto")
@Nullable
PlotParetoOptions getPareto();
/**
* (Highcharts) A pareto diagram is a type of chart that contains both bars
* and a line graph, where individual values are represented in descending
* order by bars, and the cumulative total is represented by the line.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
pareto
series are defined in plotOptions.pareto.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.pareto
*
* @implspec pareto?: PlotParetoOptions;
*
*/
@JSProperty("pareto")
void setPareto(PlotParetoOptions value);
/**
* (Highcharts) A pie chart is a circular graphic which is divided into
* slices to illustrate numerical proportion.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
pie
series are defined in plotOptions.pie.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.pie
*
* @implspec pie?: PlotPieOptions;
*
*/
@JSProperty("pie")
@Nullable
PlotPieOptions getPie();
/**
* (Highcharts) A pie chart is a circular graphic which is divided into
* slices to illustrate numerical proportion.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
pie
series are defined in plotOptions.pie.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.pie
*
* @implspec pie?: PlotPieOptions;
*
*/
@JSProperty("pie")
void setPie(PlotPieOptions value);
/**
* (Highstock) Pivot points indicator. This series requires the linkedTo
* option to be set and should be loaded after
* stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
pivotpoints
series are defined in
* plotOptions.pivotpoints.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.pivotpoints
*
* @implspec pivotpoints?: PlotPivotpointsOptions;
*
*/
@JSProperty("pivotpoints")
@Nullable
PlotPivotpointsOptions getPivotpoints();
/**
* (Highstock) Pivot points indicator. This series requires the linkedTo
* option to be set and should be loaded after
* stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
pivotpoints
series are defined in
* plotOptions.pivotpoints.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.pivotpoints
*
* @implspec pivotpoints?: PlotPivotpointsOptions;
*
*/
@JSProperty("pivotpoints")
void setPivotpoints(PlotPivotpointsOptions value);
/**
* (Highcharts, Highstock) A polygon series can be used to draw any freeform
* shape in the cartesian coordinate system. A fill is applied with the
* color
option, and stroke is applied through lineWidth
and lineColor
* options. Requires the highcharts-more.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
polygon
series are defined in plotOptions.polygon.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.polygon
* @see https://api.highcharts.com/highstock/plotOptions.polygon
*
* @implspec polygon?: PlotPolygonOptions;
*
*/
@JSProperty("polygon")
@Nullable
PlotPolygonOptions getPolygon();
/**
* (Highcharts, Highstock) A polygon series can be used to draw any freeform
* shape in the cartesian coordinate system. A fill is applied with the
* color
option, and stroke is applied through lineWidth
and lineColor
* options. Requires the highcharts-more.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
polygon
series are defined in plotOptions.polygon.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.polygon
* @see https://api.highcharts.com/highstock/plotOptions.polygon
*
* @implspec polygon?: PlotPolygonOptions;
*
*/
@JSProperty("polygon")
void setPolygon(PlotPolygonOptions value);
/**
* (Highstock) Price envelopes indicator based on SMA calculations. This
* series requires the linkedTo
option to be set and should be loaded
* after the stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
priceenvelopes
series are defined in
* plotOptions.priceenvelopes.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.priceenvelopes
*
* @implspec priceenvelopes?: PlotPriceenvelopesOptions;
*
*/
@JSProperty("priceenvelopes")
@Nullable
PlotPriceenvelopesOptions getPriceenvelopes();
/**
* (Highstock) Price envelopes indicator based on SMA calculations. This
* series requires the linkedTo
option to be set and should be loaded
* after the stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
priceenvelopes
series are defined in
* plotOptions.priceenvelopes.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.priceenvelopes
*
* @implspec priceenvelopes?: PlotPriceenvelopesOptions;
*
*/
@JSProperty("priceenvelopes")
void setPriceenvelopes(PlotPriceenvelopesOptions value);
/**
* (Highstock) Parabolic SAR. This series requires linkedTo
option to be
* set and should be loaded after stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
psar
series are defined in plotOptions.psar.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.psar
*
* @implspec psar?: PlotPsarOptions;
*
*/
@JSProperty("psar")
@Nullable
PlotPsarOptions getPsar();
/**
* (Highstock) Parabolic SAR. This series requires linkedTo
option to be
* set and should be loaded after stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
psar
series are defined in plotOptions.psar.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.psar
*
* @implspec psar?: PlotPsarOptions;
*
*/
@JSProperty("psar")
void setPsar(PlotPsarOptions value);
/**
* (Highcharts) A pyramid series is a special type of funnel, without neck
* and reversed by default. Requires the funnel module.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
pyramid
series are defined in plotOptions.pyramid.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.pyramid
*
* @implspec pyramid?: PlotPyramidOptions;
*
*/
@JSProperty("pyramid")
@Nullable
PlotPyramidOptions getPyramid();
/**
* (Highcharts) A pyramid series is a special type of funnel, without neck
* and reversed by default. Requires the funnel module.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
pyramid
series are defined in plotOptions.pyramid.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.pyramid
*
* @implspec pyramid?: PlotPyramidOptions;
*
*/
@JSProperty("pyramid")
void setPyramid(PlotPyramidOptions value);
/**
* (Highstock) Rate of change indicator (ROC). The indicator value for each
* point is defined as:
*
* (C - Cn) / Cn * 100
*
* where: C
is the close value of the point of the same x in the linked
* series and Cn
is the close value of the point n
periods ago. n
is
* set through period.
*
* This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
roc
series are defined in plotOptions.roc.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.roc
*
* @implspec roc?: PlotRocOptions;
*
*/
@JSProperty("roc")
@Nullable
PlotRocOptions getRoc();
/**
* (Highstock) Rate of change indicator (ROC). The indicator value for each
* point is defined as:
*
* (C - Cn) / Cn * 100
*
* where: C
is the close value of the point of the same x in the linked
* series and Cn
is the close value of the point n
periods ago. n
is
* set through period.
*
* This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
roc
series are defined in plotOptions.roc.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.roc
*
* @implspec roc?: PlotRocOptions;
*
*/
@JSProperty("roc")
void setRoc(PlotRocOptions value);
/**
* (Highstock) Relative strength index (RSI) technical indicator. This
* series requires the linkedTo
option to be set and should be loaded
* after the stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
rsi
series are defined in plotOptions.rsi.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.rsi
*
* @implspec rsi?: PlotRsiOptions;
*
*/
@JSProperty("rsi")
@Nullable
PlotRsiOptions getRsi();
/**
* (Highstock) Relative strength index (RSI) technical indicator. This
* series requires the linkedTo
option to be set and should be loaded
* after the stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
rsi
series are defined in plotOptions.rsi.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.rsi
*
* @implspec rsi?: PlotRsiOptions;
*
*/
@JSProperty("rsi")
void setRsi(PlotRsiOptions value);
/**
* (Highcharts) A sankey diagram is a type of flow diagram, in which the
* width of the link between two nodes is shown proportionally to the flow
* quantity.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
sankey
series are defined in plotOptions.sankey.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.sankey
*
* @implspec sankey?: PlotSankeyOptions;
*
*/
@JSProperty("sankey")
@Nullable
PlotSankeyOptions getSankey();
/**
* (Highcharts) A sankey diagram is a type of flow diagram, in which the
* width of the link between two nodes is shown proportionally to the flow
* quantity.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
sankey
series are defined in plotOptions.sankey.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.sankey
*
* @implspec sankey?: PlotSankeyOptions;
*
*/
@JSProperty("sankey")
void setSankey(PlotSankeyOptions value);
/**
* (Highcharts, Highstock) A scatter plot uses cartesian coordinates to
* display values for two variables for a set of data.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
scatter
series are defined in plotOptions.scatter.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.scatter
* @see https://api.highcharts.com/highstock/plotOptions.scatter
*
* @implspec scatter?: PlotScatterOptions;
*
*/
@JSProperty("scatter")
@Nullable
PlotScatterOptions getScatter();
/**
* (Highcharts, Highstock) A scatter plot uses cartesian coordinates to
* display values for two variables for a set of data.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
scatter
series are defined in plotOptions.scatter.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.scatter
* @see https://api.highcharts.com/highstock/plotOptions.scatter
*
* @implspec scatter?: PlotScatterOptions;
*
*/
@JSProperty("scatter")
void setScatter(PlotScatterOptions value);
/**
* (Highcharts) A 3D scatter plot uses x, y and z coordinates to display
* values for three variables for a set of data.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
scatter3d
series are defined in
* plotOptions.scatter3d.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.scatter3d
*
* @implspec scatter3d?: PlotScatter3dOptions;
*
*/
@JSProperty("scatter3d")
@Nullable
PlotScatter3dOptions getScatter3d();
/**
* (Highcharts) A 3D scatter plot uses x, y and z coordinates to display
* values for three variables for a set of data.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
scatter3d
series are defined in
* plotOptions.scatter3d.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.scatter3d
*
* @implspec scatter3d?: PlotScatter3dOptions;
*
*/
@JSProperty("scatter3d")
void setScatter3d(PlotScatter3dOptions value);
/**
* (Highcharts, Highstock, Highmaps) General options for all series types.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
line
series are defined in plotOptions.line.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.series
* @see https://api.highcharts.com/highstock/plotOptions.series
* @see https://api.highcharts.com/highmaps/plotOptions.series
*
* @implspec series?: PlotSeriesOptions;
*
*/
@JSProperty("series")
@Nullable
PlotSeriesOptions getSeries();
/**
* (Highcharts, Highstock, Highmaps) General options for all series types.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
line
series are defined in plotOptions.line.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.series
* @see https://api.highcharts.com/highstock/plotOptions.series
* @see https://api.highcharts.com/highmaps/plotOptions.series
*
* @implspec series?: PlotSeriesOptions;
*
*/
@JSProperty("series")
void setSeries(PlotSeriesOptions value);
/**
* (Highstock) Simple moving average indicator (SMA). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
sma
series are defined in plotOptions.sma.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.sma
*
* @implspec sma?: PlotSmaOptions;
*
*/
@JSProperty("sma")
@Nullable
PlotSmaOptions getSma();
/**
* (Highstock) Simple moving average indicator (SMA). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
sma
series are defined in plotOptions.sma.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.sma
*
* @implspec sma?: PlotSmaOptions;
*
*/
@JSProperty("sma")
void setSma(PlotSmaOptions value);
/**
* (Highcharts) A solid gauge is a circular gauge where the value is
* indicated by a filled arc, and the color of the arc may variate with the
* value.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
solidgauge
series are defined in
* plotOptions.solidgauge.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.solidgauge
*
* @implspec solidgauge?: PlotSolidgaugeOptions;
*
*/
@JSProperty("solidgauge")
@Nullable
PlotSolidgaugeOptions getSolidgauge();
/**
* (Highcharts) A solid gauge is a circular gauge where the value is
* indicated by a filled arc, and the color of the arc may variate with the
* value.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
solidgauge
series are defined in
* plotOptions.solidgauge.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.solidgauge
*
* @implspec solidgauge?: PlotSolidgaugeOptions;
*
*/
@JSProperty("solidgauge")
void setSolidgauge(PlotSolidgaugeOptions value);
/**
* (Highcharts, Highstock) A spline series is a special type of line series,
* where the segments between the data points are smoothed.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
spline
series are defined in plotOptions.spline.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.spline
* @see https://api.highcharts.com/highstock/plotOptions.spline
*
* @implspec spline?: PlotSplineOptions;
*
*/
@JSProperty("spline")
@Nullable
PlotSplineOptions getSpline();
/**
* (Highcharts, Highstock) A spline series is a special type of line series,
* where the segments between the data points are smoothed.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
spline
series are defined in plotOptions.spline.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.spline
* @see https://api.highcharts.com/highstock/plotOptions.spline
*
* @implspec spline?: PlotSplineOptions;
*
*/
@JSProperty("spline")
void setSpline(PlotSplineOptions value);
/**
* (Highstock) Stochastic oscillator. This series requires the linkedTo
* option to be set and should be loaded after the
* stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
stochastic
series are defined in
* plotOptions.stochastic.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.stochastic
*
* @implspec stochastic?: PlotStochasticOptions;
*
*/
@JSProperty("stochastic")
@Nullable
PlotStochasticOptions getStochastic();
/**
* (Highstock) Stochastic oscillator. This series requires the linkedTo
* option to be set and should be loaded after the
* stock/indicators/indicators.js
file.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
stochastic
series are defined in
* plotOptions.stochastic.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.stochastic
*
* @implspec stochastic?: PlotStochasticOptions;
*
*/
@JSProperty("stochastic")
void setStochastic(PlotStochasticOptions value);
/**
* (Highcharts, Highstock) A streamgraph is a type of stacked area graph
* which is displaced around a central axis, resulting in a flowing, organic
* shape.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
streamgraph
series are defined in
* plotOptions.streamgraph.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.streamgraph
* @see https://api.highcharts.com/highstock/plotOptions.streamgraph
*
* @implspec streamgraph?: PlotStreamgraphOptions;
*
*/
@JSProperty("streamgraph")
@Nullable
PlotStreamgraphOptions getStreamgraph();
/**
* (Highcharts, Highstock) A streamgraph is a type of stacked area graph
* which is displaced around a central axis, resulting in a flowing, organic
* shape.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
streamgraph
series are defined in
* plotOptions.streamgraph.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.streamgraph
* @see https://api.highcharts.com/highstock/plotOptions.streamgraph
*
* @implspec streamgraph?: PlotStreamgraphOptions;
*
*/
@JSProperty("streamgraph")
void setStreamgraph(PlotStreamgraphOptions value);
/**
* (Highcharts) A Sunburst displays hierarchical data, where a level in the
* hierarchy is represented by a circle. The center represents the root node
* of the tree. The visualization bears a resemblance to both treemap and
* pie charts.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
sunburst
series are defined in plotOptions.sunburst.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.sunburst
*
* @implspec sunburst?: PlotSunburstOptions;
*
*/
@JSProperty("sunburst")
@Nullable
PlotSunburstOptions getSunburst();
/**
* (Highcharts) A Sunburst displays hierarchical data, where a level in the
* hierarchy is represented by a circle. The center represents the root node
* of the tree. The visualization bears a resemblance to both treemap and
* pie charts.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
sunburst
series are defined in plotOptions.sunburst.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.sunburst
*
* @implspec sunburst?: PlotSunburstOptions;
*
*/
@JSProperty("sunburst")
void setSunburst(PlotSunburstOptions value);
/**
* (Highcharts, Highmaps) A tilemap series is a type of heatmap where the
* tile shapes are configurable.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
tilemap
series are defined in plotOptions.tilemap.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.tilemap
* @see https://api.highcharts.com/highmaps/plotOptions.tilemap
*
* @implspec tilemap?: PlotTilemapOptions;
*
*/
@JSProperty("tilemap")
@Nullable
PlotTilemapOptions getTilemap();
/**
* (Highcharts, Highmaps) A tilemap series is a type of heatmap where the
* tile shapes are configurable.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
tilemap
series are defined in plotOptions.tilemap.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.tilemap
* @see https://api.highcharts.com/highmaps/plotOptions.tilemap
*
* @implspec tilemap?: PlotTilemapOptions;
*
*/
@JSProperty("tilemap")
void setTilemap(PlotTilemapOptions value);
/**
* (Highcharts) A treemap displays hierarchical data using nested
* rectangles. The data can be laid out in varying ways depending on
* options.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
treemap
series are defined in plotOptions.treemap.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap
*
* @implspec treemap?: PlotTreemapOptions;
*
*/
@JSProperty("treemap")
@Nullable
PlotTreemapOptions getTreemap();
/**
* (Highcharts) A treemap displays hierarchical data using nested
* rectangles. The data can be laid out in varying ways depending on
* options.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
treemap
series are defined in plotOptions.treemap.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap
*
* @implspec treemap?: PlotTreemapOptions;
*
*/
@JSProperty("treemap")
void setTreemap(PlotTreemapOptions value);
/**
* (Highcharts) A variable pie series is a two dimensional series type,
* where each point renders an Y and Z value. Each point is drawn as a pie
* slice where the size (arc) of the slice relates to the Y value and the
* radius of pie slice relates to the Z value. Requires
* highcharts-more.js
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
variablepie
series are defined in
* plotOptions.variablepie.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.variablepie
*
* @implspec variablepie?: PlotVariablepieOptions;
*
*/
@JSProperty("variablepie")
@Nullable
PlotVariablepieOptions getVariablepie();
/**
* (Highcharts) A variable pie series is a two dimensional series type,
* where each point renders an Y and Z value. Each point is drawn as a pie
* slice where the size (arc) of the slice relates to the Y value and the
* radius of pie slice relates to the Z value. Requires
* highcharts-more.js
.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
variablepie
series are defined in
* plotOptions.variablepie.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.variablepie
*
* @implspec variablepie?: PlotVariablepieOptions;
*
*/
@JSProperty("variablepie")
void setVariablepie(PlotVariablepieOptions value);
/**
* (Highcharts) A variwide chart (related to marimekko chart) is a column
* chart with a variable width expressing a third dimension.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
variwide
series are defined in plotOptions.variwide.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.variwide
*
* @implspec variwide?: PlotVariwideOptions;
*
*/
@JSProperty("variwide")
@Nullable
PlotVariwideOptions getVariwide();
/**
* (Highcharts) A variwide chart (related to marimekko chart) is a column
* chart with a variable width expressing a third dimension.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
variwide
series are defined in plotOptions.variwide.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.variwide
*
* @implspec variwide?: PlotVariwideOptions;
*
*/
@JSProperty("variwide")
void setVariwide(PlotVariwideOptions value);
/**
* (Highstock) Volume By Price indicator.
*
* This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
vbp
series are defined in plotOptions.vbp.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.vbp
*
* @implspec vbp?: PlotVbpOptions;
*
*/
@JSProperty("vbp")
@Nullable
PlotVbpOptions getVbp();
/**
* (Highstock) Volume By Price indicator.
*
* This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
vbp
series are defined in plotOptions.vbp.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.vbp
*
* @implspec vbp?: PlotVbpOptions;
*
*/
@JSProperty("vbp")
void setVbp(PlotVbpOptions value);
/**
* (Highcharts, Highstock) A vector plot is a type of cartesian chart where
* each point has an X and Y position, a length and a direction. Vectors are
* drawn as arrows.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
vector
series are defined in plotOptions.vector.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.vector
* @see https://api.highcharts.com/highstock/plotOptions.vector
*
* @implspec vector?: PlotVectorOptions;
*
*/
@JSProperty("vector")
@Nullable
PlotVectorOptions getVector();
/**
* (Highcharts, Highstock) A vector plot is a type of cartesian chart where
* each point has an X and Y position, a length and a direction. Vectors are
* drawn as arrows.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
vector
series are defined in plotOptions.vector.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.vector
* @see https://api.highcharts.com/highstock/plotOptions.vector
*
* @implspec vector?: PlotVectorOptions;
*
*/
@JSProperty("vector")
void setVector(PlotVectorOptions value);
/**
* (Highstock) Volume Weighted Average Price indicator.
*
* This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
vwap
series are defined in plotOptions.vwap.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.vwap
*
* @implspec vwap?: PlotVwapOptions;
*
*/
@JSProperty("vwap")
@Nullable
PlotVwapOptions getVwap();
/**
* (Highstock) Volume Weighted Average Price indicator.
*
* This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
vwap
series are defined in plotOptions.vwap.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.vwap
*
* @implspec vwap?: PlotVwapOptions;
*
*/
@JSProperty("vwap")
void setVwap(PlotVwapOptions value);
/**
* (Highcharts) A waterfall chart displays sequentially introduced positive
* or negative values in cumulative columns.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
waterfall
series are defined in
* plotOptions.waterfall.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.waterfall
*
* @implspec waterfall?: PlotWaterfallOptions;
*
*/
@JSProperty("waterfall")
@Nullable
PlotWaterfallOptions getWaterfall();
/**
* (Highcharts) A waterfall chart displays sequentially introduced positive
* or negative values in cumulative columns.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
waterfall
series are defined in
* plotOptions.waterfall.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.waterfall
*
* @implspec waterfall?: PlotWaterfallOptions;
*
*/
@JSProperty("waterfall")
void setWaterfall(PlotWaterfallOptions value);
/**
* (Highcharts, Highstock) Wind barbs are a convenient way to represent wind
* speed and direction in one graphical form. Wind direction is given by the
* stem direction, and wind speed by the number and shape of barbs.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
windbarb
series are defined in plotOptions.windbarb.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.windbarb
* @see https://api.highcharts.com/highstock/plotOptions.windbarb
*
* @implspec windbarb?: PlotWindbarbOptions;
*
*/
@JSProperty("windbarb")
@Nullable
PlotWindbarbOptions getWindbarb();
/**
* (Highcharts, Highstock) Wind barbs are a convenient way to represent wind
* speed and direction in one graphical form. Wind direction is given by the
* stem direction, and wind speed by the number and shape of barbs.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
windbarb
series are defined in plotOptions.windbarb.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.windbarb
* @see https://api.highcharts.com/highstock/plotOptions.windbarb
*
* @implspec windbarb?: PlotWindbarbOptions;
*
*/
@JSProperty("windbarb")
void setWindbarb(PlotWindbarbOptions value);
/**
* (Highstock) Weighted moving average indicator (WMA). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
wma
series are defined in plotOptions.wma.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.wma
*
* @implspec wma?: PlotWmaOptions;
*
*/
@JSProperty("wma")
@Nullable
PlotWmaOptions getWma();
/**
* (Highstock) Weighted moving average indicator (WMA). This series requires
* linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
wma
series are defined in plotOptions.wma.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.wma
*
* @implspec wma?: PlotWmaOptions;
*
*/
@JSProperty("wma")
void setWma(PlotWmaOptions value);
/**
* (Highcharts) A word cloud is a visualization of a set of words, where the
* size and placement of a word is determined by how it is weighted.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
wordcloud
series are defined in
* plotOptions.wordcloud.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.wordcloud
*
* @implspec wordcloud?: PlotWordcloudOptions;
*
*/
@JSProperty("wordcloud")
@Nullable
PlotWordcloudOptions getWordcloud();
/**
* (Highcharts) A word cloud is a visualization of a set of words, where the
* size and placement of a word is determined by how it is weighted.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
wordcloud
series are defined in
* plotOptions.wordcloud.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.wordcloud
*
* @implspec wordcloud?: PlotWordcloudOptions;
*
*/
@JSProperty("wordcloud")
void setWordcloud(PlotWordcloudOptions value);
/**
* (Highcharts, Highstock, Gantt) The X-range series displays ranges on the
* X axis, typically time intervals with a start and end date.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
xrange
series are defined in plotOptions.xrange.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.xrange
* @see https://api.highcharts.com/highstock/plotOptions.xrange
* @see https://api.highcharts.com/gantt/plotOptions.xrange
*
* @implspec xrange?: PlotXrangeOptions;
*
*/
@JSProperty("xrange")
@Nullable
PlotXrangeOptions getXrange();
/**
* (Highcharts, Highstock, Gantt) The X-range series displays ranges on the
* X axis, typically time intervals with a start and end date.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
xrange
series are defined in plotOptions.xrange.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highcharts/plotOptions.xrange
* @see https://api.highcharts.com/highstock/plotOptions.xrange
* @see https://api.highcharts.com/gantt/plotOptions.xrange
*
* @implspec xrange?: PlotXrangeOptions;
*
*/
@JSProperty("xrange")
void setXrange(PlotXrangeOptions value);
/**
* (Highstock) Zig Zag indicator.
*
* This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
zigzag
series are defined in plotOptions.zigzag.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.zigzag
*
* @implspec zigzag?: PlotZigzagOptions;
*
*/
@JSProperty("zigzag")
@Nullable
PlotZigzagOptions getZigzag();
/**
* (Highstock) Zig Zag indicator.
*
* This series requires linkedTo
option to be set.
*
* Configuration options for the series are given in three levels:
*
*
* -
* Options for all series in a chart are defined in the
* plotOptions.series object.
*
*
* -
* Options for all
zigzag
series are defined in plotOptions.zigzag.
*
*
* -
* Options for one single series are given in the series instance array.
*
*
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/plotOptions.zigzag
*
* @implspec zigzag?: PlotZigzagOptions;
*
*/
@JSProperty("zigzag")
void setZigzag(PlotZigzagOptions value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy