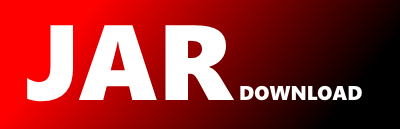
com.github.fluorumlabs.disconnect.highcharts.SeriesGanttDataOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import java.lang.String;
import javax.annotation.Nullable;
import js.lang.Any;
import js.lang.Unknown;
import js.util.collections.Array;
import org.teavm.jso.JSProperty;
/**
* (Gantt) Data for a Gantt series.
*
* @see https://api.highcharts.com/gantt/series.gantt.data
*
*/
public interface SeriesGanttDataOptions extends Any {
/**
* (Gantt) Whether the grid node belonging to this point should start as
* collapsed. Used in axes of type treegrid.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.collapsed
*
* @implspec collapsed?: boolean;
*
*/
@JSProperty("collapsed")
boolean getCollapsed();
/**
* (Gantt) Whether the grid node belonging to this point should start as
* collapsed. Used in axes of type treegrid.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.collapsed
*
* @implspec collapsed?: boolean;
*
*/
@JSProperty("collapsed")
void setCollapsed(boolean value);
/**
* (Gantt) Progress indicator, how much of the task completed. If it is a
* number, the fill
will be applied automatically.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.completed
*
* @implspec completed?: (number|SeriesGanttDataCompletedOptions);
*
*/
@JSProperty("completed")
@Nullable
Unknown getCompleted();
/**
* (Gantt) Progress indicator, how much of the task completed. If it is a
* number, the fill
will be applied automatically.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.completed
*
* @implspec completed?: (number|SeriesGanttDataCompletedOptions);
*
*/
@JSProperty("completed")
void setCompleted(double value);
/**
* (Gantt) Progress indicator, how much of the task completed. If it is a
* number, the fill
will be applied automatically.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.completed
*
* @implspec completed?: (number|SeriesGanttDataCompletedOptions);
*
*/
@JSProperty("completed")
void setCompleted(SeriesGanttDataCompletedOptions value);
/**
* (Gantt) The ID of the point (task) that this point depends on in Gantt
* charts. Aliases connect. Can also be an object, specifying further
* connecting options between the points. Multiple connections can be
* specified by providing an array.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.dependency
*
* @implspec dependency?: (string|SeriesGanttDataDependencyOptions|Array<(string|SeriesGanttDataDependencyOptions)>);
*
*/
@JSProperty("dependency")
@Nullable
Unknown getDependency();
/**
* (Gantt) The ID of the point (task) that this point depends on in Gantt
* charts. Aliases connect. Can also be an object, specifying further
* connecting options between the points. Multiple connections can be
* specified by providing an array.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.dependency
*
* @implspec dependency?: (string|SeriesGanttDataDependencyOptions|Array<(string|SeriesGanttDataDependencyOptions)>);
*
*/
@JSProperty("dependency")
void setDependency(SeriesGanttDataDependencyOptions value);
/**
* (Gantt) The ID of the point (task) that this point depends on in Gantt
* charts. Aliases connect. Can also be an object, specifying further
* connecting options between the points. Multiple connections can be
* specified by providing an array.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.dependency
*
* @implspec dependency?: (string|SeriesGanttDataDependencyOptions|Array<(string|SeriesGanttDataDependencyOptions)>);
*
*/
@JSProperty("dependency")
void setDependency(String value);
/**
* (Gantt) The ID of the point (task) that this point depends on in Gantt
* charts. Aliases connect. Can also be an object, specifying further
* connecting options between the points. Multiple connections can be
* specified by providing an array.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.dependency
*
* @implspec dependency?: (string|SeriesGanttDataDependencyOptions|Array<(string|SeriesGanttDataDependencyOptions)>);
*
*/
@JSProperty("dependency")
void setDependency(Array value);
/**
* (Gantt) A description of the point to add to the screen reader
* information about the point. Requires the Accessibility module.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.description
*
* @implspec description?: string;
*
*/
@JSProperty("description")
@Nullable
String getDescription();
/**
* (Gantt) A description of the point to add to the screen reader
* information about the point. Requires the Accessibility module.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.description
*
* @implspec description?: string;
*
*/
@JSProperty("description")
void setDescription(String value);
/**
* (Highcharts, Highstock, Highmaps) Point specific options for the
* draggable-points module. Overrides options on series.dragDrop
.
*
* Requires the draggable-points
module.
*
* @see https://api.highcharts.com/highcharts/series.gantt.data.dragDrop
* @see https://api.highcharts.com/highstock/series.gantt.data.dragDrop
* @see https://api.highcharts.com/highmaps/series.gantt.data.dragDrop
*
* @implspec dragDrop?: SeriesGanttDataDragDropOptions;
*
*/
@JSProperty("dragDrop")
@Nullable
SeriesGanttDataDragDropOptions getDragDrop();
/**
* (Highcharts, Highstock, Highmaps) Point specific options for the
* draggable-points module. Overrides options on series.dragDrop
.
*
* Requires the draggable-points
module.
*
* @see https://api.highcharts.com/highcharts/series.gantt.data.dragDrop
* @see https://api.highcharts.com/highstock/series.gantt.data.dragDrop
* @see https://api.highcharts.com/highmaps/series.gantt.data.dragDrop
*
* @implspec dragDrop?: SeriesGanttDataDragDropOptions;
*
*/
@JSProperty("dragDrop")
void setDragDrop(SeriesGanttDataDragDropOptions value);
/**
* (Highcharts) The id
of a series in the drilldown.series array to use
* for a drilldown for this point.
*
* @see https://api.highcharts.com/highcharts/series.gantt.data.drilldown
*
* @implspec drilldown?: string;
*
*/
@JSProperty("drilldown")
@Nullable
String getDrilldown();
/**
* (Highcharts) The id
of a series in the drilldown.series array to use
* for a drilldown for this point.
*
* @see https://api.highcharts.com/highcharts/series.gantt.data.drilldown
*
* @implspec drilldown?: string;
*
*/
@JSProperty("drilldown")
void setDrilldown(String value);
/**
* (Gantt) The end time of a task.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.end
*
* @implspec end?: number;
*
*/
@JSProperty("end")
double getEnd();
/**
* (Gantt) The end time of a task.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.end
*
* @implspec end?: number;
*
*/
@JSProperty("end")
void setEnd(double value);
/**
* (Gantt) The rank for this point's data label in case of collision. If two
* data labels are about to overlap, only the one with the highest
* labelrank
will be drawn.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.labelrank
*
* @implspec labelrank?: number;
*
*/
@JSProperty("labelrank")
double getLabelrank();
/**
* (Gantt) The rank for this point's data label in case of collision. If two
* data labels are about to overlap, only the one with the highest
* labelrank
will be drawn.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.labelrank
*
* @implspec labelrank?: number;
*
*/
@JSProperty("labelrank")
void setLabelrank(double value);
/**
* (Highcharts, Highstock) Options for the point markers of line-like
* series. Properties like fillColor
, lineColor
and lineWidth
define
* the visual appearance of the markers. Other series types, like column
* series, don't have markers, but have visual options on the series level
* instead.
*
* In styled mode, the markers can be styled with the .highcharts-point
,
* .highcharts-point-hover
and .highcharts-point-select
class names.
*
* @see https://api.highcharts.com/highcharts/series.gantt.data.marker
* @see https://api.highcharts.com/highstock/series.gantt.data.marker
*
* @implspec marker?: SeriesGanttDataMarkerOptions;
*
*/
@JSProperty("marker")
@Nullable
SeriesGanttDataMarkerOptions getMarker();
/**
* (Highcharts, Highstock) Options for the point markers of line-like
* series. Properties like fillColor
, lineColor
and lineWidth
define
* the visual appearance of the markers. Other series types, like column
* series, don't have markers, but have visual options on the series level
* instead.
*
* In styled mode, the markers can be styled with the .highcharts-point
,
* .highcharts-point-hover
and .highcharts-point-select
class names.
*
* @see https://api.highcharts.com/highcharts/series.gantt.data.marker
* @see https://api.highcharts.com/highstock/series.gantt.data.marker
*
* @implspec marker?: SeriesGanttDataMarkerOptions;
*
*/
@JSProperty("marker")
void setMarker(SeriesGanttDataMarkerOptions value);
/**
* (Gantt) Whether this point is a milestone. If so, only the start
option
* is handled, while end
is ignored.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.milestone
*
* @implspec milestone?: boolean;
*
*/
@JSProperty("milestone")
boolean getMilestone();
/**
* (Gantt) Whether this point is a milestone. If so, only the start
option
* is handled, while end
is ignored.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.milestone
*
* @implspec milestone?: boolean;
*
*/
@JSProperty("milestone")
void setMilestone(boolean value);
/**
* (Gantt) The name of a task. If a treegrid
y-axis is used (default in
* Gantt charts), this will be picked up automatically, and used to
* calculate the y-value.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.name
*
* @implspec name?: string;
*
*/
@JSProperty("name")
@Nullable
String getName();
/**
* (Gantt) The name of a task. If a treegrid
y-axis is used (default in
* Gantt charts), this will be picked up automatically, and used to
* calculate the y-value.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.name
*
* @implspec name?: string;
*
*/
@JSProperty("name")
void setName(String value);
/**
* (Gantt) The ID of the parent point (task) of this point in Gantt charts.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.parent
*
* @implspec parent?: string;
*
*/
@JSProperty("parent")
@Nullable
String getParent();
/**
* (Gantt) The ID of the parent point (task) of this point in Gantt charts.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.parent
*
* @implspec parent?: string;
*
*/
@JSProperty("parent")
void setParent(String value);
/**
* (Gantt) The start time of a task.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.start
*
* @implspec start?: number;
*
*/
@JSProperty("start")
double getStart();
/**
* (Gantt) The start time of a task.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.start
*
* @implspec start?: number;
*
*/
@JSProperty("start")
void setStart(double value);
/**
* (Gantt) The Y value of a task.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.y
*
* @implspec y?: number;
*
*/
@JSProperty("y")
double getY();
/**
* (Gantt) The Y value of a task.
*
* @see https://api.highcharts.com/gantt/series.gantt.data.y
*
* @implspec y?: number;
*
*/
@JSProperty("y")
void setY(double value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy