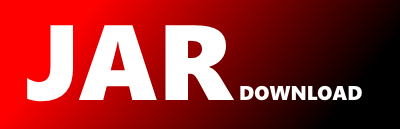
com.github.fluorumlabs.disconnect.highcharts.SeriesOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of disconnect-highcharts Show documentation
Show all versions of disconnect-highcharts Show documentation
Highcharts API bindings for Disconnect Zero
package com.github.fluorumlabs.disconnect.highcharts;
import js.extras.JsEnum;
import js.lang.Any;
import js.lang.Unknown;
import js.util.collections.Array;
import org.teavm.jso.JSProperty;
import javax.annotation.Nullable;
/**
* (Highcharts, Highstock, Highmaps) Series options for specific data and the
* data itself. In TypeScript you have to cast the series options to specific
* series types, to get all possible options for a series.
*
* @see https://api.highcharts.com/highcharts/series
* @see https://api.highcharts.com/highstock/series
* @see https://api.highcharts.com/highmaps/series
*
*/
public interface SeriesOptions extends Any {
/**
* (Highcharts, Highstock, Highmaps) An id for the series. This can be used
* after render time to get a pointer to the series object through
* chart.get()
.
*
* @see https://api.highcharts.com/highcharts/series.id
* @see https://api.highcharts.com/highstock/series.id
* @see https://api.highcharts.com/highmaps/series.id
*
* @implspec id?: string;
*
*/
@JSProperty("id")
@Nullable
String getId();
/**
* (Highcharts, Highstock, Highmaps) An id for the series. This can be used
* after render time to get a pointer to the series object through
* chart.get()
.
*
* @see https://api.highcharts.com/highcharts/series.id
* @see https://api.highcharts.com/highstock/series.id
* @see https://api.highcharts.com/highmaps/series.id
*
* @implspec id?: string;
*
*/
@JSProperty("id")
void setId(String value);
/**
* (Highcharts, Highstock, Highmaps) The index of the series in the chart,
* affecting the internal index in the chart.series
array, the visible Z
* index as well as the order in the legend.
*
* @see https://api.highcharts.com/highcharts/series.index
* @see https://api.highcharts.com/highstock/series.index
* @see https://api.highcharts.com/highmaps/series.index
*
* @implspec index?: number;
*
*/
@JSProperty("index")
double getIndex();
/**
* (Highcharts, Highstock, Highmaps) The index of the series in the chart,
* affecting the internal index in the chart.series
array, the visible Z
* index as well as the order in the legend.
*
* @see https://api.highcharts.com/highcharts/series.index
* @see https://api.highcharts.com/highstock/series.index
* @see https://api.highcharts.com/highmaps/series.index
*
* @implspec index?: number;
*
*/
@JSProperty("index")
void setIndex(double value);
/**
* (Highcharts, Highstock, Highmaps) The sequential index of the series in
* the legend.
*
* @see https://api.highcharts.com/highcharts/series.legendIndex
* @see https://api.highcharts.com/highstock/series.legendIndex
* @see https://api.highcharts.com/highmaps/series.legendIndex
*
* @implspec legendIndex?: number;
*
*/
@JSProperty("legendIndex")
double getLegendIndex();
/**
* (Highcharts, Highstock, Highmaps) The sequential index of the series in
* the legend.
*
* @see https://api.highcharts.com/highcharts/series.legendIndex
* @see https://api.highcharts.com/highstock/series.legendIndex
* @see https://api.highcharts.com/highmaps/series.legendIndex
*
* @implspec legendIndex?: number;
*
*/
@JSProperty("legendIndex")
void setLegendIndex(double value);
/**
* (Highmaps) A map data object containing a path
definition and
* optionally additional properties to join in the data as per the joinBy
* option.
*
* @see https://api.highcharts.com/highmaps/series.mapData
*
* @implspec mapData?: (MapDataObject|Array);
*
*/
@JSProperty("mapData")
@Nullable
Unknown getMapData();
/**
* (Highmaps) A map data object containing a path
definition and
* optionally additional properties to join in the data as per the joinBy
* option.
*
* @see https://api.highcharts.com/highmaps/series.mapData
*
* @implspec mapData?: (MapDataObject|Array);
*
*/
@JSProperty("mapData")
void setMapData(MapDataObject value);
/**
* (Highmaps) A map data object containing a path
definition and
* optionally additional properties to join in the data as per the joinBy
* option.
*
* @see https://api.highcharts.com/highmaps/series.mapData
*
* @implspec mapData?: (MapDataObject|Array);
*
*/
@JSProperty("mapData")
void setMapData(Array value);
/**
* (Highcharts, Highstock, Highmaps) The name of the series as shown in the
* legend, tooltip etc.
*
* @see https://api.highcharts.com/highcharts/series.name
* @see https://api.highcharts.com/highstock/series.name
* @see https://api.highcharts.com/highmaps/series.name
*
* @implspec name?: string;
*
*/
@JSProperty("name")
@Nullable
String getName();
/**
* (Highcharts, Highstock, Highmaps) The name of the series as shown in the
* legend, tooltip etc.
*
* @see https://api.highcharts.com/highcharts/series.name
* @see https://api.highcharts.com/highstock/series.name
* @see https://api.highcharts.com/highmaps/series.name
*
* @implspec name?: string;
*
*/
@JSProperty("name")
void setName(String value);
/**
* (Highcharts, Highstock) This option allows grouping series in a stacked
* chart. The stack option can be a string or anything else, as long as the
* grouped series' stack options match each other after conversion into a
* string.
*
* @see https://api.highcharts.com/highcharts/series.stack
* @see https://api.highcharts.com/highstock/series.stack
*
* @implspec stack?: (object|string);
*
*/
@JSProperty("stack")
@Nullable
Unknown getStack();
/**
* (Highcharts, Highstock) This option allows grouping series in a stacked
* chart. The stack option can be a string or anything else, as long as the
* grouped series' stack options match each other after conversion into a
* string.
*
* @see https://api.highcharts.com/highcharts/series.stack
* @see https://api.highcharts.com/highstock/series.stack
*
* @implspec stack?: (object|string);
*
*/
@JSProperty("stack")
void setStack(String value);
/**
* (Highcharts, Highstock) This option allows grouping series in a stacked
* chart. The stack option can be a string or anything else, as long as the
* grouped series' stack options match each other after conversion into a
* string.
*
* @see https://api.highcharts.com/highcharts/series.stack
* @see https://api.highcharts.com/highstock/series.stack
*
* @implspec stack?: (object|string);
*
*/
@JSProperty("stack")
void setStack(Any value);
/**
* (Highcharts, Highstock, Highmaps) The type of series, for example line
* or column
. By default, the series type is inherited from chart.type, so
* unless the chart is a combination of series types, there is no need to
* set it on the series level.
*
* @see https://api.highcharts.com/highcharts/series.type
* @see https://api.highcharts.com/highstock/series.type
* @see https://api.highcharts.com/highmaps/series.type
*
* @implspec type: string;
*
*/
@JSProperty("type")
JsEnum getType();
/**
* (Highcharts, Highstock, Highmaps) The type of series, for example line
* or column
. By default, the series type is inherited from chart.type, so
* unless the chart is a combination of series types, there is no need to
* set it on the series level.
*
* @see https://api.highcharts.com/highcharts/series.type
* @see https://api.highcharts.com/highstock/series.type
* @see https://api.highcharts.com/highmaps/series.type
*
* @implspec type: string;
*
*/
@JSProperty("type")
void setType(JsEnum value);
/**
* (Highcharts, Highstock) When using dual or multiple x axes, this number
* defines which xAxis the particular series is connected to. It refers to
* either the axis id or the index of the axis in the xAxis array, with 0
* being the first.
*
* @see https://api.highcharts.com/highcharts/series.xAxis
* @see https://api.highcharts.com/highstock/series.xAxis
*
* @implspec xAxis?: (number|string);
*
*/
@JSProperty("xAxis")
@Nullable
Unknown getXAxis();
/**
* (Highcharts, Highstock) When using dual or multiple x axes, this number
* defines which xAxis the particular series is connected to. It refers to
* either the axis id or the index of the axis in the xAxis array, with 0
* being the first.
*
* @see https://api.highcharts.com/highcharts/series.xAxis
* @see https://api.highcharts.com/highstock/series.xAxis
*
* @implspec xAxis?: (number|string);
*
*/
@JSProperty("xAxis")
void setXAxis(double value);
/**
* (Highcharts, Highstock) When using dual or multiple x axes, this number
* defines which xAxis the particular series is connected to. It refers to
* either the axis id or the index of the axis in the xAxis array, with 0
* being the first.
*
* @see https://api.highcharts.com/highcharts/series.xAxis
* @see https://api.highcharts.com/highstock/series.xAxis
*
* @implspec xAxis?: (number|string);
*
*/
@JSProperty("xAxis")
void setXAxis(String value);
/**
* (Highcharts, Highstock) When using dual or multiple y axes, this number
* defines which yAxis the particular series is connected to. It refers to
* either the axis id or the index of the axis in the yAxis array, with 0
* being the first.
*
* @see https://api.highcharts.com/highcharts/series.yAxis
* @see https://api.highcharts.com/highstock/series.yAxis
*
* @implspec yAxis?: (number|string);
*
*/
@JSProperty("yAxis")
@Nullable
Unknown getYAxis();
/**
* (Highcharts, Highstock) When using dual or multiple y axes, this number
* defines which yAxis the particular series is connected to. It refers to
* either the axis id or the index of the axis in the yAxis array, with 0
* being the first.
*
* @see https://api.highcharts.com/highcharts/series.yAxis
* @see https://api.highcharts.com/highstock/series.yAxis
*
* @implspec yAxis?: (number|string);
*
*/
@JSProperty("yAxis")
void setYAxis(double value);
/**
* (Highcharts, Highstock) When using dual or multiple y axes, this number
* defines which yAxis the particular series is connected to. It refers to
* either the axis id or the index of the axis in the yAxis array, with 0
* being the first.
*
* @see https://api.highcharts.com/highcharts/series.yAxis
* @see https://api.highcharts.com/highstock/series.yAxis
*
* @implspec yAxis?: (number|string);
*
*/
@JSProperty("yAxis")
void setYAxis(String value);
/**
* (Highcharts, Highstock) Define the visual z index of the series.
*
* @see https://api.highcharts.com/highcharts/series.zIndex
* @see https://api.highcharts.com/highstock/series.zIndex
*
* @implspec zIndex?: number;
*
*/
@JSProperty("zIndex")
double getZIndex();
/**
* (Highcharts, Highstock) Define the visual z index of the series.
*
* @see https://api.highcharts.com/highcharts/series.zIndex
* @see https://api.highcharts.com/highstock/series.zIndex
*
* @implspec zIndex?: number;
*
*/
@JSProperty("zIndex")
void setZIndex(double value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy