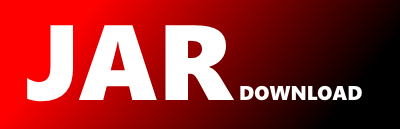
com.github.fluorumlabs.disconnect.highcharts.PlotTreemapLevelsOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import java.lang.String;
import javax.annotation.Nullable;
import js.extras.JsEnum;
import js.lang.Any;
import js.lang.Unknown;
import org.teavm.jso.JSProperty;
/**
* (Highcharts) Set options on specific levels. Takes precedence over series
* options, but not point options.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels
*
*/
public interface PlotTreemapLevelsOptions extends Any {
/**
* (Highcharts) Can set a borderColor
on all points which lies on the same
* level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.borderColor
*
* @implspec borderColor?: ColorString;
*
*/
@JSProperty("borderColor")
@Nullable
String getBorderColor();
/**
* (Highcharts) Can set a borderColor
on all points which lies on the same
* level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.borderColor
*
* @implspec borderColor?: ColorString;
*
*/
@JSProperty("borderColor")
void setBorderColor(String value);
/**
* (Highcharts) Set the dash style of the border of all the point which lies
* on the level. See (see online documentation for example) for possible
* options.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.borderDashStyle
*
* @implspec borderDashStyle?: string;
*
*/
@JSProperty("borderDashStyle")
@Nullable
String getBorderDashStyle();
/**
* (Highcharts) Set the dash style of the border of all the point which lies
* on the level. See (see online documentation for example) for possible
* options.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.borderDashStyle
*
* @implspec borderDashStyle?: string;
*
*/
@JSProperty("borderDashStyle")
void setBorderDashStyle(String value);
/**
* (Highcharts) Can set the borderWidth on all points which lies on the same
* level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.borderWidth
*
* @implspec borderWidth?: number;
*
*/
@JSProperty("borderWidth")
double getBorderWidth();
/**
* (Highcharts) Can set the borderWidth on all points which lies on the same
* level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.borderWidth
*
* @implspec borderWidth?: number;
*
*/
@JSProperty("borderWidth")
void setBorderWidth(double value);
/**
* (Highcharts) Can set a color on all points which lies on the same level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.color
*
* @implspec color?: (ColorString|GradientColorObject|object);
*
*/
@JSProperty("color")
@Nullable
Unknown getColor();
/**
* (Highcharts) Can set a color on all points which lies on the same level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.color
*
* @implspec color?: (ColorString|GradientColorObject|object);
*
*/
@JSProperty("color")
void setColor(GradientColorObject value);
/**
* (Highcharts) Can set a color on all points which lies on the same level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.color
*
* @implspec color?: (ColorString|GradientColorObject|object);
*
*/
@JSProperty("color")
void setColor(String value);
/**
* (Highcharts) Can set a color on all points which lies on the same level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.color
*
* @implspec color?: (ColorString|GradientColorObject|object);
*
*/
@JSProperty("color")
void setColor(Any value);
/**
* (Highcharts) A configuration object to define how the color of a child
* varies from the parent's color. The variation is distributed among the
* children of node. For example when setting brightness, the brightness
* change will range from the parent's original brightness on the first
* child, to the amount set in the to
setting on the last node. This
* allows a gradient-like color scheme that sets children out from each
* other while highlighting the grouping on treemaps and sectors on sunburst
* charts.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.colorVariation
*
* @implspec colorVariation?: PlotTreemapLevelsColorVariationOptions;
*
*/
@JSProperty("colorVariation")
@Nullable
PlotTreemapLevelsColorVariationOptions getColorVariation();
/**
* (Highcharts) A configuration object to define how the color of a child
* varies from the parent's color. The variation is distributed among the
* children of node. For example when setting brightness, the brightness
* change will range from the parent's original brightness on the first
* child, to the amount set in the to
setting on the last node. This
* allows a gradient-like color scheme that sets children out from each
* other while highlighting the grouping on treemaps and sectors on sunburst
* charts.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.colorVariation
*
* @implspec colorVariation?: PlotTreemapLevelsColorVariationOptions;
*
*/
@JSProperty("colorVariation")
void setColorVariation(PlotTreemapLevelsColorVariationOptions value);
/**
* (Highcharts) Can set the options of dataLabels on each point which lies
* on the level. plotOptions.treemap.dataLabels for possible values.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.dataLabels
*
* @implspec dataLabels?: object;
*
*/
@JSProperty("dataLabels")
@Nullable
Any getDataLabels();
/**
* (Highcharts) Can set the options of dataLabels on each point which lies
* on the level. plotOptions.treemap.dataLabels for possible values.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.dataLabels
*
* @implspec dataLabels?: object;
*
*/
@JSProperty("dataLabels")
void setDataLabels(Any value);
/**
* (Highcharts) Can set the layoutAlgorithm option on a specific level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.layoutAlgorithm
*
* @implspec layoutAlgorithm?: ("squarified"|"strip"|"stripes"|"sliceAndDice");
*
*/
@JSProperty("layoutAlgorithm")
@Nullable
LayoutAlgorithm getLayoutAlgorithm();
/**
* (Highcharts) Can set the layoutAlgorithm option on a specific level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.layoutAlgorithm
*
* @implspec layoutAlgorithm?: ("squarified"|"strip"|"stripes"|"sliceAndDice");
*
*/
@JSProperty("layoutAlgorithm")
void setLayoutAlgorithm(LayoutAlgorithm value);
/**
* (Highcharts) Can set the layoutStartingDirection option on a specific
* level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.layoutStartingDirection
*
* @implspec layoutStartingDirection?: ("horizontal"|"vertical");
*
*/
@JSProperty("layoutStartingDirection")
@Nullable
LayoutStartingDirection getLayoutStartingDirection();
/**
* (Highcharts) Can set the layoutStartingDirection option on a specific
* level.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.layoutStartingDirection
*
* @implspec layoutStartingDirection?: ("horizontal"|"vertical");
*
*/
@JSProperty("layoutStartingDirection")
void setLayoutStartingDirection(LayoutStartingDirection value);
/**
* (Highcharts) Decides which level takes effect from the options set in the
* levels object.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.level
*
* @implspec level?: number;
*
*/
@JSProperty("level")
double getLevel();
/**
* (Highcharts) Decides which level takes effect from the options set in the
* levels object.
*
* @see https://api.highcharts.com/highcharts/plotOptions.treemap.levels.level
*
* @implspec level?: number;
*
*/
@JSProperty("level")
void setLevel(double value);
/**
*/
abstract class LayoutAlgorithm extends JsEnum {
public static final LayoutAlgorithm SQUARIFIED = JsEnum.of("squarified");
public static final LayoutAlgorithm STRIP = JsEnum.of("strip");
public static final LayoutAlgorithm STRIPES = JsEnum.of("stripes");
public static final LayoutAlgorithm SLICEANDDICE = JsEnum.of("sliceAndDice");
}
/**
*/
abstract class LayoutStartingDirection extends JsEnum {
public static final LayoutStartingDirection HORIZONTAL = JsEnum.of("horizontal");
public static final LayoutStartingDirection VERTICAL = JsEnum.of("vertical");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy