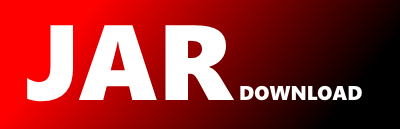
com.github.fluorumlabs.disconnect.highcharts.RangeSelectorOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import java.lang.String;
import javax.annotation.Nullable;
import js.extras.JsEnum;
import js.lang.Any;
import js.util.collections.Array;
import org.teavm.jso.JSProperty;
/**
* (Highstock) The range selector is a tool for selecting ranges to display
* within the chart. It provides buttons to select preconfigured ranges in the
* chart, like 1 day, 1 week, 1 month etc. It also provides input boxes where
* min and max dates can be manually input.
*
* @see https://api.highcharts.com/highstock/rangeSelector
*
*/
public interface RangeSelectorOptions extends Any {
/**
* (Highstock) Whether to enable all buttons from the start. By default
* buttons are only enabled if the corresponding time range exists on the X
* axis, but enabling all buttons allows for dynamically loading different
* time ranges.
*
* @see https://api.highcharts.com/highstock/rangeSelector.allButtonsEnabled
*
* @implspec allButtonsEnabled?: boolean;
*
*/
@JSProperty("allButtonsEnabled")
boolean getAllButtonsEnabled();
/**
* (Highstock) Whether to enable all buttons from the start. By default
* buttons are only enabled if the corresponding time range exists on the X
* axis, but enabling all buttons allows for dynamically loading different
* time ranges.
*
* @see https://api.highcharts.com/highstock/rangeSelector.allButtonsEnabled
*
* @implspec allButtonsEnabled?: boolean;
*
*/
@JSProperty("allButtonsEnabled")
void setAllButtonsEnabled(boolean value);
/**
* (Highstock) Positioning for the button row.
*
* @see https://api.highcharts.com/highstock/rangeSelector.buttonPosition
*
* @implspec buttonPosition?: RangeSelectorButtonPositionOptions;
*
*/
@JSProperty("buttonPosition")
@Nullable
RangeSelectorButtonPositionOptions getButtonPosition();
/**
* (Highstock) Positioning for the button row.
*
* @see https://api.highcharts.com/highstock/rangeSelector.buttonPosition
*
* @implspec buttonPosition?: RangeSelectorButtonPositionOptions;
*
*/
@JSProperty("buttonPosition")
void setButtonPosition(RangeSelectorButtonPositionOptions value);
/**
* (Highstock) An array of configuration objects for the buttons.
*
* Defaults to
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/rangeSelector.buttons
*
* @implspec buttons?: Array;
*
*/
@JSProperty("buttons")
@Nullable
Array getButtons();
/**
* (Highstock) An array of configuration objects for the buttons.
*
* Defaults to
*
* (see online documentation for example)
*
* @see https://api.highcharts.com/highstock/rangeSelector.buttons
*
* @implspec buttons?: Array;
*
*/
@JSProperty("buttons")
void setButtons(Array value);
/**
* (Highstock) The space in pixels between the buttons in the range
* selector.
*
* @see https://api.highcharts.com/highstock/rangeSelector.buttonSpacing
*
* @implspec buttonSpacing?: number;
*
*/
@JSProperty("buttonSpacing")
double getButtonSpacing();
/**
* (Highstock) The space in pixels between the buttons in the range
* selector.
*
* @see https://api.highcharts.com/highstock/rangeSelector.buttonSpacing
*
* @implspec buttonSpacing?: number;
*
*/
@JSProperty("buttonSpacing")
void setButtonSpacing(double value);
/**
* (Highstock) A collection of attributes for the buttons. The object takes
* SVG attributes like fill
, stroke
, stroke-width
, as well as style
,
* a collection of CSS properties for the text.
*
* The object can also be extended with states, so you can set
* presentational options for hover
, select
or disabled
button states.
*
* CSS styles for the text label.
*
* In styled mode, the buttons are styled by the
* .highcharts-range-selector-buttons .highcharts-button
rule with its
* different states.
*
* @see https://api.highcharts.com/highstock/rangeSelector.buttonTheme
*
* @implspec buttonTheme?: CSSObject;
*
*/
@JSProperty("buttonTheme")
@Nullable
CSSObject getButtonTheme();
/**
* (Highstock) A collection of attributes for the buttons. The object takes
* SVG attributes like fill
, stroke
, stroke-width
, as well as style
,
* a collection of CSS properties for the text.
*
* The object can also be extended with states, so you can set
* presentational options for hover
, select
or disabled
button states.
*
* CSS styles for the text label.
*
* In styled mode, the buttons are styled by the
* .highcharts-range-selector-buttons .highcharts-button
rule with its
* different states.
*
* @see https://api.highcharts.com/highstock/rangeSelector.buttonTheme
*
* @implspec buttonTheme?: CSSObject;
*
*/
@JSProperty("buttonTheme")
void setButtonTheme(CSSObject value);
/**
* (Highstock) Enable or disable the range selector.
*
* @see https://api.highcharts.com/highstock/rangeSelector.enabled
*
* @implspec enabled?: boolean;
*
*/
@JSProperty("enabled")
boolean getEnabled();
/**
* (Highstock) Enable or disable the range selector.
*
* @see https://api.highcharts.com/highstock/rangeSelector.enabled
*
* @implspec enabled?: boolean;
*
*/
@JSProperty("enabled")
void setEnabled(boolean value);
/**
* (Highstock) When the rangeselector is floating, the plot area does not
* reserve space for it. This opens for positioning anywhere on the chart.
*
* @see https://api.highcharts.com/highstock/rangeSelector.floating
*
* @implspec floating?: boolean;
*
*/
@JSProperty("floating")
boolean getFloating();
/**
* (Highstock) When the rangeselector is floating, the plot area does not
* reserve space for it. This opens for positioning anywhere on the chart.
*
* @see https://api.highcharts.com/highstock/rangeSelector.floating
*
* @implspec floating?: boolean;
*
*/
@JSProperty("floating")
void setFloating(boolean value);
/**
* (Highstock) Deprecated. The height of the range selector. Currently it is
* calculated dynamically.
*
* @see https://api.highcharts.com/highstock/rangeSelector.height
*
* @implspec height?: (number|undefined);
*
*/
@JSProperty("height")
double getHeight();
/**
* (Highstock) Deprecated. The height of the range selector. Currently it is
* calculated dynamically.
*
* @see https://api.highcharts.com/highstock/rangeSelector.height
*
* @implspec height?: (number|undefined);
*
*/
@JSProperty("height")
void setHeight(double value);
/**
* (Highstock) The border color of the date input boxes.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputBoxBorderColor
*
* @implspec inputBoxBorderColor?: ColorString;
*
*/
@JSProperty("inputBoxBorderColor")
@Nullable
String getInputBoxBorderColor();
/**
* (Highstock) The border color of the date input boxes.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputBoxBorderColor
*
* @implspec inputBoxBorderColor?: ColorString;
*
*/
@JSProperty("inputBoxBorderColor")
void setInputBoxBorderColor(String value);
/**
* (Highstock) The pixel height of the date input boxes.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputBoxHeight
*
* @implspec inputBoxHeight?: number;
*
*/
@JSProperty("inputBoxHeight")
double getInputBoxHeight();
/**
* (Highstock) The pixel height of the date input boxes.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputBoxHeight
*
* @implspec inputBoxHeight?: number;
*
*/
@JSProperty("inputBoxHeight")
void setInputBoxHeight(double value);
/**
* (Highstock) CSS for the container DIV holding the input boxes. Deprecated
* as of 1.2.5. Use inputPosition instead.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputBoxStyle
*
* @implspec inputBoxStyle?: CSSObject;
*
*/
@JSProperty("inputBoxStyle")
@Nullable
CSSObject getInputBoxStyle();
/**
* (Highstock) CSS for the container DIV holding the input boxes. Deprecated
* as of 1.2.5. Use inputPosition instead.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputBoxStyle
*
* @implspec inputBoxStyle?: CSSObject;
*
*/
@JSProperty("inputBoxStyle")
void setInputBoxStyle(CSSObject value);
/**
* (Highstock) The pixel width of the date input boxes.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputBoxWidth
*
* @implspec inputBoxWidth?: number;
*
*/
@JSProperty("inputBoxWidth")
double getInputBoxWidth();
/**
* (Highstock) The pixel width of the date input boxes.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputBoxWidth
*
* @implspec inputBoxWidth?: number;
*
*/
@JSProperty("inputBoxWidth")
void setInputBoxWidth(double value);
/**
* (Highstock) The date format in the input boxes when not selected for
* editing. Defaults to %b %e, %Y
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputDateFormat
*
* @implspec inputDateFormat?: string;
*
*/
@JSProperty("inputDateFormat")
@Nullable
String getInputDateFormat();
/**
* (Highstock) The date format in the input boxes when not selected for
* editing. Defaults to %b %e, %Y
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputDateFormat
*
* @implspec inputDateFormat?: string;
*
*/
@JSProperty("inputDateFormat")
void setInputDateFormat(String value);
/**
* (Highstock) A custom callback function to parse values entered in the
* input boxes and return a valid JavaScript time as milliseconds since
* 1970.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputDateParser
*
* @implspec inputDateParser?: RangeSelectorParseCallbackFunction;
*
*/
@JSProperty("inputDateParser")
@Nullable
RangeSelectorParseCallbackFunction getInputDateParser();
/**
* (Highstock) A custom callback function to parse values entered in the
* input boxes and return a valid JavaScript time as milliseconds since
* 1970.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputDateParser
*
* @implspec inputDateParser?: RangeSelectorParseCallbackFunction;
*
*/
@JSProperty("inputDateParser")
void setInputDateParser(RangeSelectorParseCallbackFunction value);
/**
* (Highstock) The date format in the input boxes when they are selected for
* editing. This must be a format that is recognized by JavaScript
* Date.parse.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputEditDateFormat
*
* @implspec inputEditDateFormat?: string;
*
*/
@JSProperty("inputEditDateFormat")
@Nullable
String getInputEditDateFormat();
/**
* (Highstock) The date format in the input boxes when they are selected for
* editing. This must be a format that is recognized by JavaScript
* Date.parse.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputEditDateFormat
*
* @implspec inputEditDateFormat?: string;
*
*/
@JSProperty("inputEditDateFormat")
void setInputEditDateFormat(String value);
/**
* (Highstock) Enable or disable the date input boxes. Defaults to enabled
* when there is enough space, disabled if not (typically mobile).
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputEnabled
*
* @implspec inputEnabled?: boolean;
*
*/
@JSProperty("inputEnabled")
boolean getInputEnabled();
/**
* (Highstock) Enable or disable the date input boxes. Defaults to enabled
* when there is enough space, disabled if not (typically mobile).
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputEnabled
*
* @implspec inputEnabled?: boolean;
*
*/
@JSProperty("inputEnabled")
void setInputEnabled(boolean value);
/**
* (Highstock) Positioning for the input boxes. Allowed properties are
* align
, x
and y
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputPosition
*
* @implspec inputPosition?: RangeSelectorInputPositionOptions;
*
*/
@JSProperty("inputPosition")
@Nullable
RangeSelectorInputPositionOptions getInputPosition();
/**
* (Highstock) Positioning for the input boxes. Allowed properties are
* align
, x
and y
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputPosition
*
* @implspec inputPosition?: RangeSelectorInputPositionOptions;
*
*/
@JSProperty("inputPosition")
void setInputPosition(RangeSelectorInputPositionOptions value);
/**
* (Highstock) CSS for the HTML inputs in the range selector.
*
* In styled mode, the inputs are styled by the .highcharts-range-input text
rule in SVG mode, and input.highcharts-range-selector
when
* active.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputStyle
*
* @implspec inputStyle?: CSSObject;
*
*/
@JSProperty("inputStyle")
@Nullable
CSSObject getInputStyle();
/**
* (Highstock) CSS for the HTML inputs in the range selector.
*
* In styled mode, the inputs are styled by the .highcharts-range-input text
rule in SVG mode, and input.highcharts-range-selector
when
* active.
*
* @see https://api.highcharts.com/highstock/rangeSelector.inputStyle
*
* @implspec inputStyle?: CSSObject;
*
*/
@JSProperty("inputStyle")
void setInputStyle(CSSObject value);
/**
* (Highstock) CSS styles for the labels - the Zoom, From and To texts.
*
* In styled mode, the labels are styled by the .highcharts-range-label
* class.
*
* @see https://api.highcharts.com/highstock/rangeSelector.labelStyle
*
* @implspec labelStyle?: CSSObject;
*
*/
@JSProperty("labelStyle")
@Nullable
CSSObject getLabelStyle();
/**
* (Highstock) CSS styles for the labels - the Zoom, From and To texts.
*
* In styled mode, the labels are styled by the .highcharts-range-label
* class.
*
* @see https://api.highcharts.com/highstock/rangeSelector.labelStyle
*
* @implspec labelStyle?: CSSObject;
*
*/
@JSProperty("labelStyle")
void setLabelStyle(CSSObject value);
/**
* (Highstock) The index of the button to appear pre-selected.
*
* @see https://api.highcharts.com/highstock/rangeSelector.selected
*
* @implspec selected?: number;
*
*/
@JSProperty("selected")
double getSelected();
/**
* (Highstock) The index of the button to appear pre-selected.
*
* @see https://api.highcharts.com/highstock/rangeSelector.selected
*
* @implspec selected?: number;
*
*/
@JSProperty("selected")
void setSelected(double value);
/**
* (Highstock) The vertical alignment of the rangeselector box. Allowed
* properties are top
, middle
, bottom
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.verticalAlign
*
* @implspec verticalAlign?: ("bottom"|"middle"|"top");
*
*/
@JSProperty("verticalAlign")
@Nullable
VerticalAlign getVerticalAlign();
/**
* (Highstock) The vertical alignment of the rangeselector box. Allowed
* properties are top
, middle
, bottom
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.verticalAlign
*
* @implspec verticalAlign?: ("bottom"|"middle"|"top");
*
*/
@JSProperty("verticalAlign")
void setVerticalAlign(VerticalAlign value);
/**
* (Highstock) The x offset of the range selector relative to its horizontal
* alignment within chart.spacingLeft
and chart.spacingRight
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.x
*
* @implspec x?: number;
*
*/
@JSProperty("x")
double getX();
/**
* (Highstock) The x offset of the range selector relative to its horizontal
* alignment within chart.spacingLeft
and chart.spacingRight
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.x
*
* @implspec x?: number;
*
*/
@JSProperty("x")
void setX(double value);
/**
* (Highstock) The y offset of the range selector relative to its horizontal
* alignment within chart.spacingLeft
and chart.spacingRight
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.y
*
* @implspec y?: number;
*
*/
@JSProperty("y")
double getY();
/**
* (Highstock) The y offset of the range selector relative to its horizontal
* alignment within chart.spacingLeft
and chart.spacingRight
.
*
* @see https://api.highcharts.com/highstock/rangeSelector.y
*
* @implspec y?: number;
*
*/
@JSProperty("y")
void setY(double value);
/**
*/
abstract class VerticalAlign extends JsEnum {
public static final VerticalAlign BOTTOM = JsEnum.of("bottom");
public static final VerticalAlign MIDDLE = JsEnum.of("middle");
public static final VerticalAlign TOP = JsEnum.of("top");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy